vb_ufunc¶
numpy.abs(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.abs(a) for t, a in squares_.iteritems() if t in types]
Performance graph
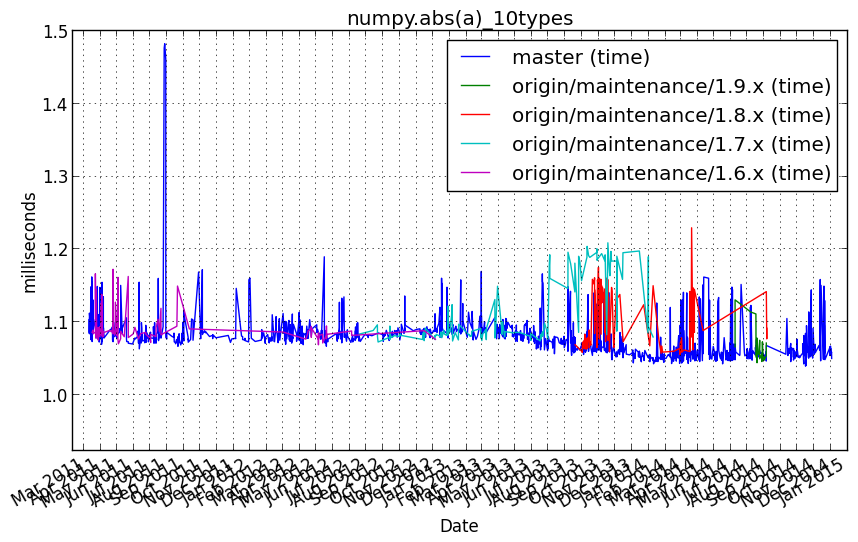
numpy.absolute(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.absolute(a) for t, a in squares_.iteritems() if t in types]
Performance graph
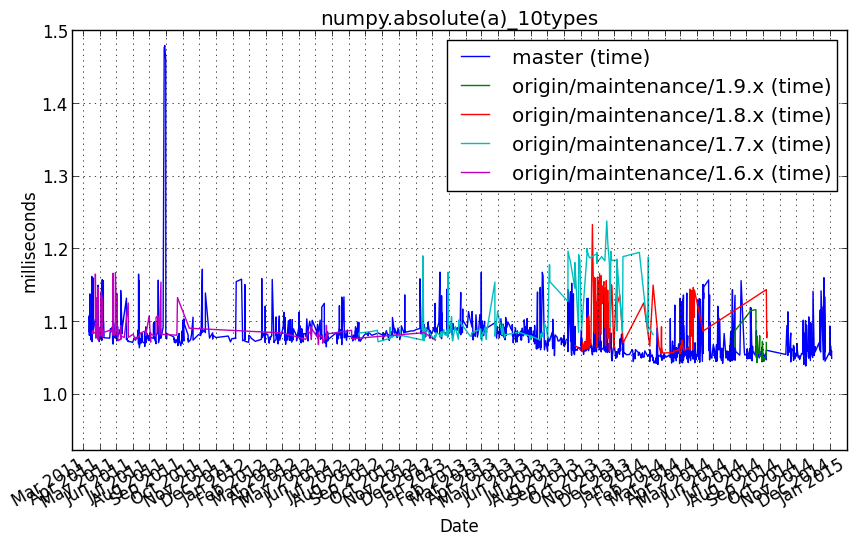
numpy.add(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.add(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
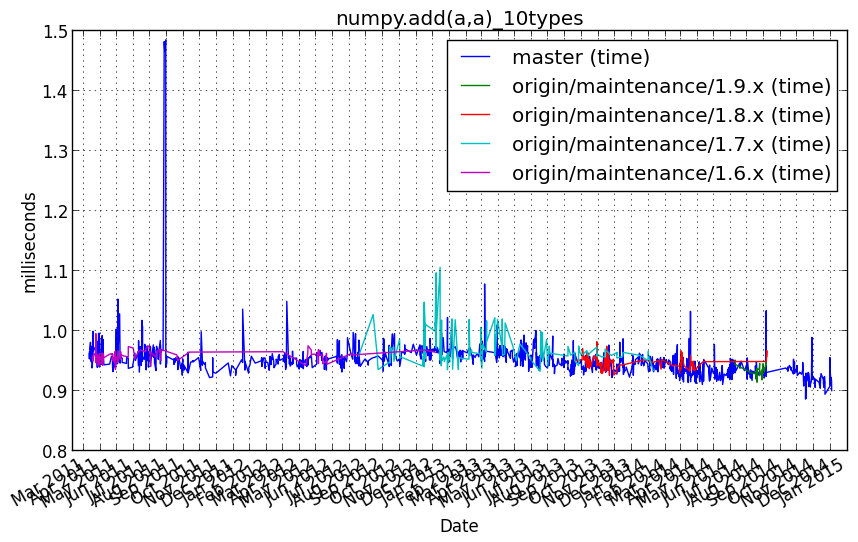
numpy.add_scalar¶
Benchmark setup
from numpy_vb_common import *
x = numpy.asarray(1.0)
Benchmark statement
x+x
Performance graph
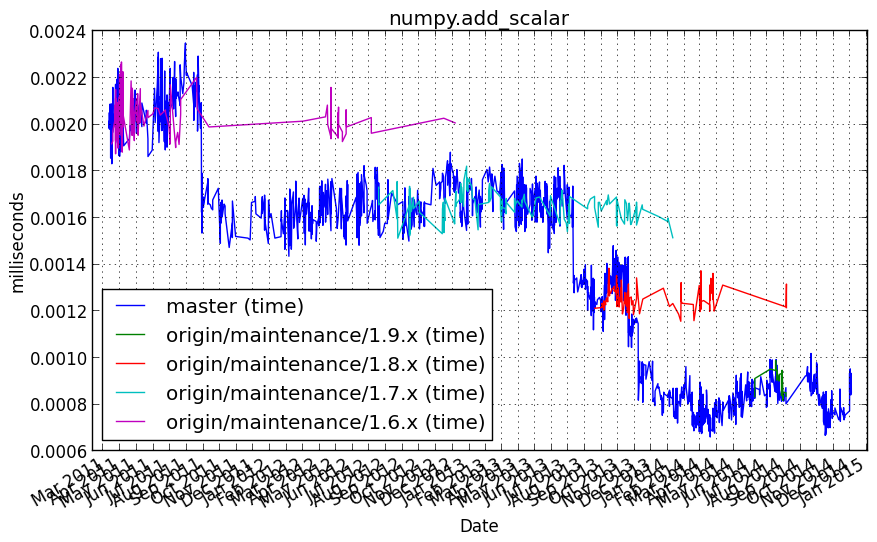
numpy.add_scalar2_numpy.float32¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float32)
Benchmark statement
numpy.add(d, 1)
Performance graph
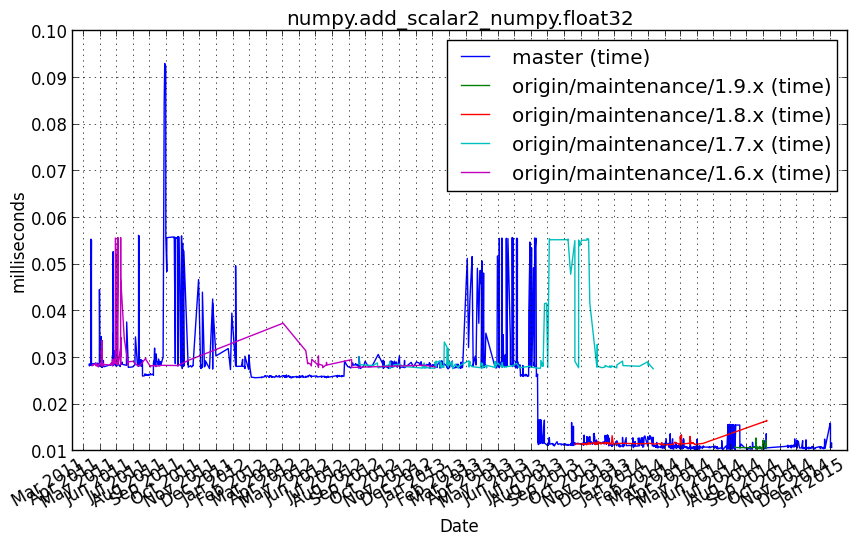
numpy.add_scalar2_numpy.float64¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float64)
Benchmark statement
numpy.add(d, 1)
Performance graph
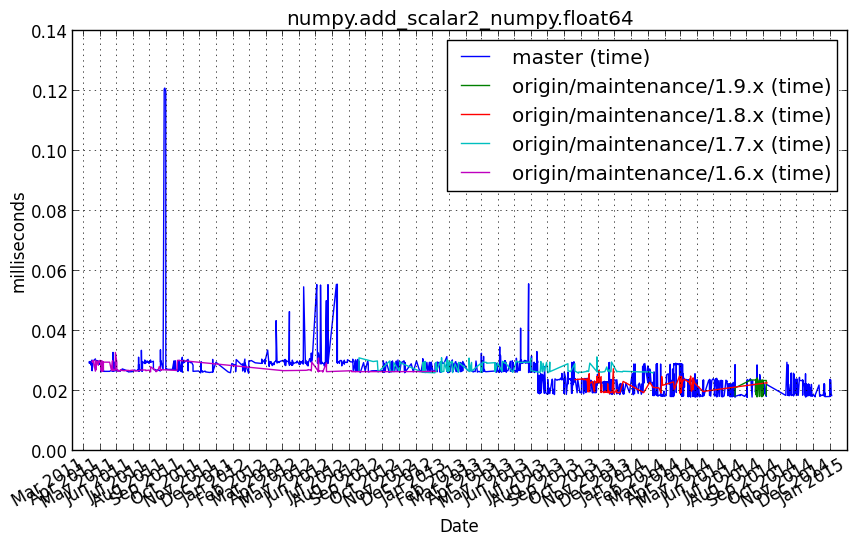
numpy.add_scalar_conv¶
Benchmark setup
from numpy_vb_common import *
x = numpy.asarray(1.0)
Benchmark statement
x+1.
Performance graph
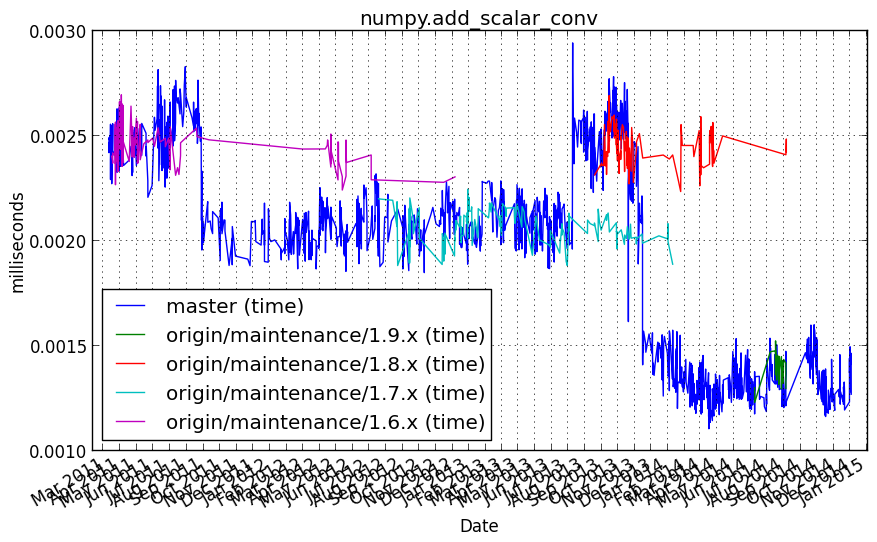
numpy.add_scalar_conv_complex¶
Benchmark setup
from numpy_vb_common import *
x = numpy.asarray(1.0+1j); y = complex(1., 1.)
Benchmark statement
x+y
Performance graph
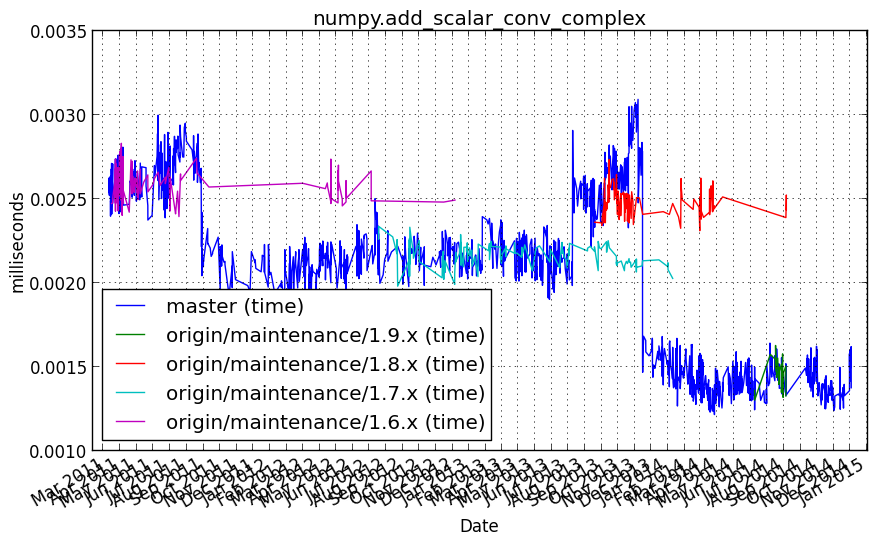
numpy.and_bool¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.bool)
Benchmark statement
d & d
Performance graph
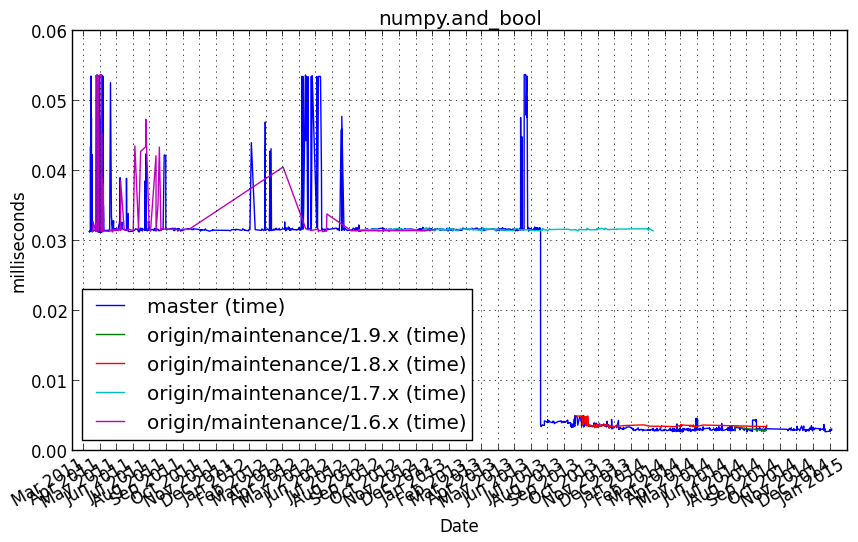
numpy.arccos(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arccos(a) for t, a in squares_.iteritems() if t in types]
Performance graph
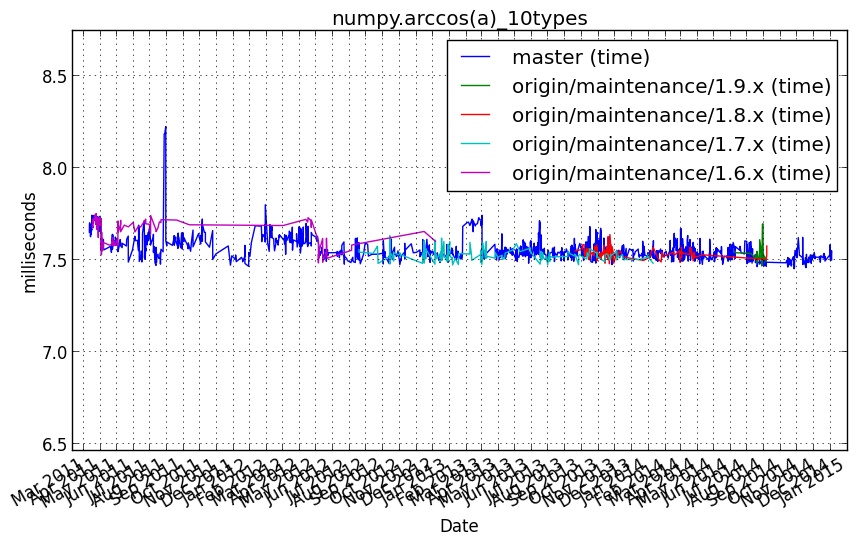
numpy.arccosh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arccosh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
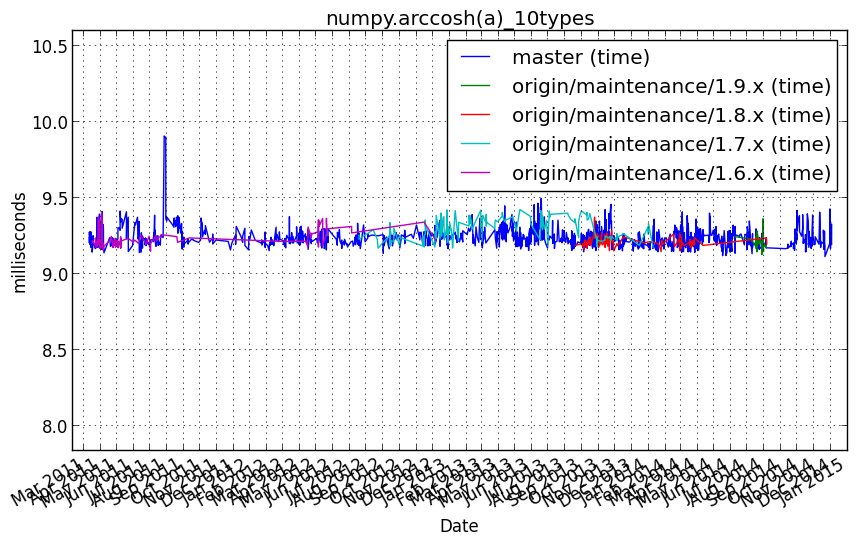
numpy.arcsin(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arcsin(a) for t, a in squares_.iteritems() if t in types]
Performance graph
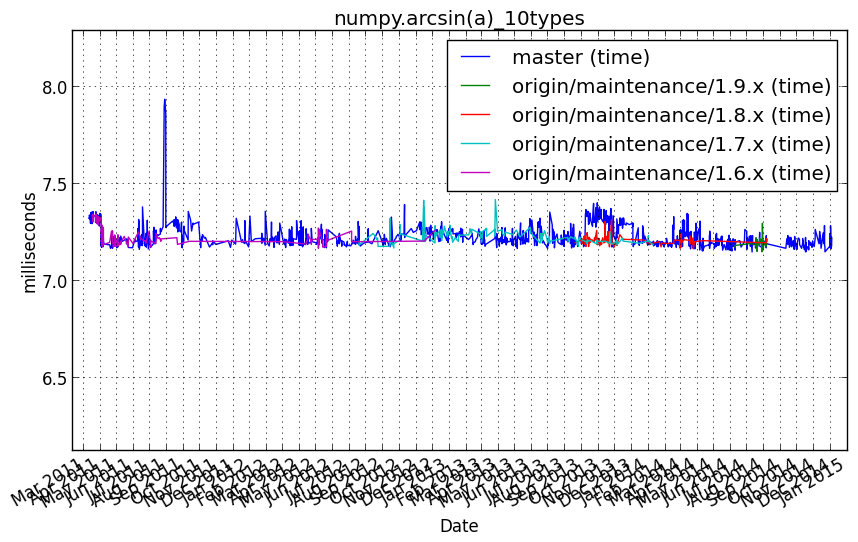
numpy.arcsinh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arcsinh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
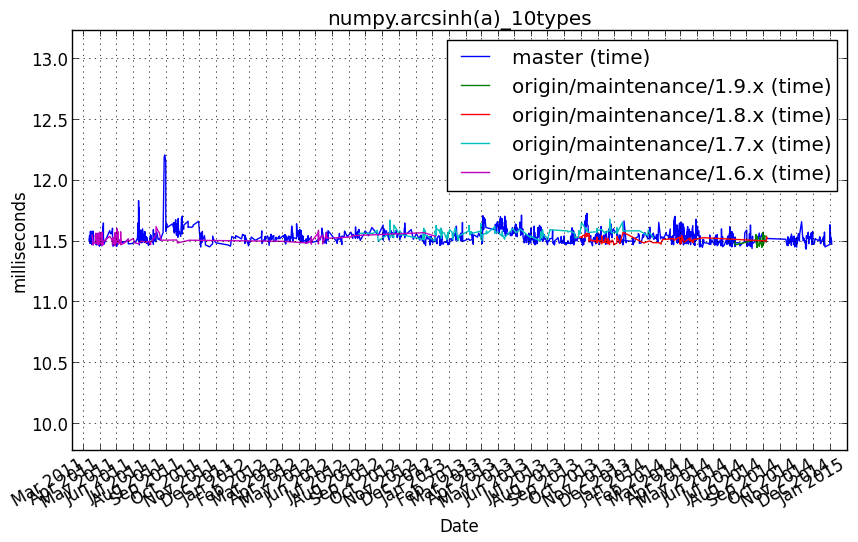
numpy.arctan(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arctan(a) for t, a in squares_.iteritems() if t in types]
Performance graph
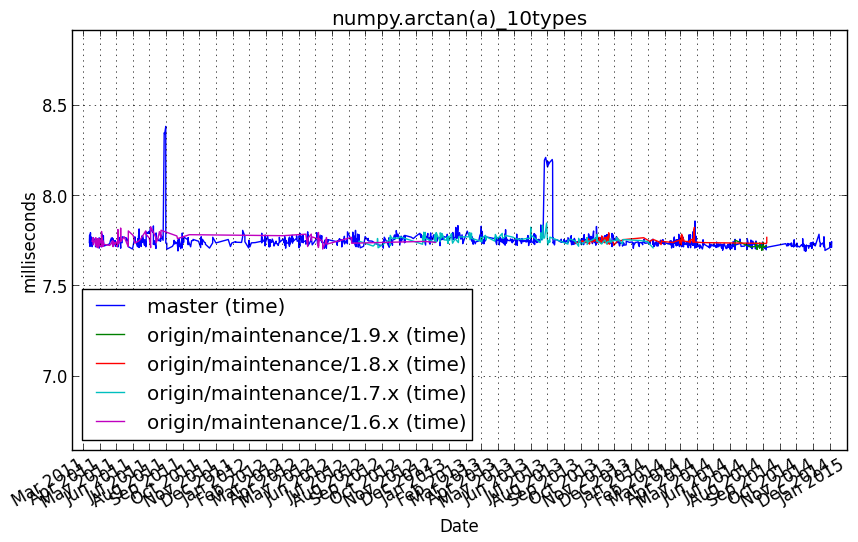
numpy.arctan2(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.arctan2(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
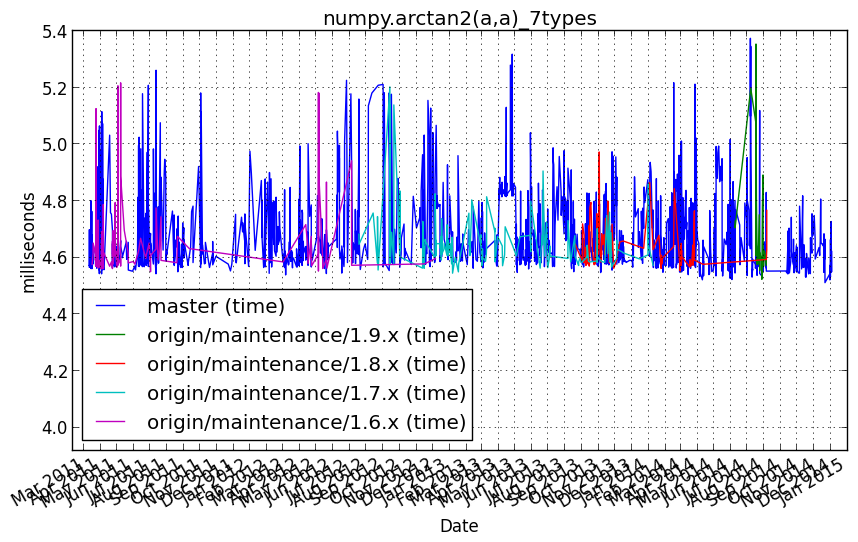
numpy.arctanh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.arctanh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
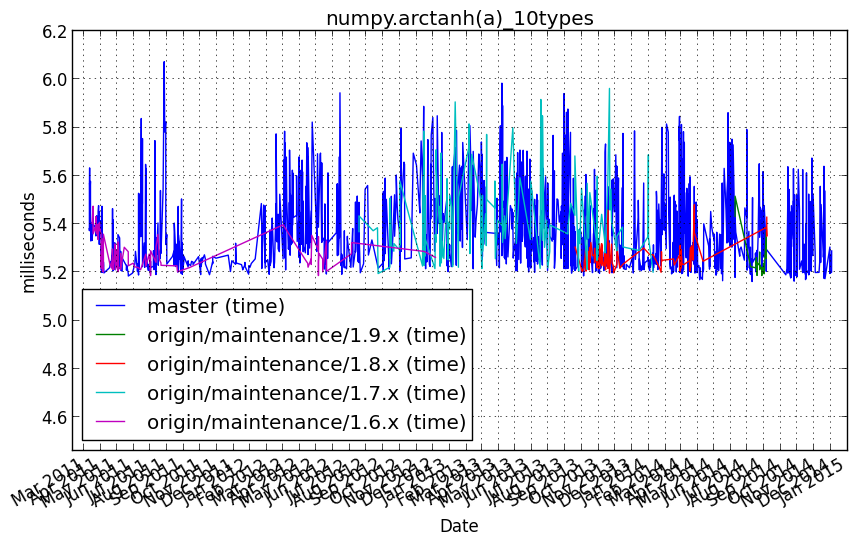
numpy.bitwise_and(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.bitwise_and(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
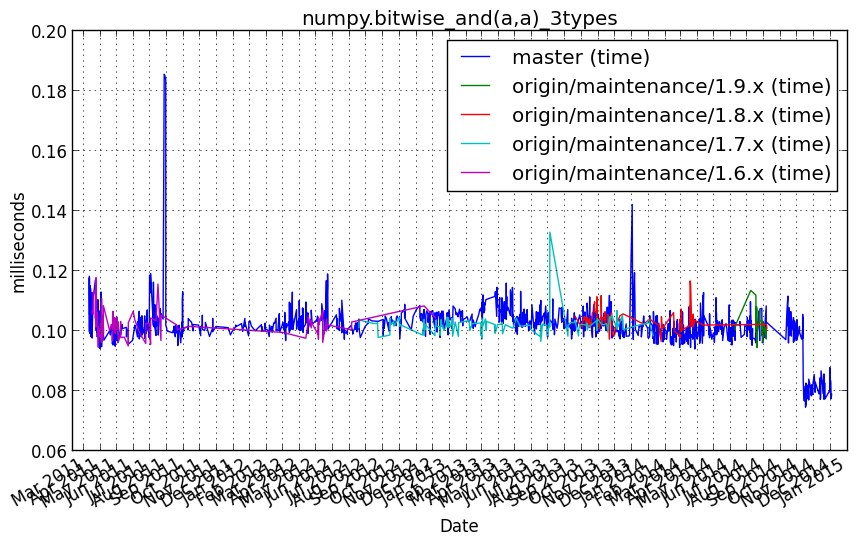
numpy.bitwise_not(a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.bitwise_not(a) for t, a in squares_.iteritems() if t in types]
Performance graph
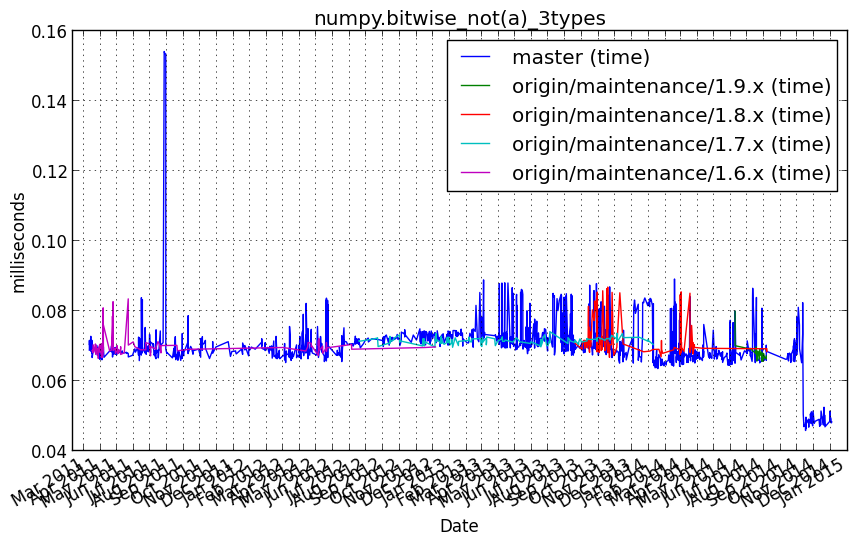
numpy.bitwise_or(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.bitwise_or(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
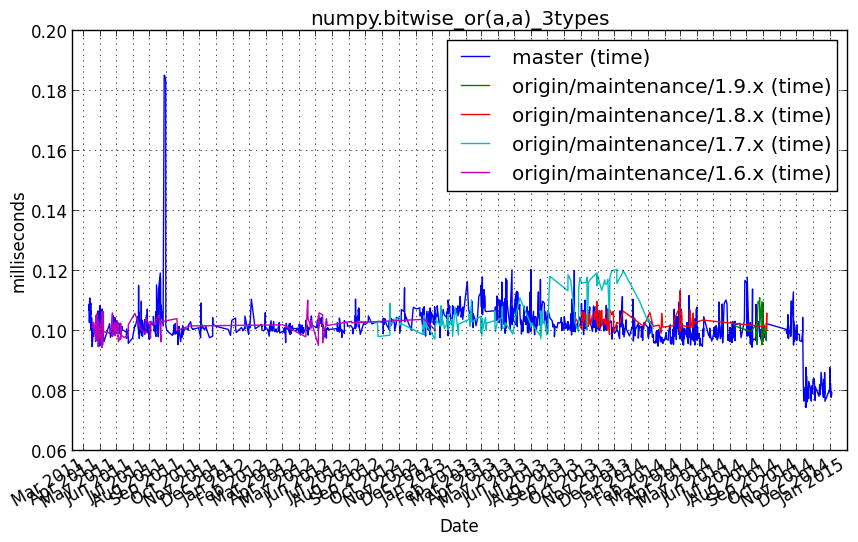
numpy.bitwise_xor(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.bitwise_xor(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
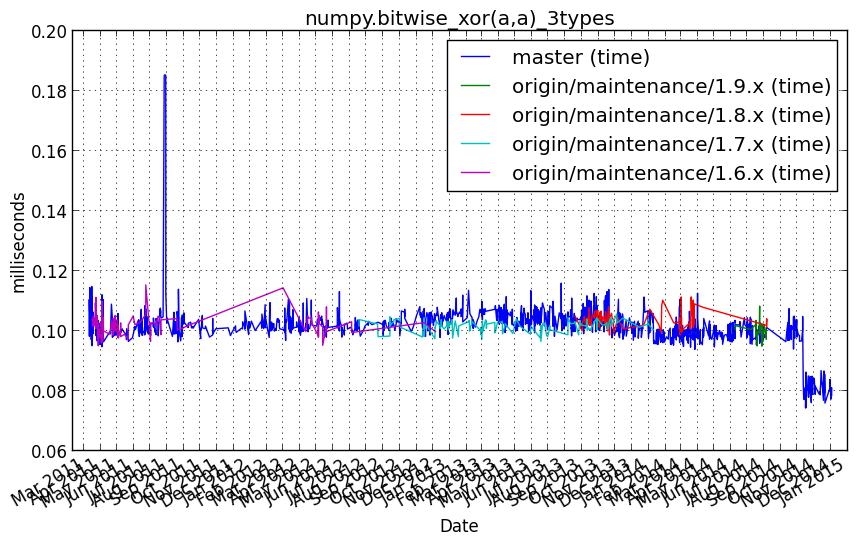
numpy.broadcast¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones((50000, 100), dtype=numpy.float64)
e = numpy.ones((100,), dtype=numpy.float64)
Benchmark statement
d - e
Performance graph
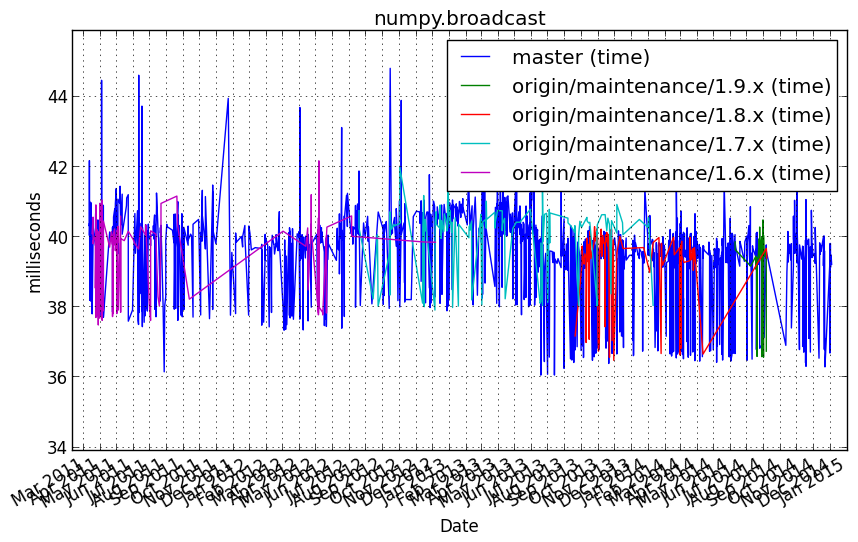
numpy.ceil(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.ceil(a) for t, a in squares_.iteritems() if t in types]
Performance graph
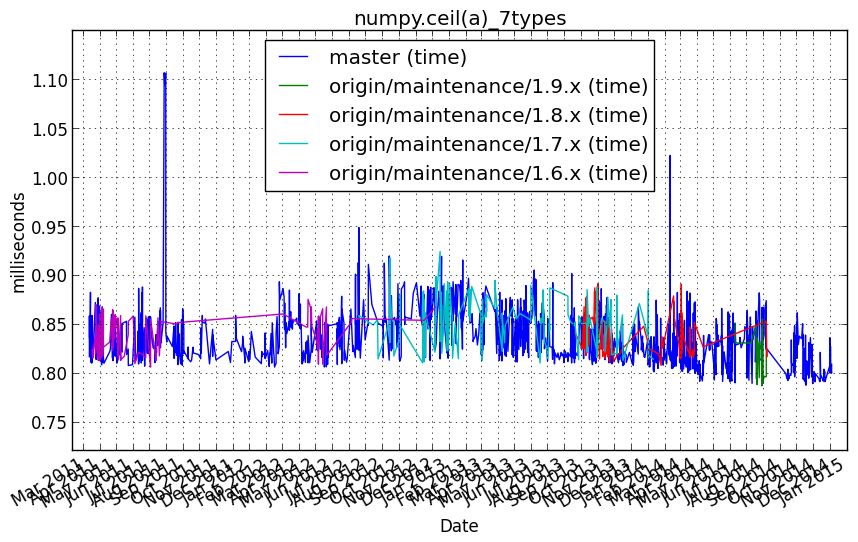
numpy.conj(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.conj(a) for t, a in squares_.iteritems() if t in types]
Performance graph
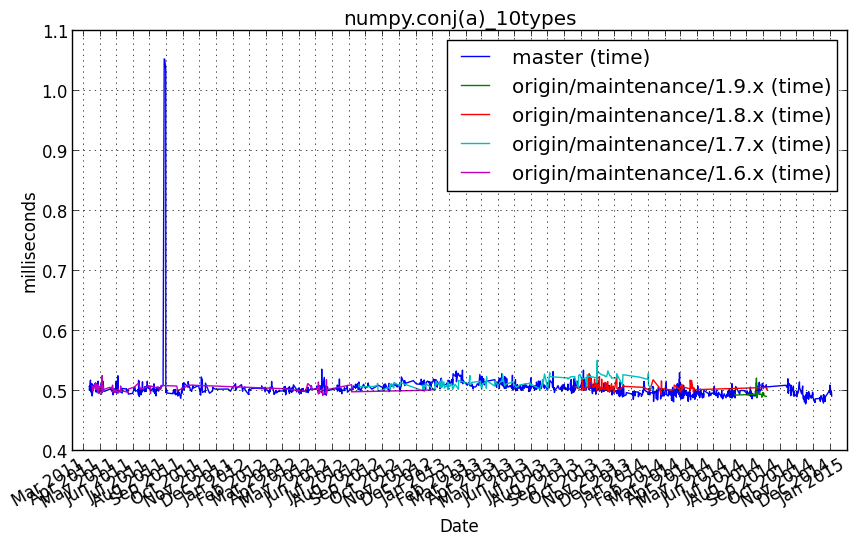
numpy.conjugate(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.conjugate(a) for t, a in squares_.iteritems() if t in types]
Performance graph
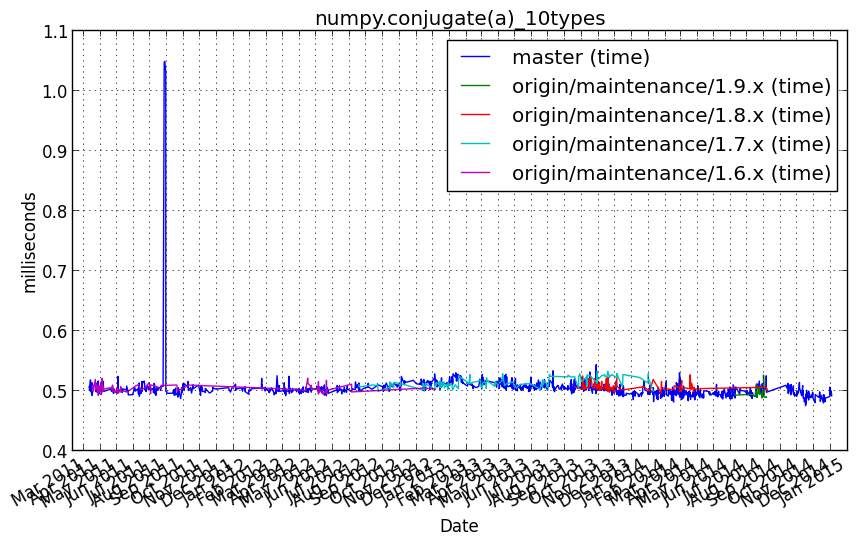
numpy.copysign(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.copysign(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
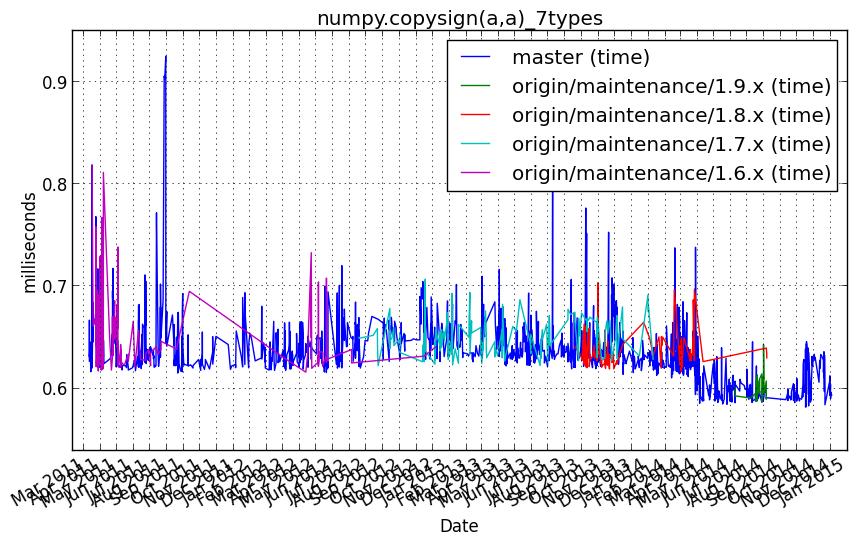
numpy.cos(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.cos(a) for t, a in squares_.iteritems() if t in types]
Performance graph
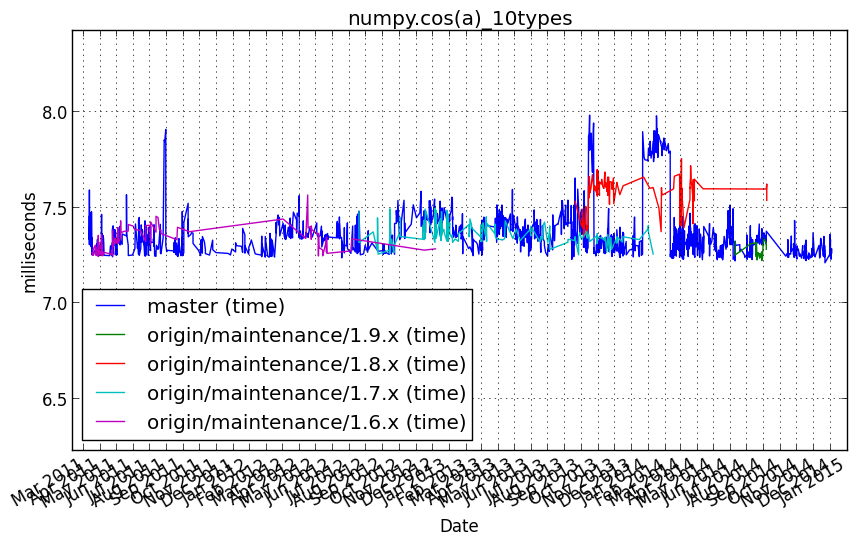
numpy.cosh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.cosh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
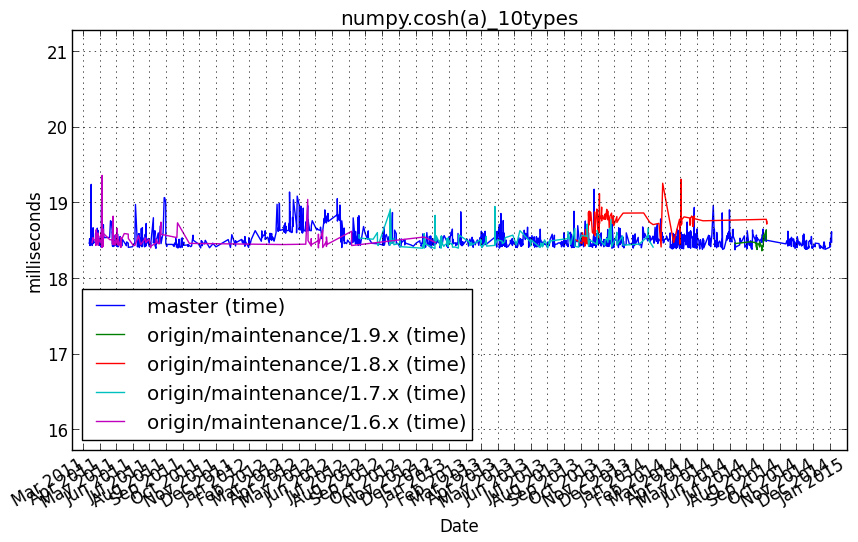
numpy.count_nonzero¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.bool)
Benchmark statement
numpy.count_nonzero(d)
Performance graph
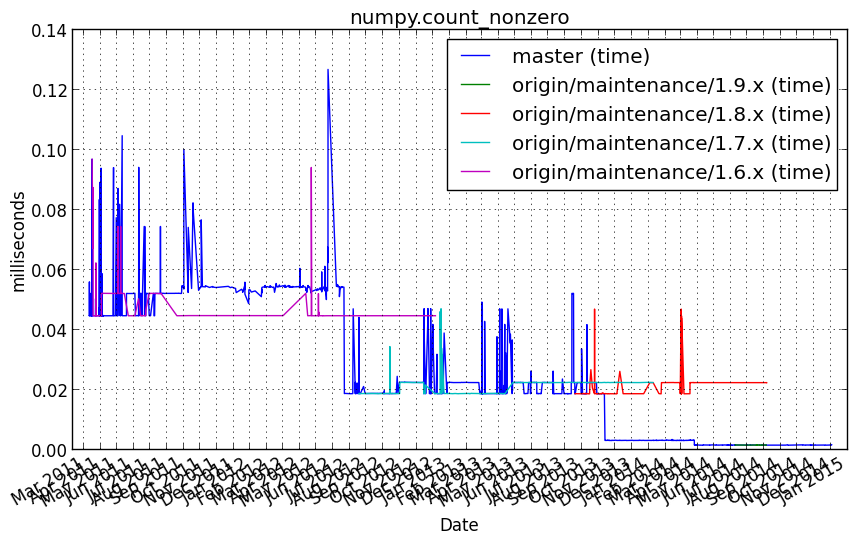
numpy.deg2rad(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.deg2rad(a) for t, a in squares_.iteritems() if t in types]
Performance graph
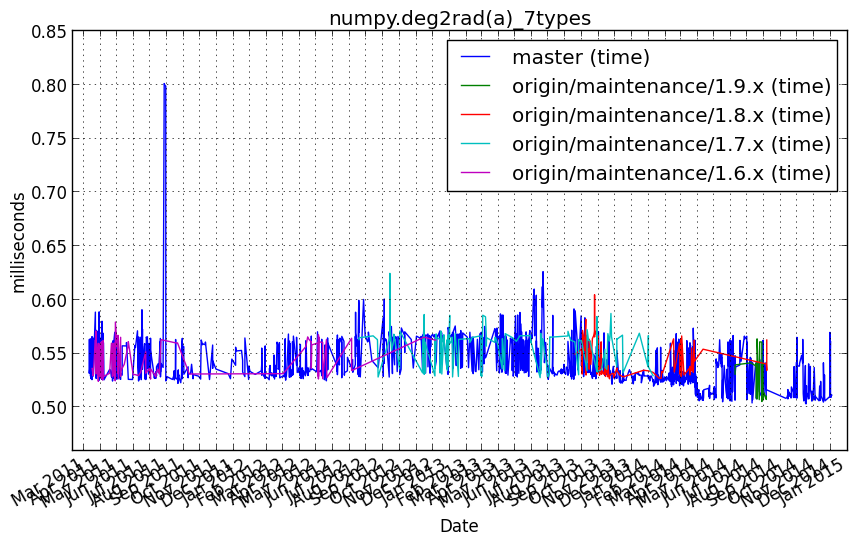
numpy.degrees(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.degrees(a) for t, a in squares_.iteritems() if t in types]
Performance graph
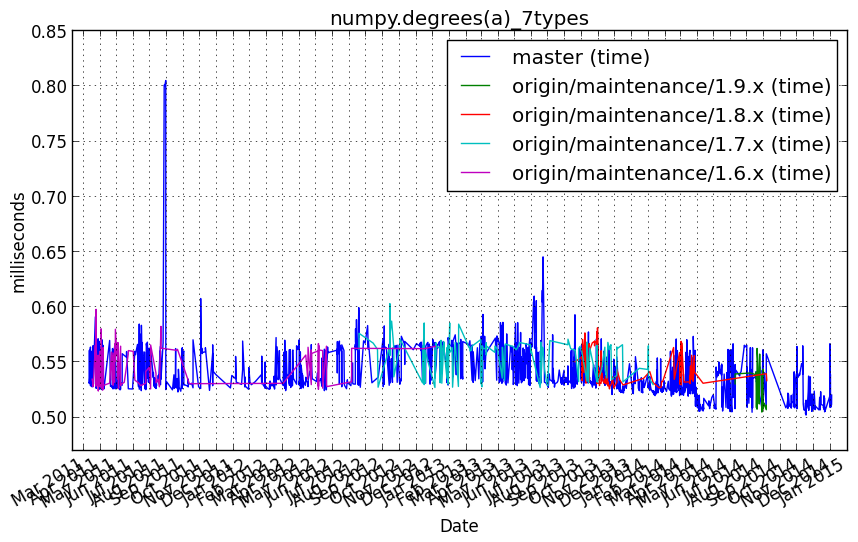
numpy.divide(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.divide(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
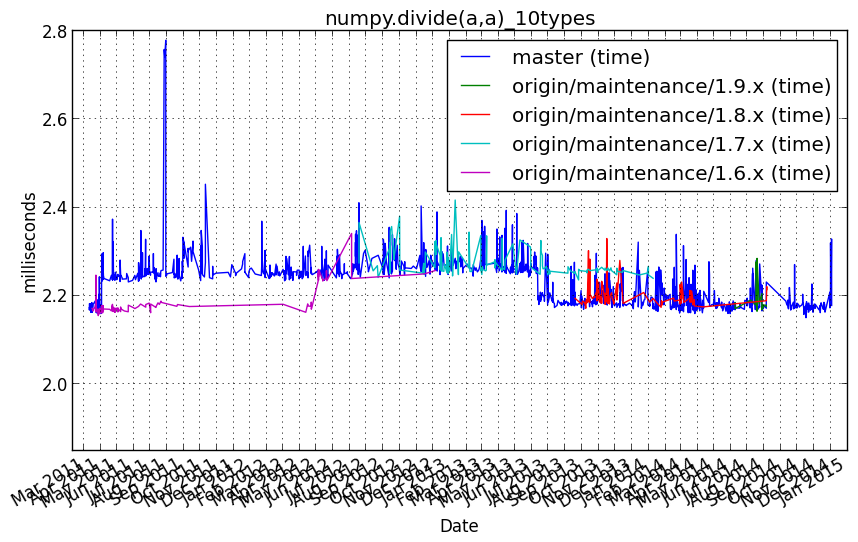
numpy.divide_scalar2_inplace_numpy.float32¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float32)
Benchmark statement
numpy.divide(d, 1, out=d)
Performance graph
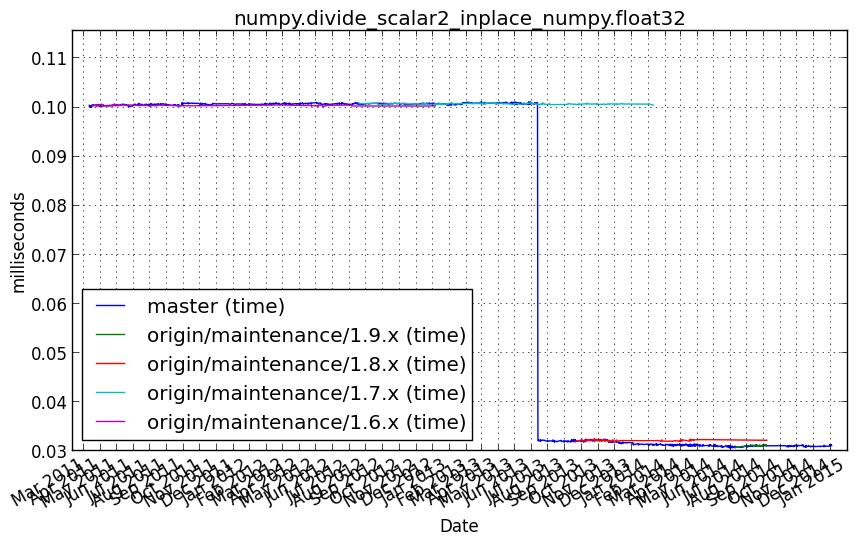
numpy.divide_scalar2_inplace_numpy.float64¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float64)
Benchmark statement
numpy.divide(d, 1, out=d)
Performance graph
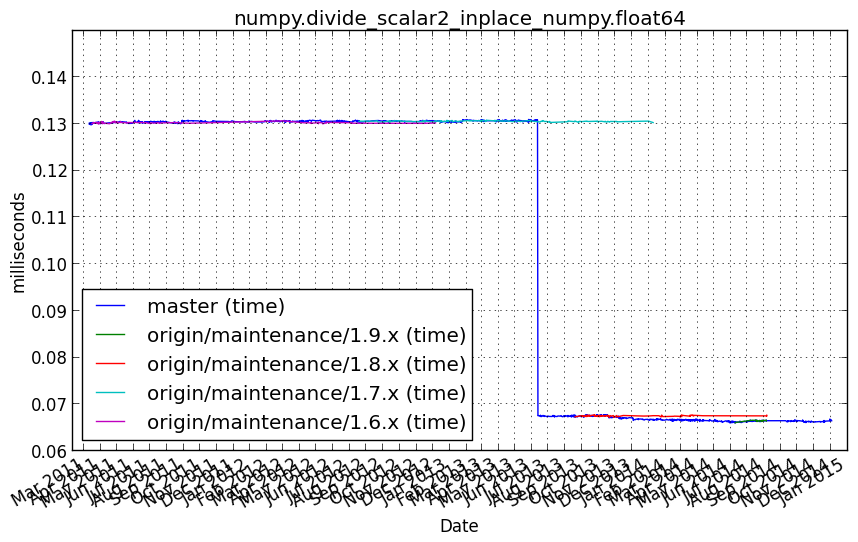
numpy.divide_scalar2_numpy.float32¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float32)
Benchmark statement
numpy.divide(d, 1)
Performance graph
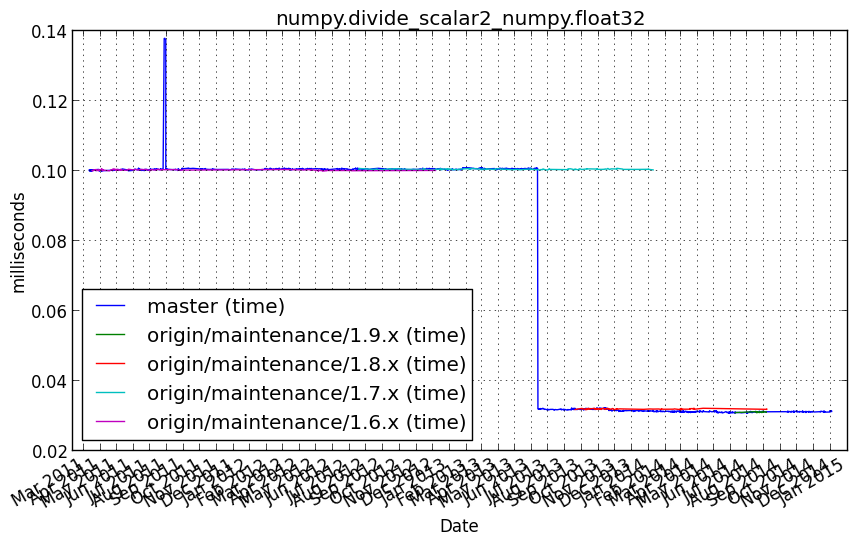
numpy.divide_scalar2_numpy.float64¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float64)
Benchmark statement
numpy.divide(d, 1)
Performance graph
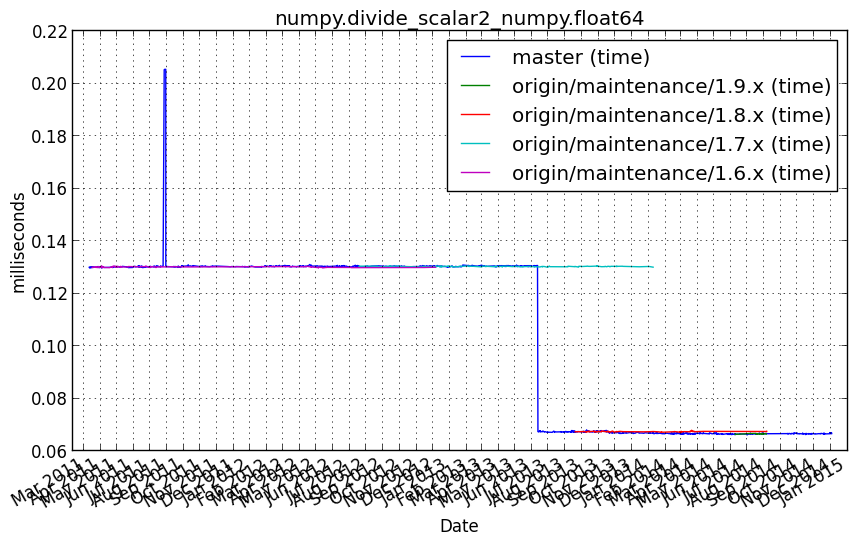
numpy.equal(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.equal(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
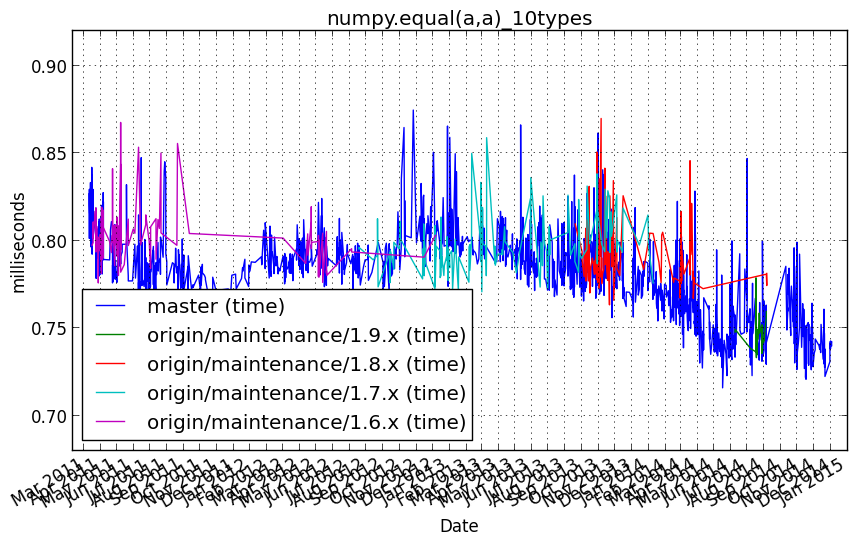
numpy.exp(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.exp(a) for t, a in squares_.iteritems() if t in types]
Performance graph
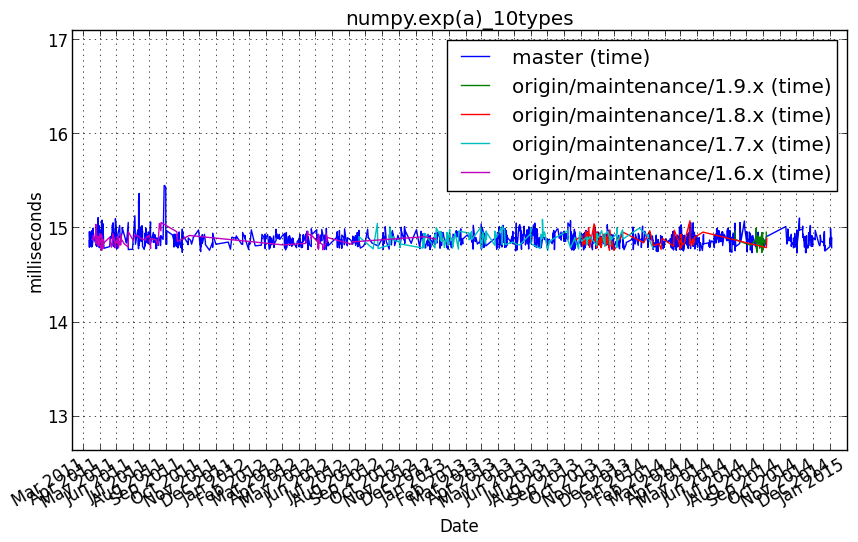
numpy.exp2(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.exp2(a) for t, a in squares_.iteritems() if t in types]
Performance graph
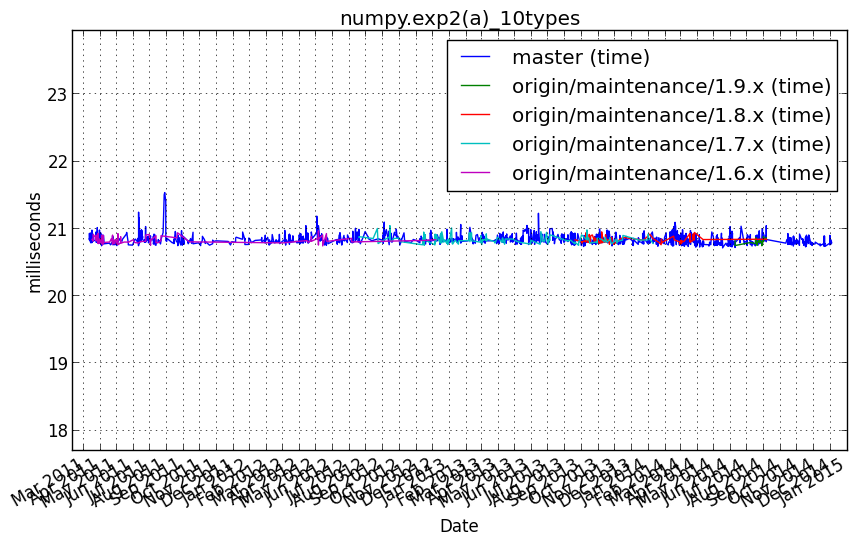
numpy.expm1(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.expm1(a) for t, a in squares_.iteritems() if t in types]
Performance graph
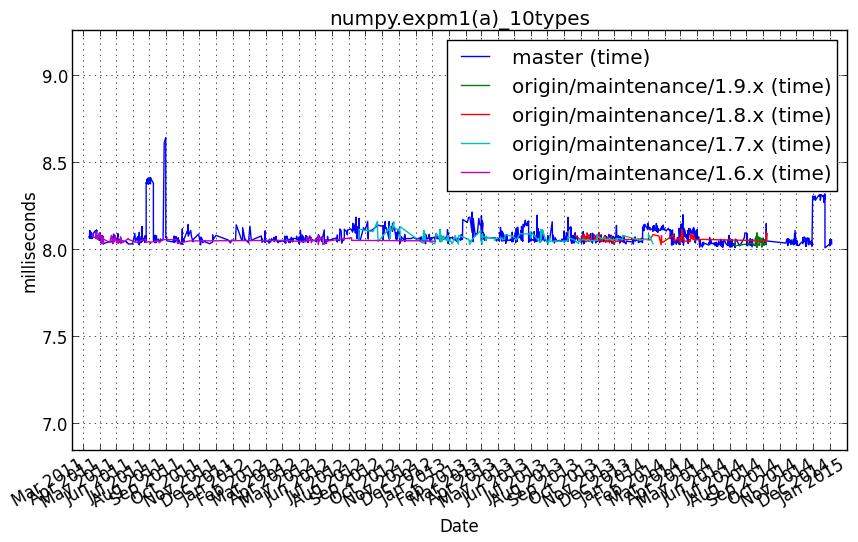
numpy.fabs(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.fabs(a) for t, a in squares_.iteritems() if t in types]
Performance graph
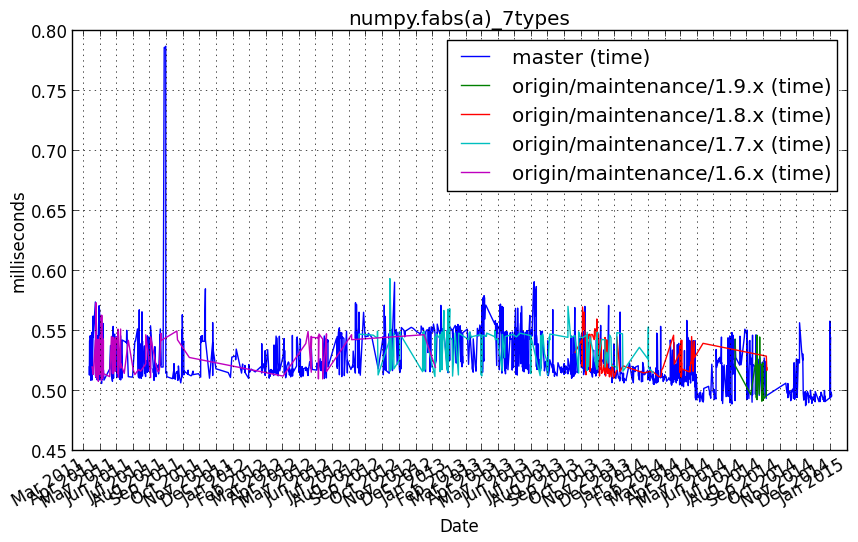
numpy.floor(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.floor(a) for t, a in squares_.iteritems() if t in types]
Performance graph
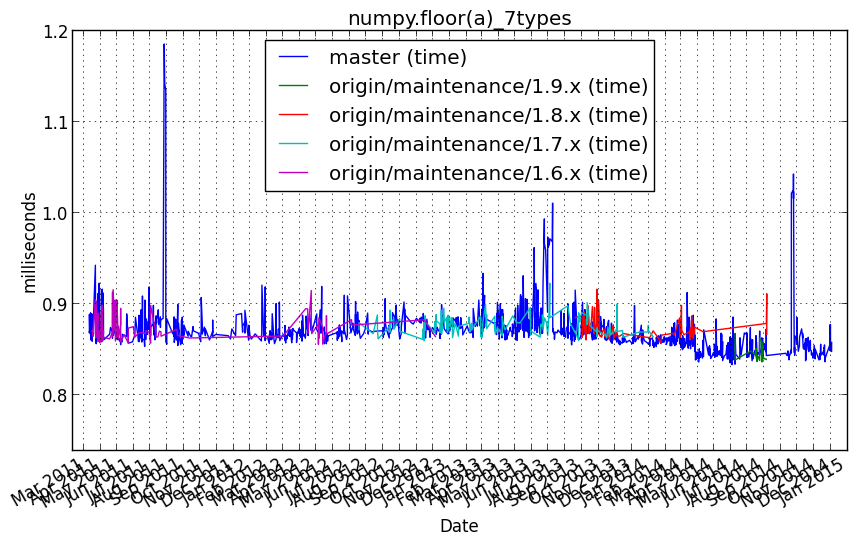
numpy.floor_divide(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.floor_divide(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
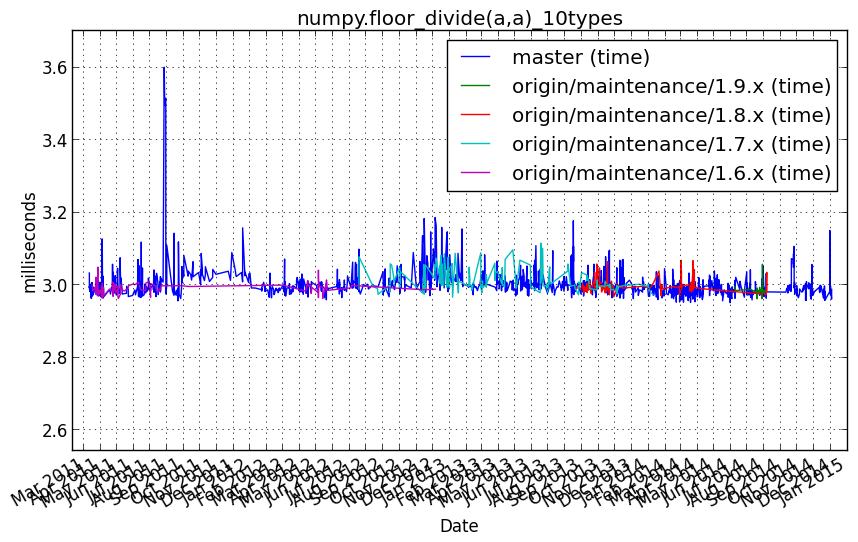
numpy.fmax(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.fmax(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
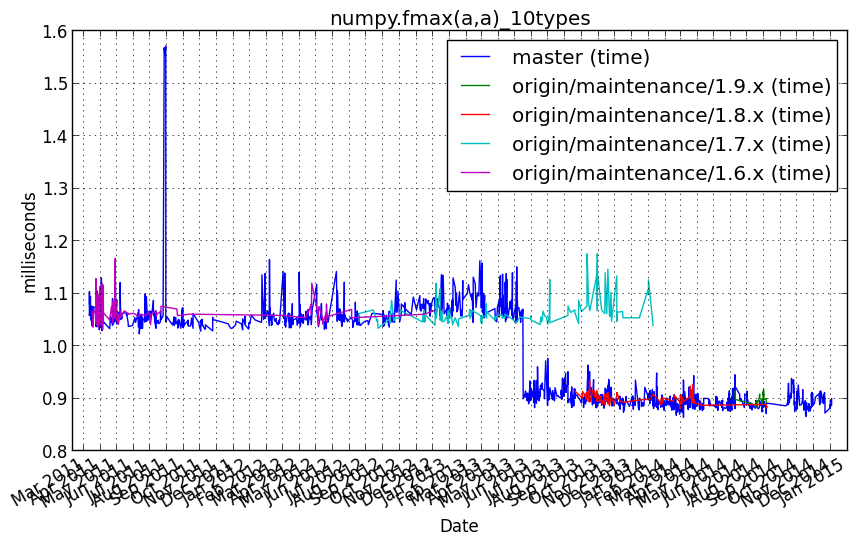
numpy.fmin(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.fmin(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
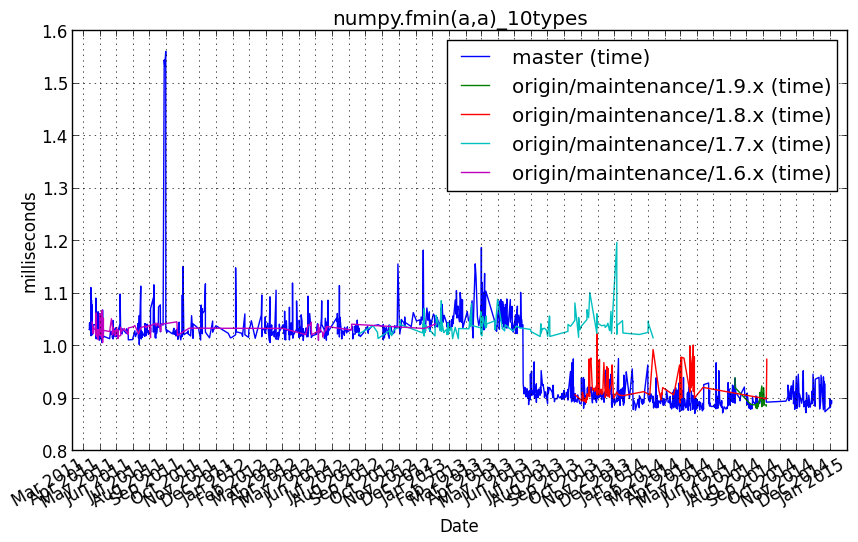
numpy.fmod(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.fmod(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
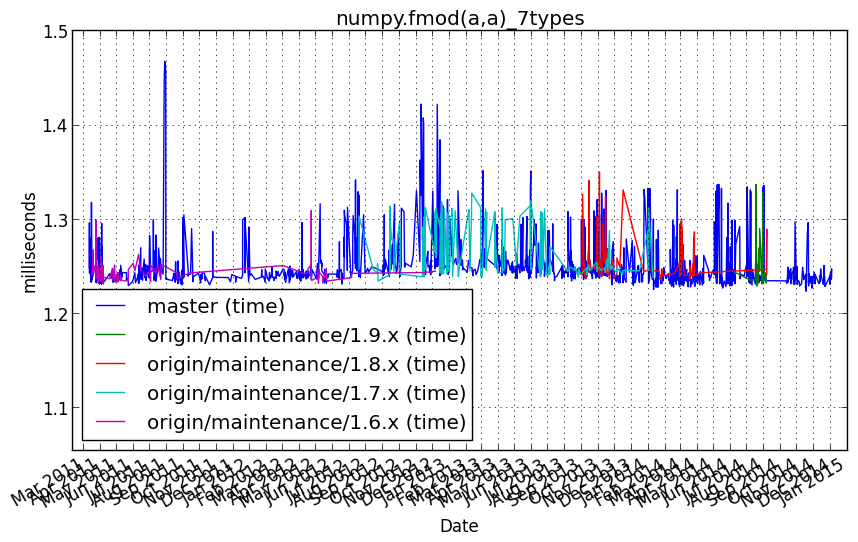
numpy.frexp(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.frexp(a) for t, a in squares_.iteritems() if t in types]
Performance graph
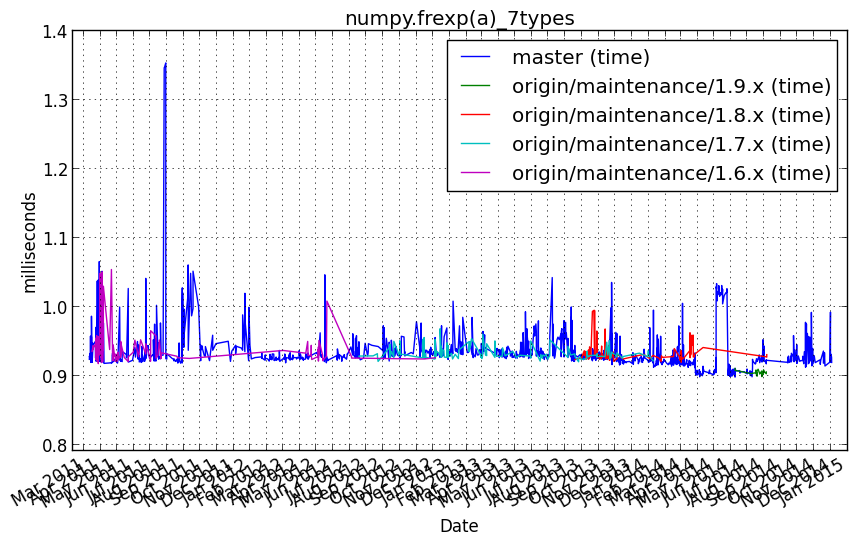
numpy.greater(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.greater(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
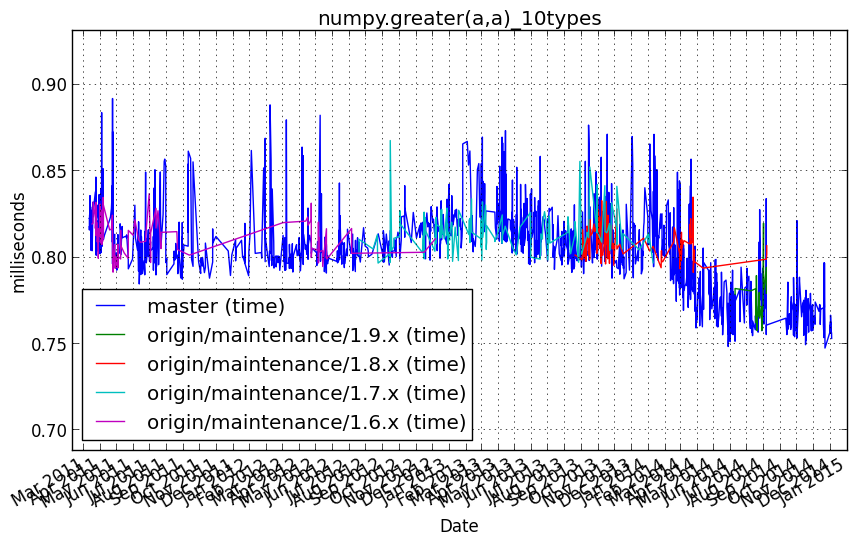
numpy.greater_equal(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.greater_equal(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
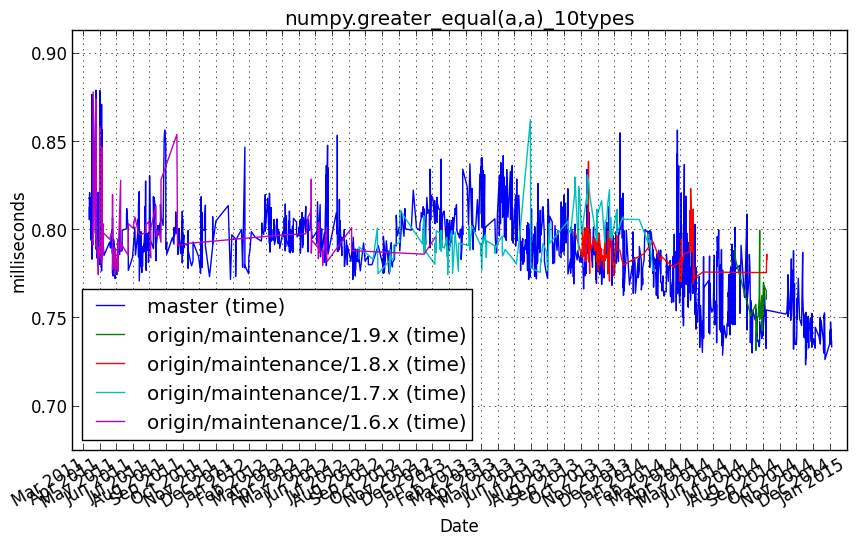
numpy.hypot(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.hypot(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
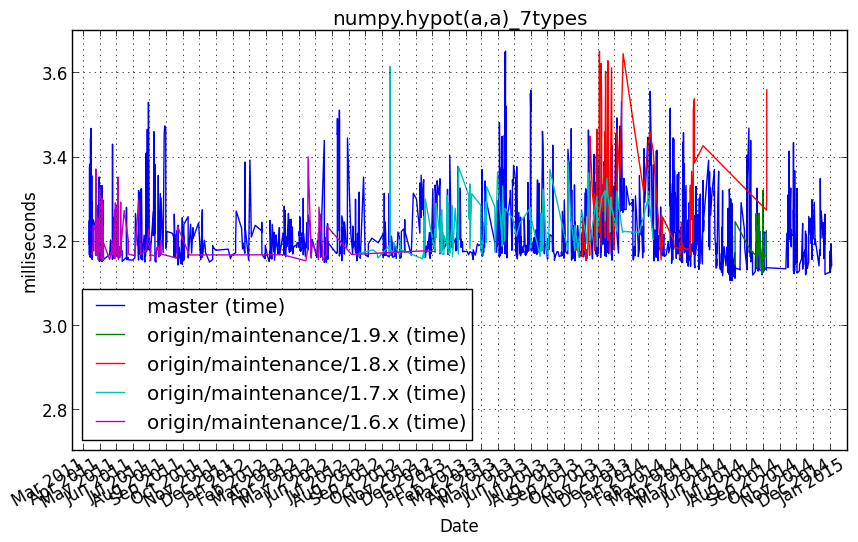
numpy.invert(a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.invert(a) for t, a in squares_.iteritems() if t in types]
Performance graph
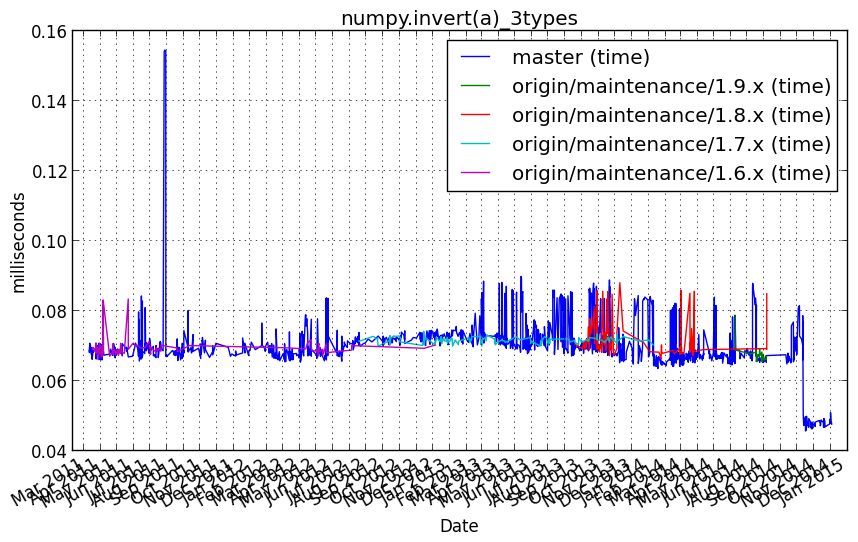
numpy.isfinite(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.isfinite(a) for t, a in squares_.iteritems() if t in types]
Performance graph
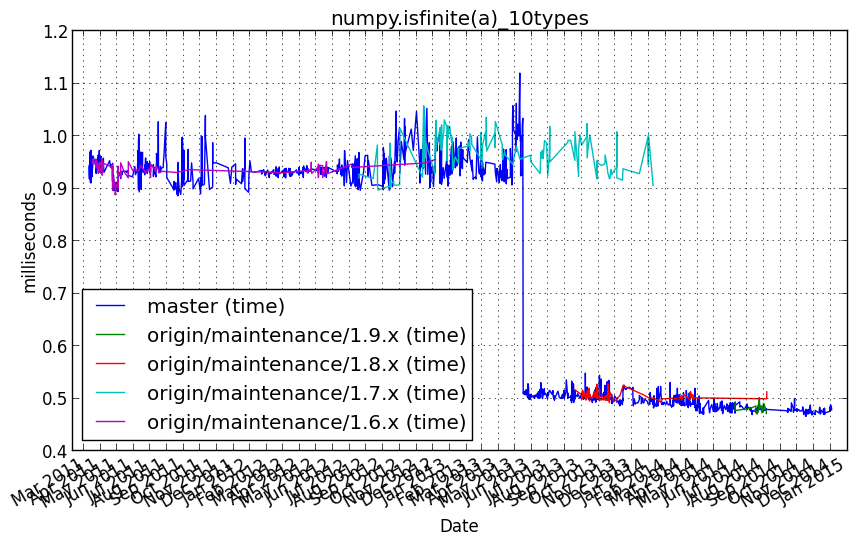
numpy.isinf(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.isinf(a) for t, a in squares_.iteritems() if t in types]
Performance graph
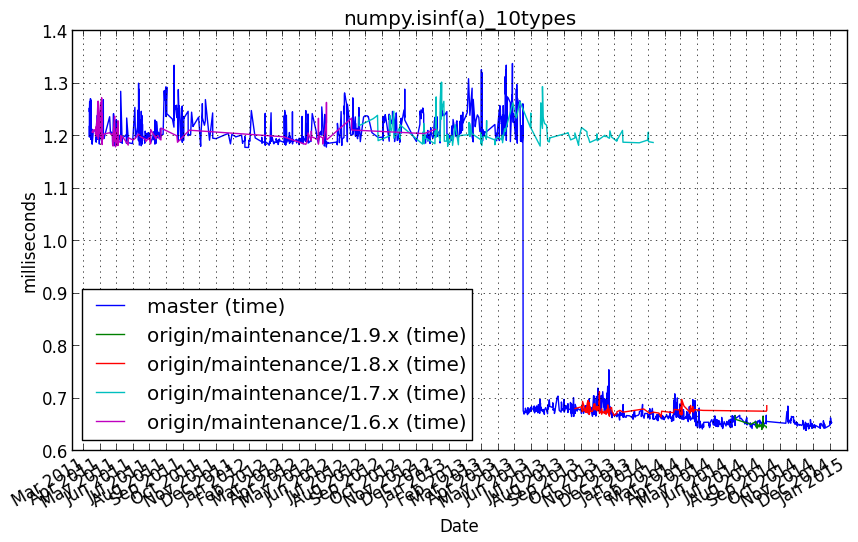
numpy.isnan(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.isnan(a) for t, a in squares_.iteritems() if t in types]
Performance graph
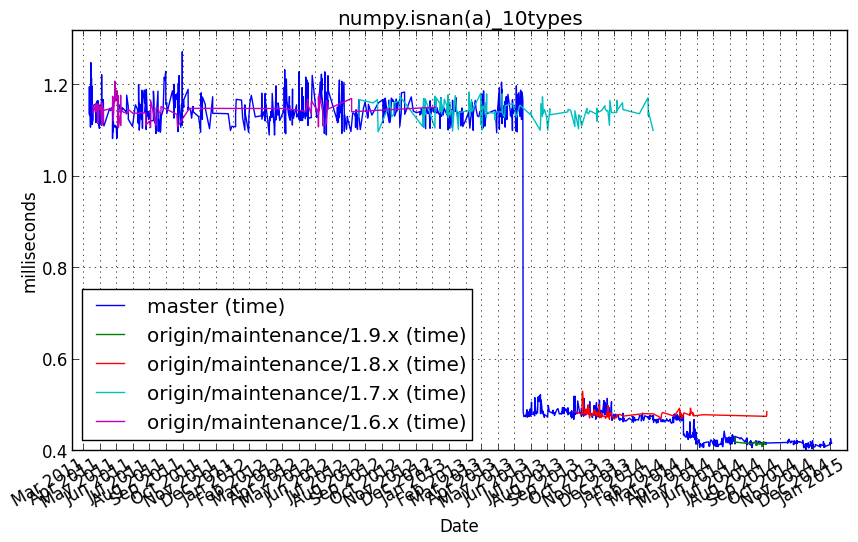
numpy.ldexp(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.ldexp(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
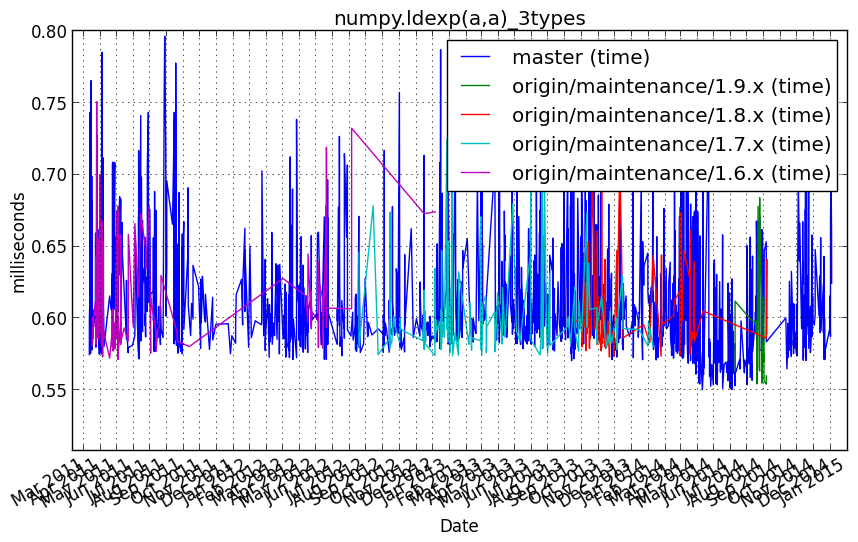
numpy.left_shift(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.left_shift(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
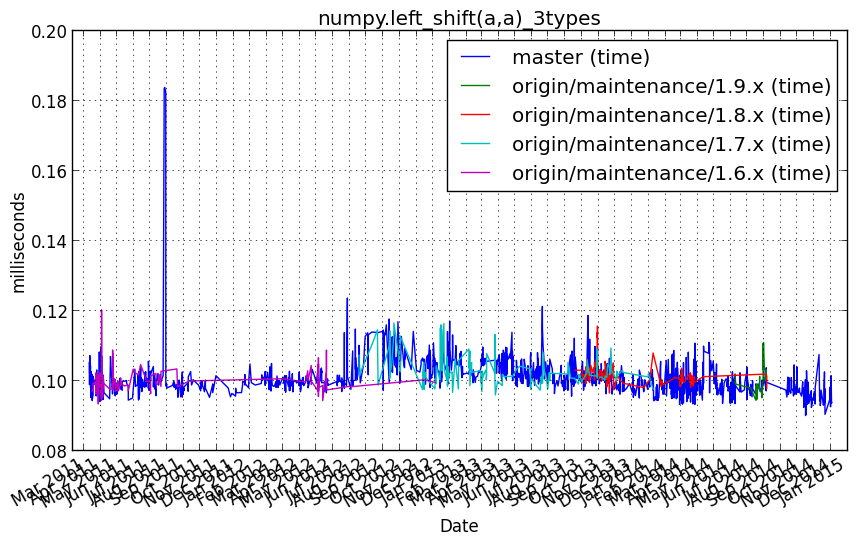
numpy.less(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.less(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
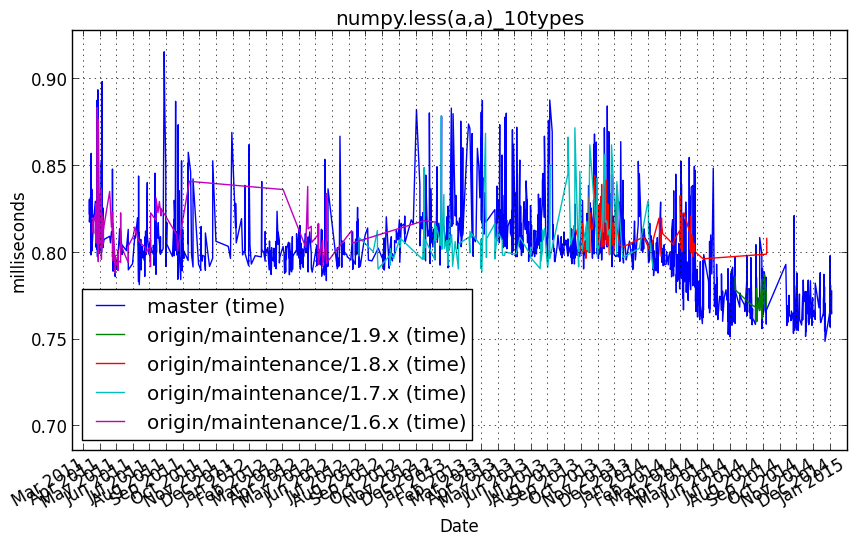
numpy.less_equal(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.less_equal(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
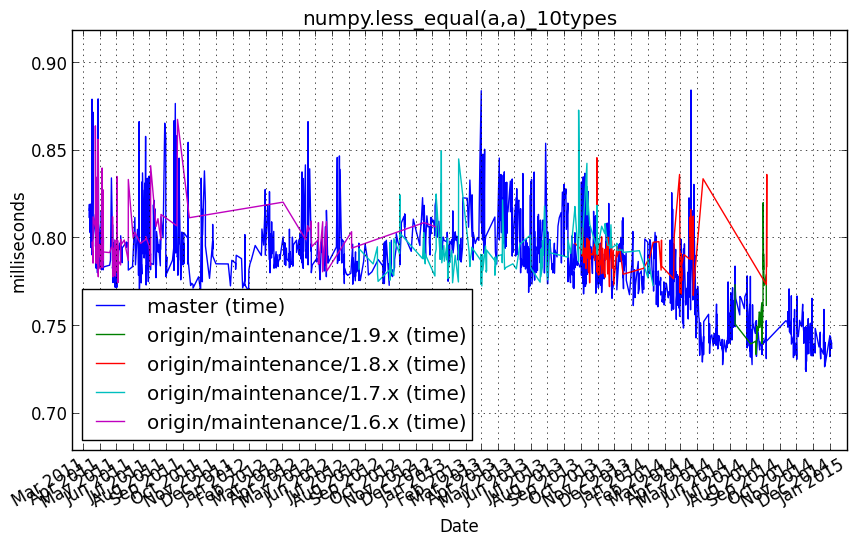
numpy.less_than_scalar2_numpy.float32¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float32)
Benchmark statement
d < 1
Performance graph
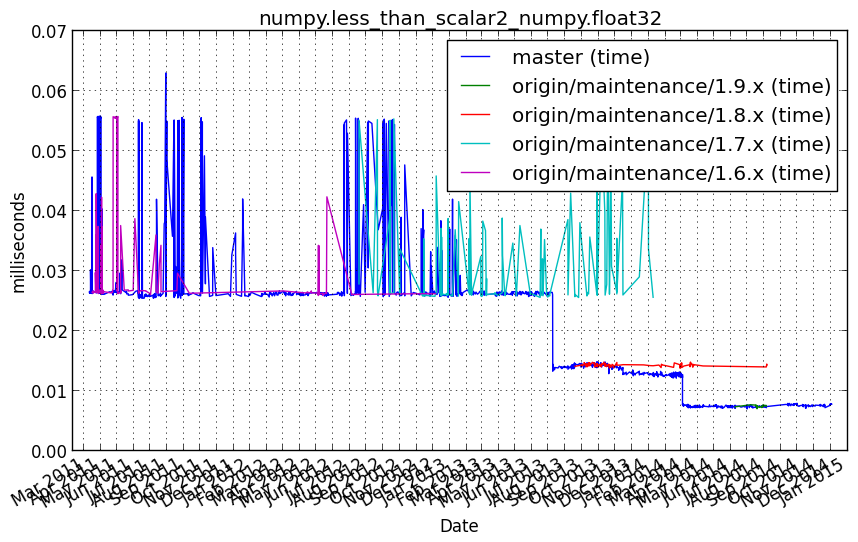
numpy.less_than_scalar2_numpy.float64¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.float64)
Benchmark statement
d < 1
Performance graph
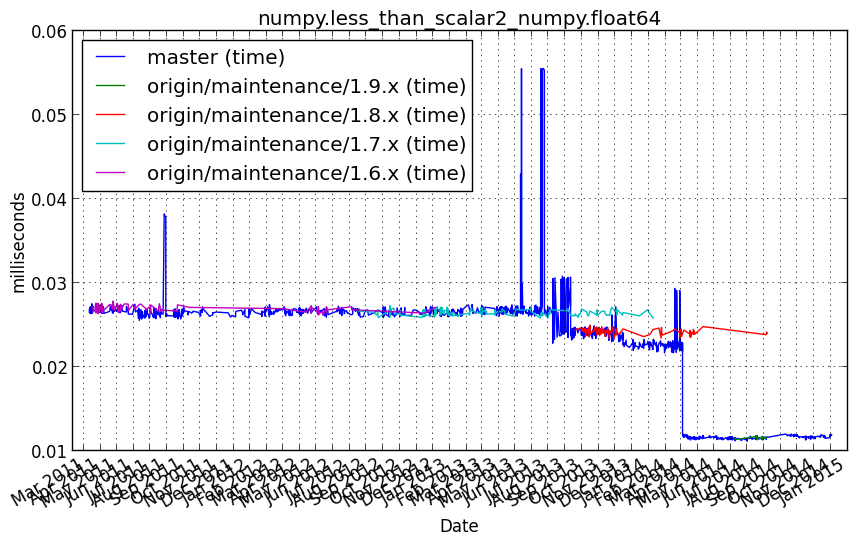
numpy.log(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.log(a) for t, a in squares_.iteritems() if t in types]
Performance graph
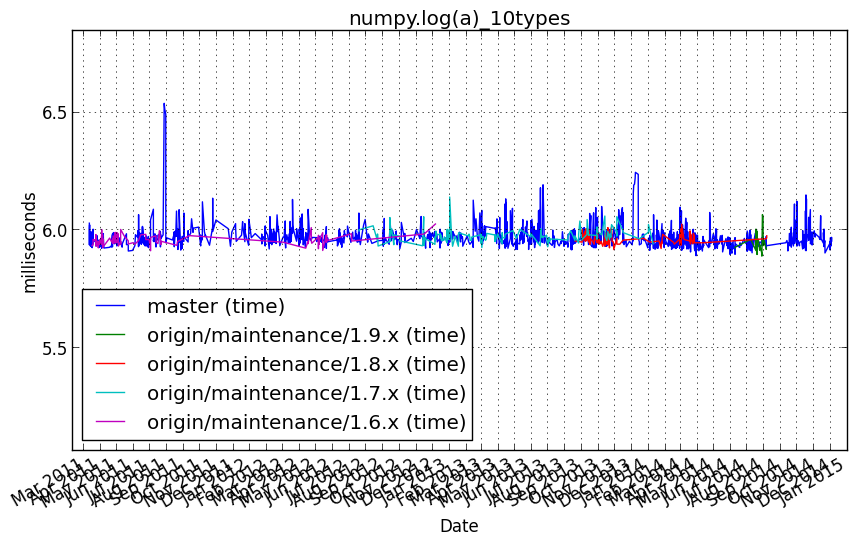
numpy.log10(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.log10(a) for t, a in squares_.iteritems() if t in types]
Performance graph
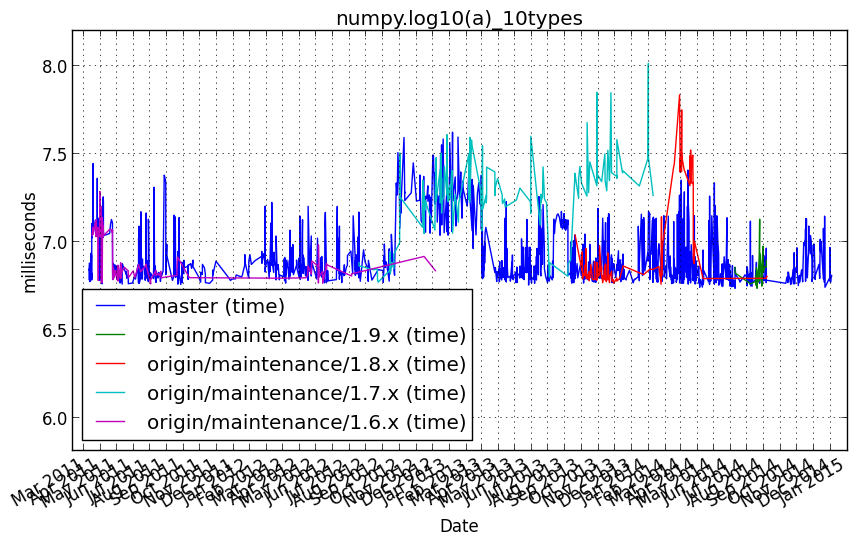
numpy.log1p(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.log1p(a) for t, a in squares_.iteritems() if t in types]
Performance graph
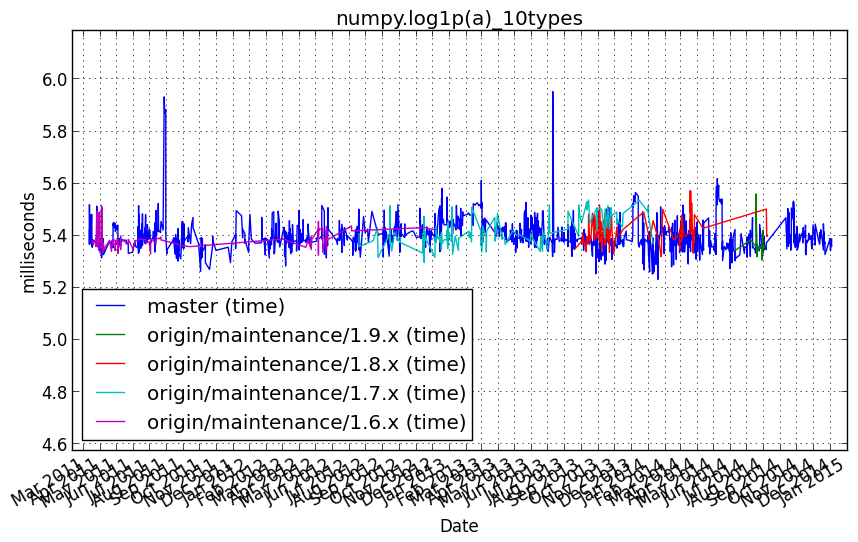
numpy.log2(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.log2(a) for t, a in squares_.iteritems() if t in types]
Performance graph
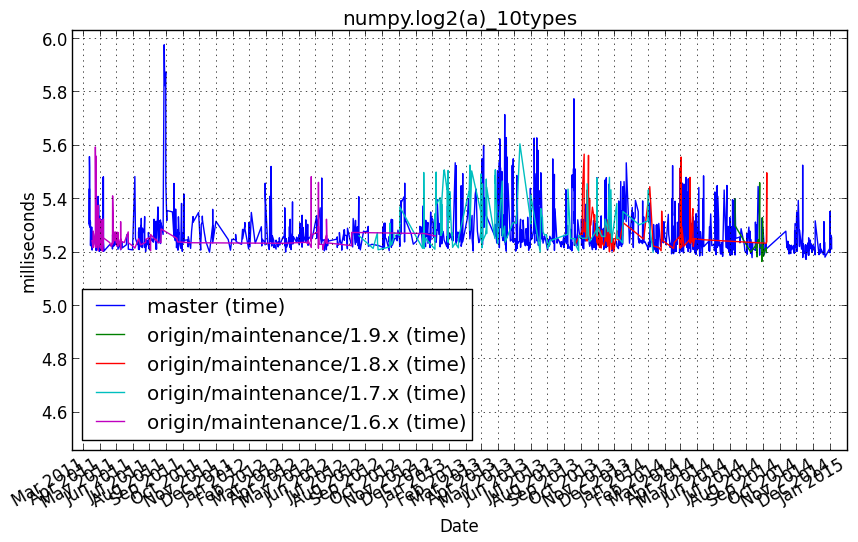
numpy.logaddexp(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.logaddexp(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
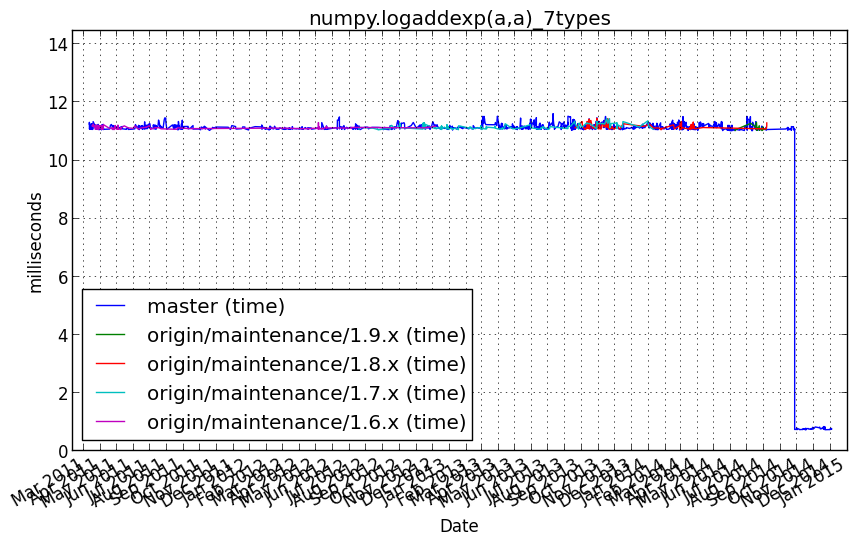
numpy.logaddexp2(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.logaddexp2(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
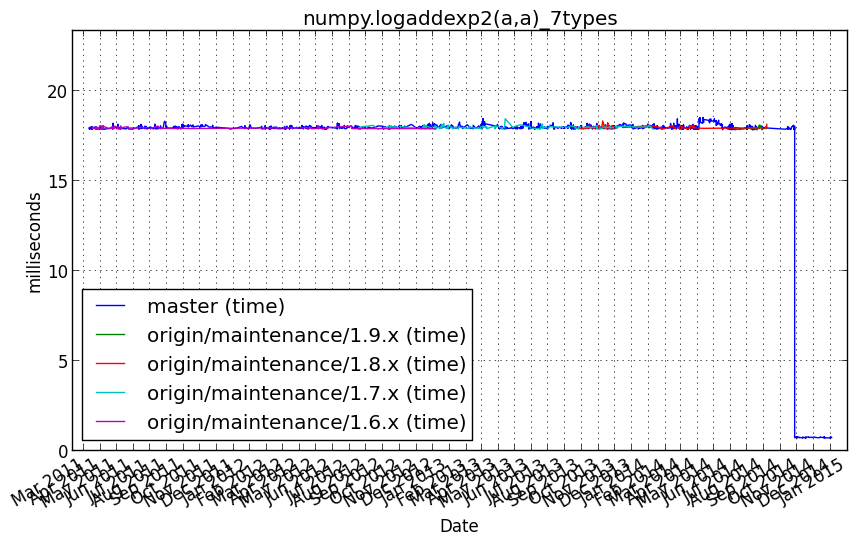
numpy.logical_and(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.logical_and(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
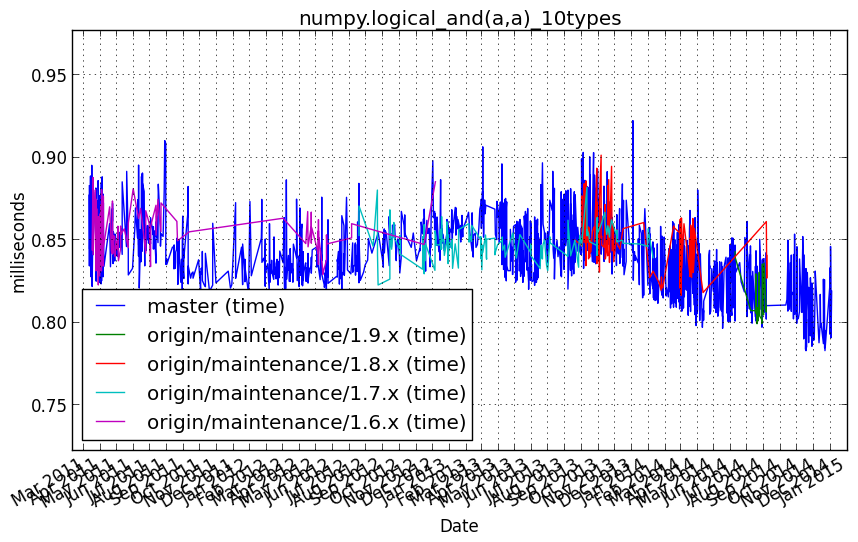
numpy.logical_not(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.logical_not(a) for t, a in squares_.iteritems() if t in types]
Performance graph
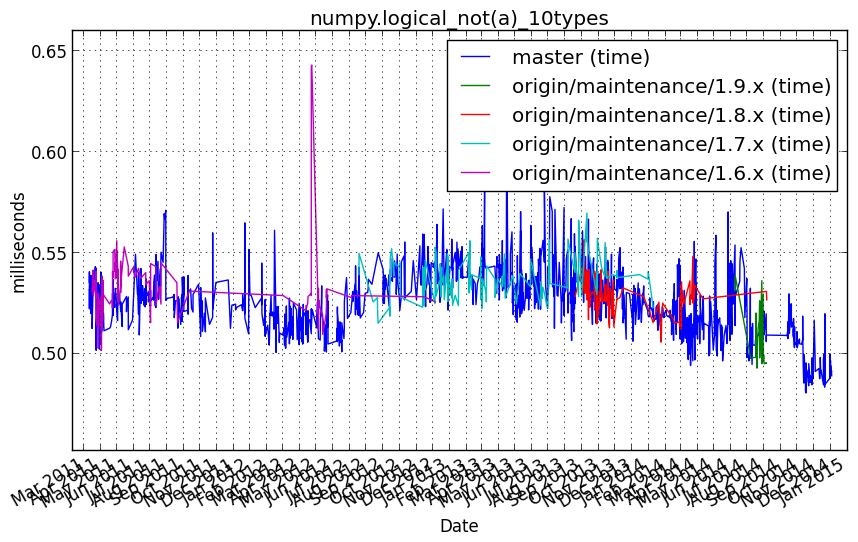
numpy.logical_or(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.logical_or(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
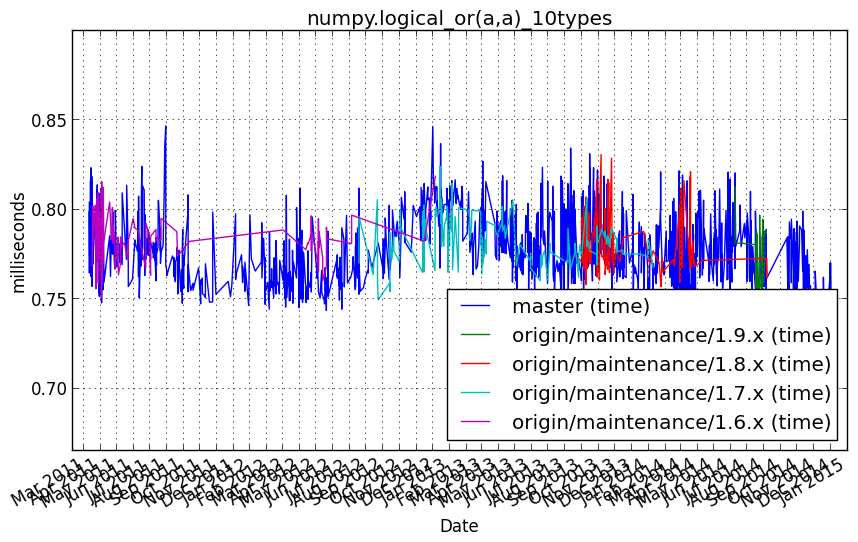
numpy.logical_xor(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.logical_xor(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
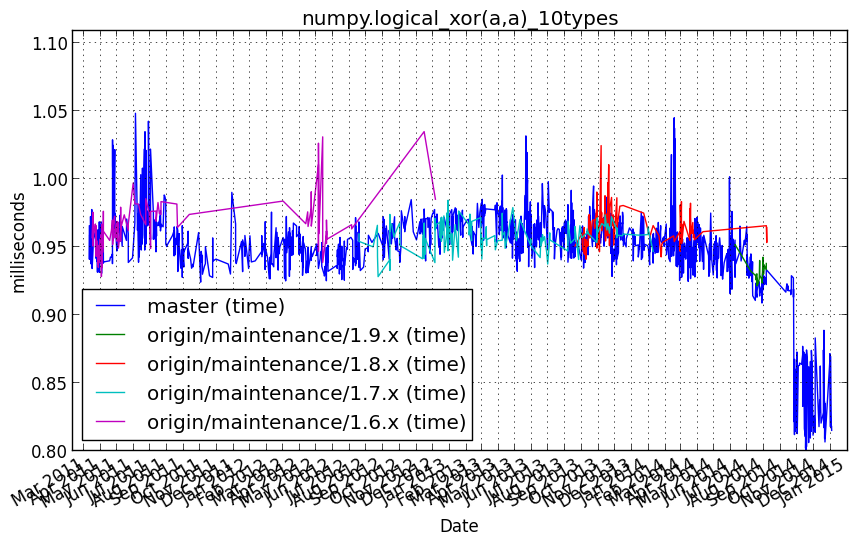
numpy.maximum(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.maximum(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
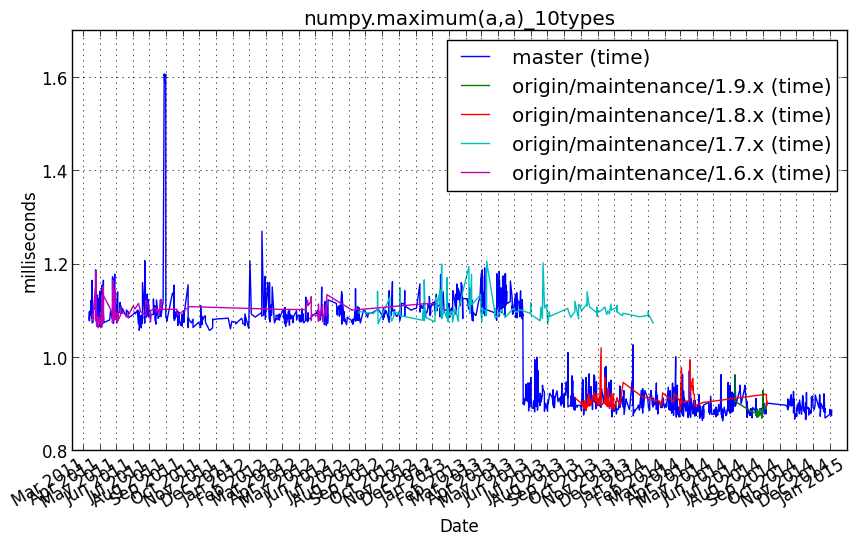
numpy.minimum(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.minimum(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
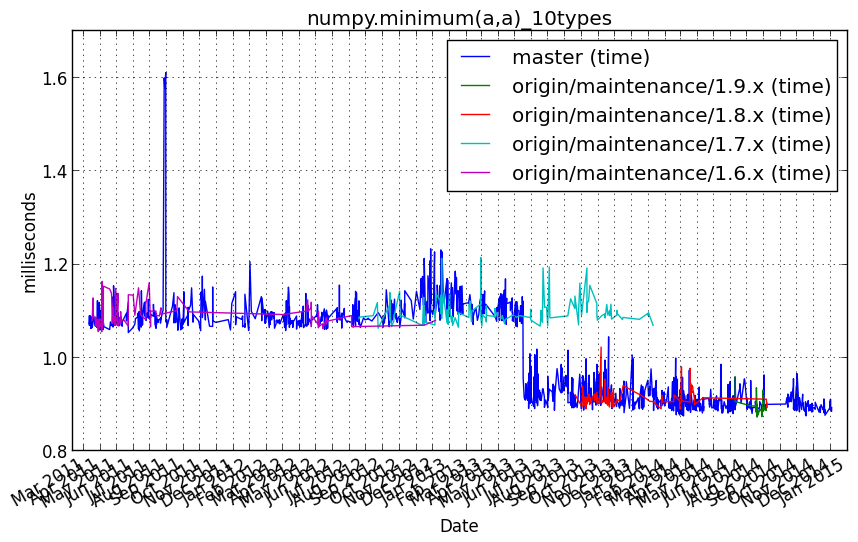
numpy.mod(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.mod(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
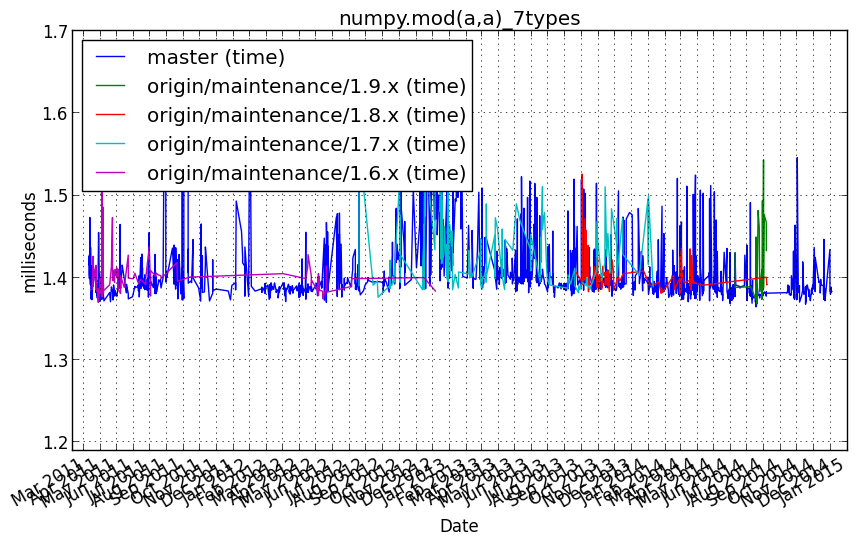
numpy.modf(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.modf(a) for t, a in squares_.iteritems() if t in types]
Performance graph
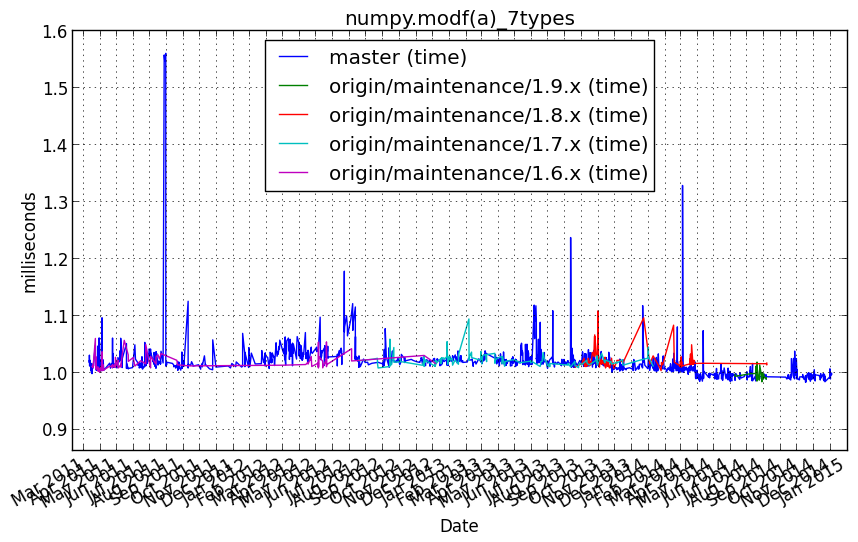
numpy.multiply(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.multiply(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
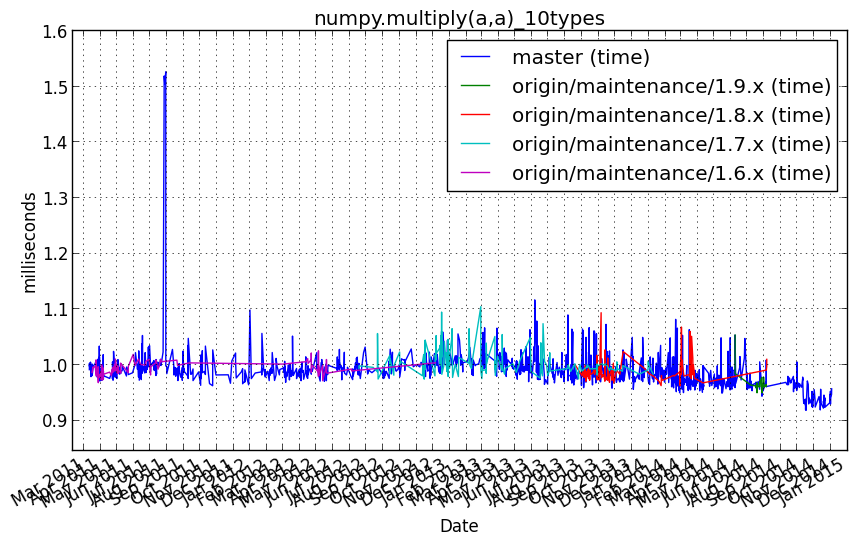
numpy.negative(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.negative(a) for t, a in squares_.iteritems() if t in types]
Performance graph
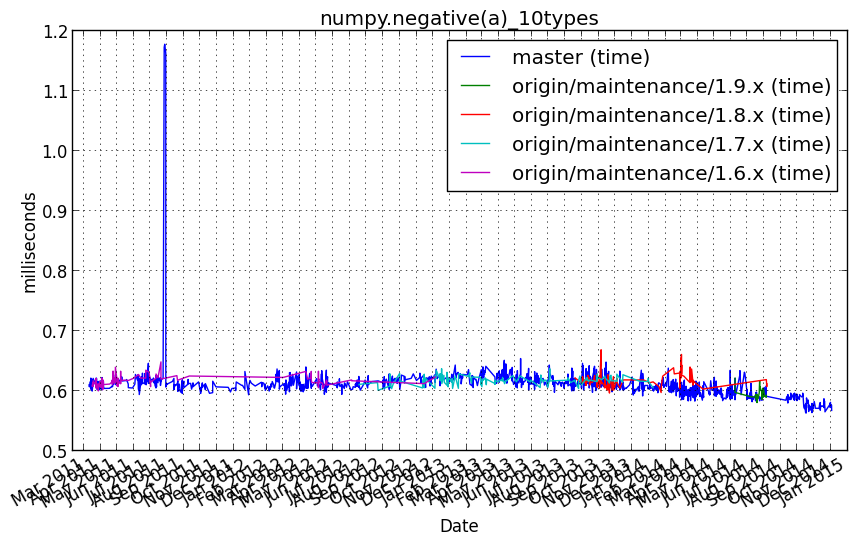
numpy.nextafter(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.nextafter(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
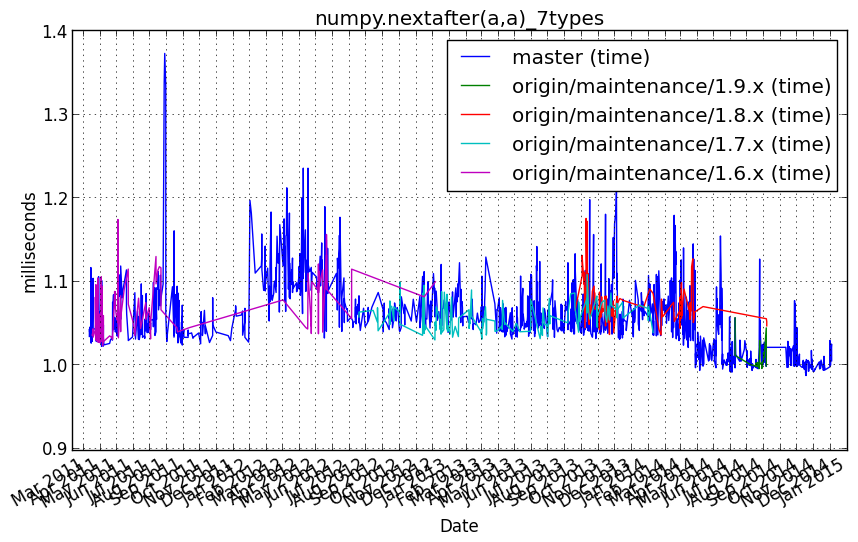
numpy.nonzero¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.bool)
Benchmark statement
numpy.nonzero(d)
Performance graph
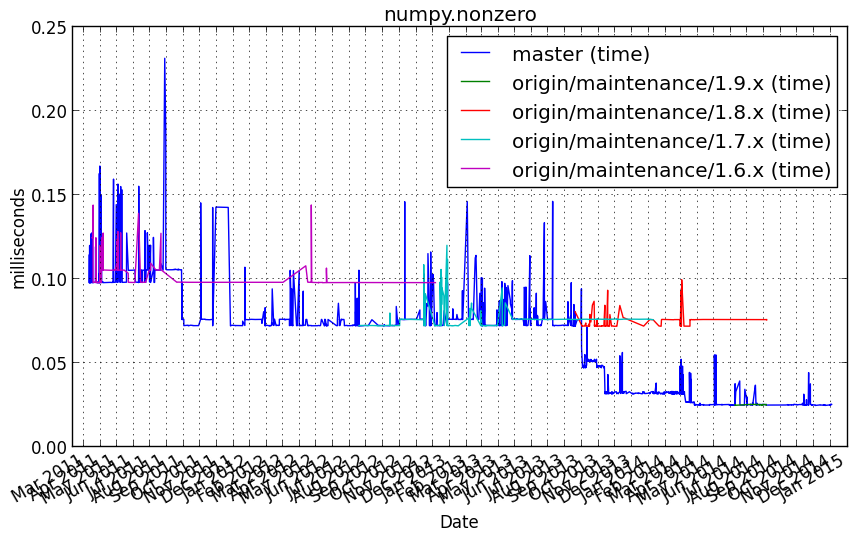
numpy.not_bool¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.bool)
Benchmark statement
~d
Performance graph
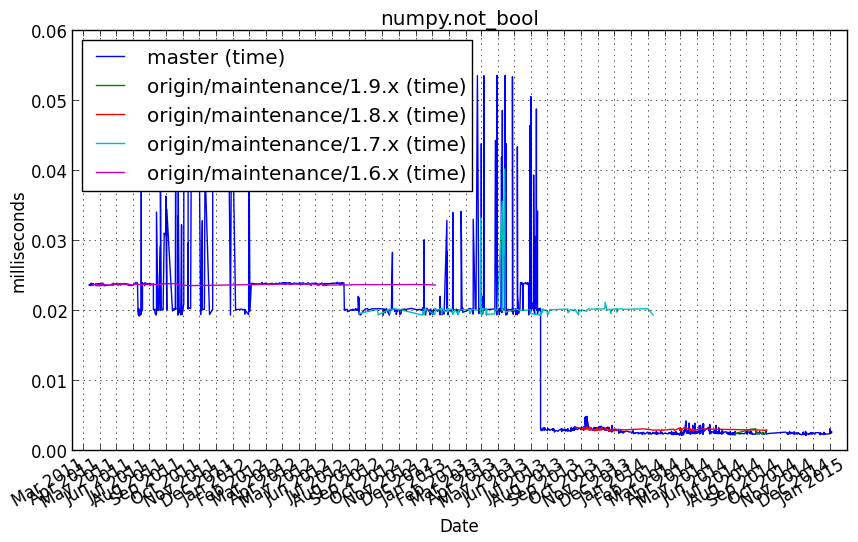
numpy.not_equal(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.not_equal(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
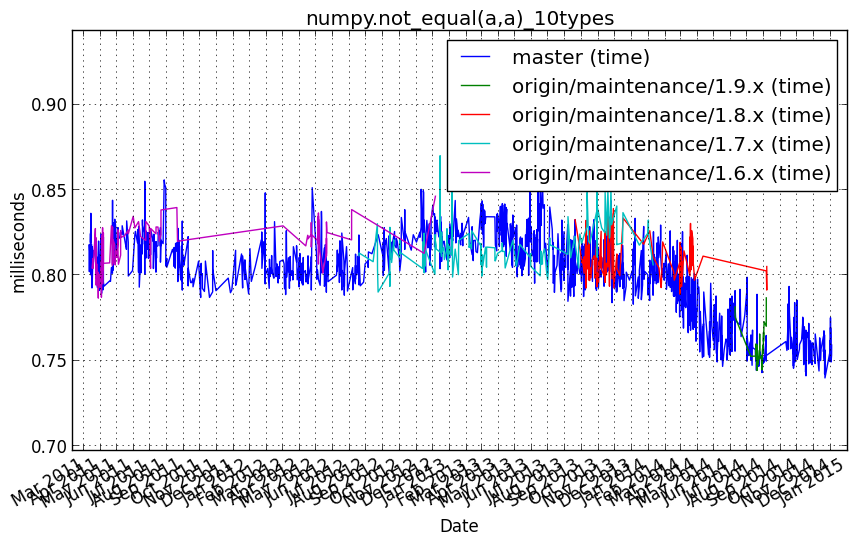
numpy.ones_like(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.ones_like(a) for t, a in squares_.iteritems() if t in types]
Performance graph
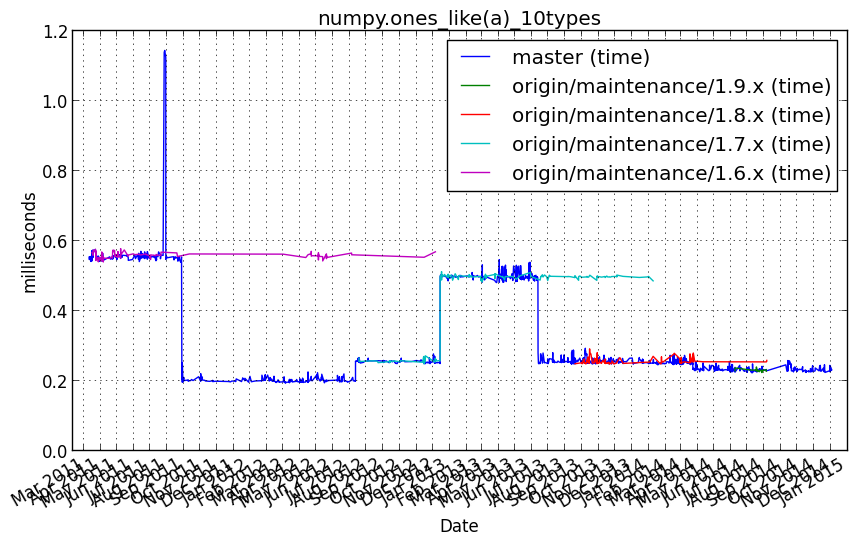
numpy.or_bool¶
Benchmark setup
from numpy_vb_common import *
d = numpy.ones(20000, dtype=numpy.bool)
Benchmark statement
d | d
Performance graph
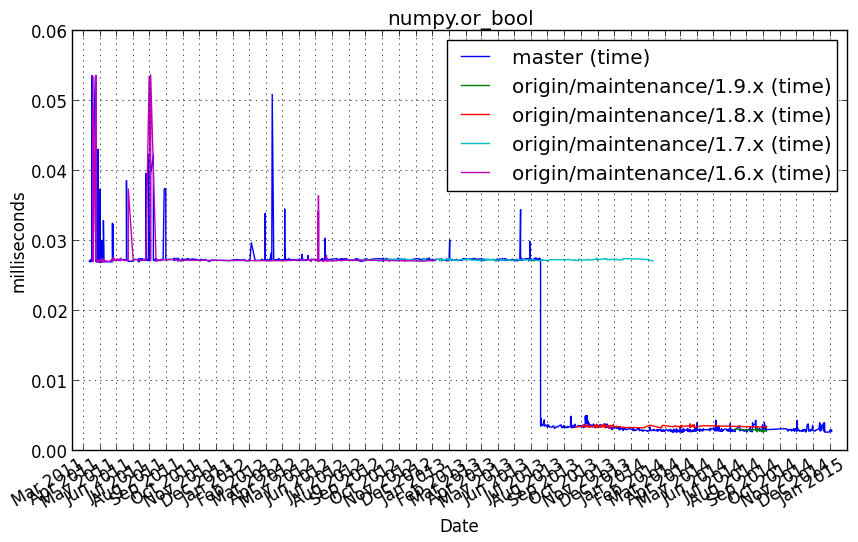
numpy.power(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.power(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
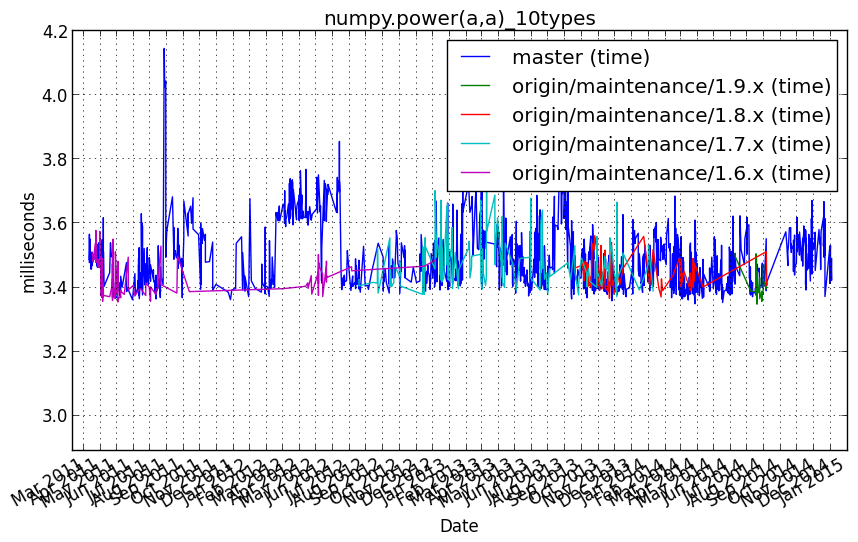
numpy.rad2deg(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.rad2deg(a) for t, a in squares_.iteritems() if t in types]
Performance graph
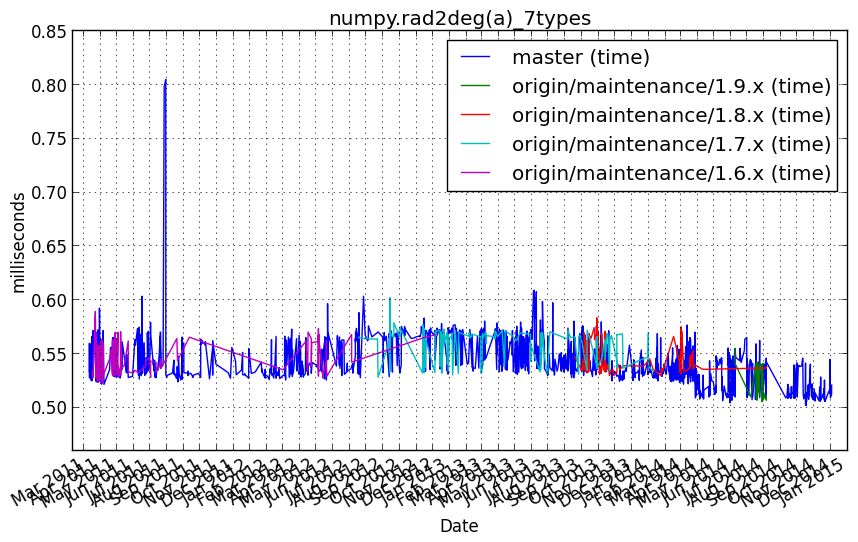
numpy.radians(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.radians(a) for t, a in squares_.iteritems() if t in types]
Performance graph
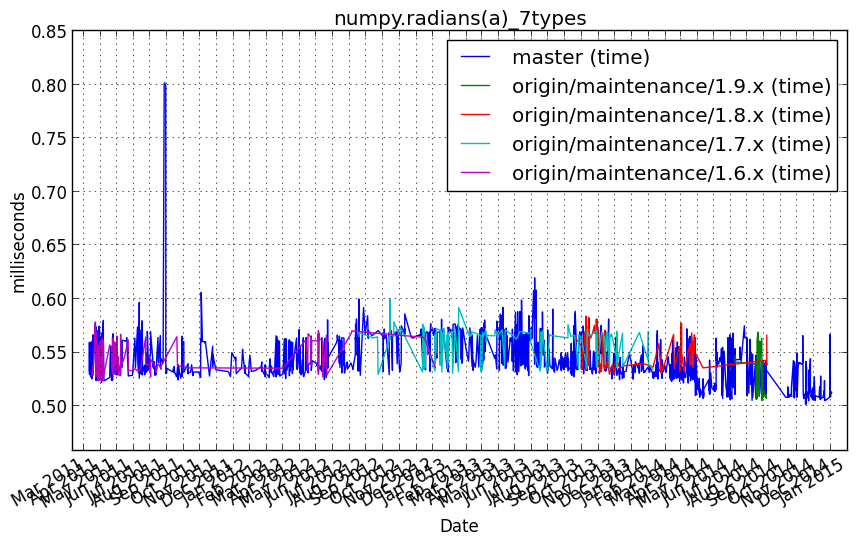
numpy.reciprocal(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.reciprocal(a) for t, a in squares_.iteritems() if t in types]
Performance graph
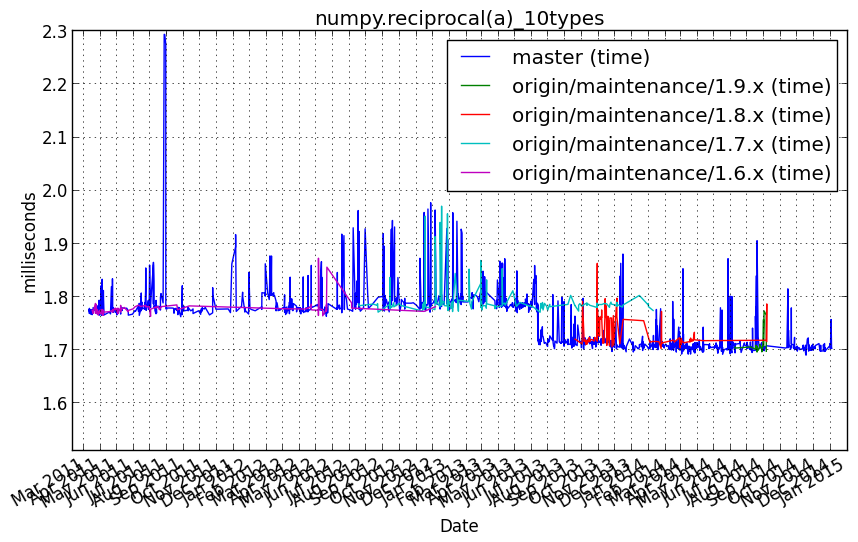
numpy.remainder(a,a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.remainder(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
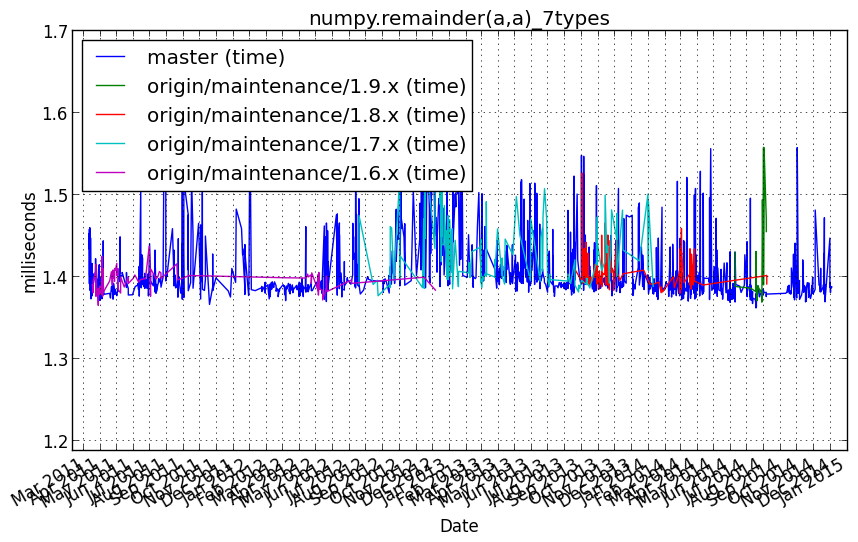
numpy.right_shift(a,a)_3types¶
Benchmark setup
from numpy_vb_common import *
types=['int32', 'int16', 'int64']
Benchmark statement
[numpy.right_shift(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
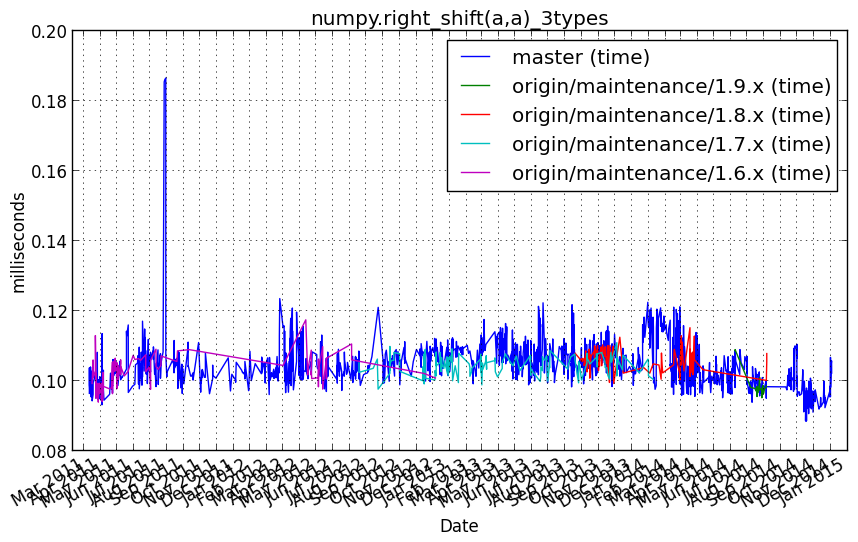
numpy.rint(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.rint(a) for t, a in squares_.iteritems() if t in types]
Performance graph
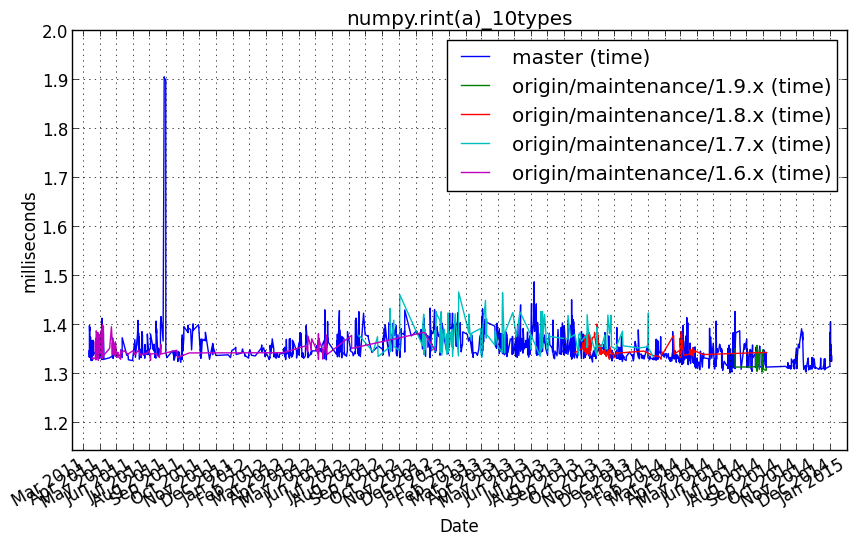
numpy.sign(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.sign(a) for t, a in squares_.iteritems() if t in types]
Performance graph
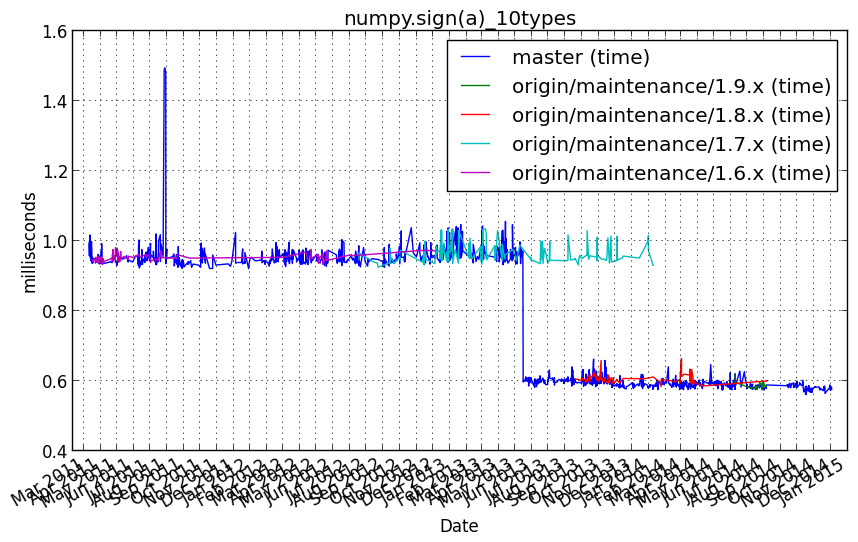
numpy.signbit(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.signbit(a) for t, a in squares_.iteritems() if t in types]
Performance graph
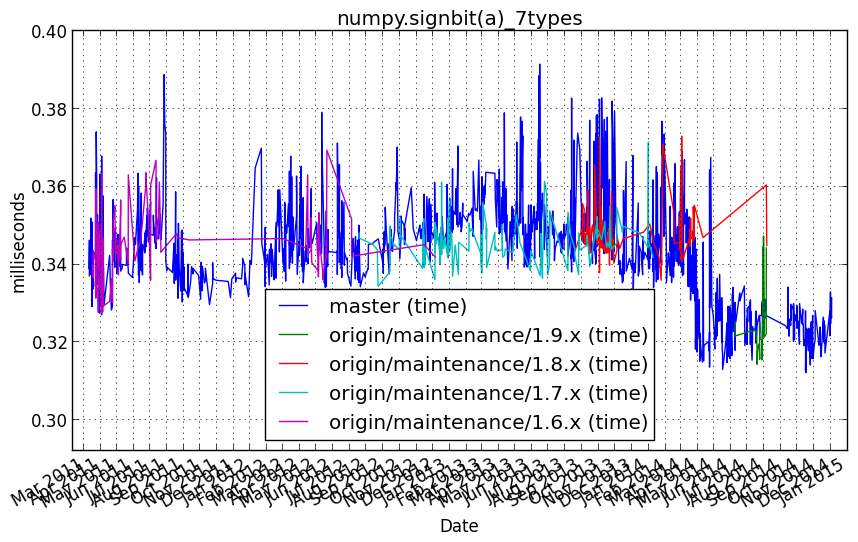
numpy.sin(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.sin(a) for t, a in squares_.iteritems() if t in types]
Performance graph
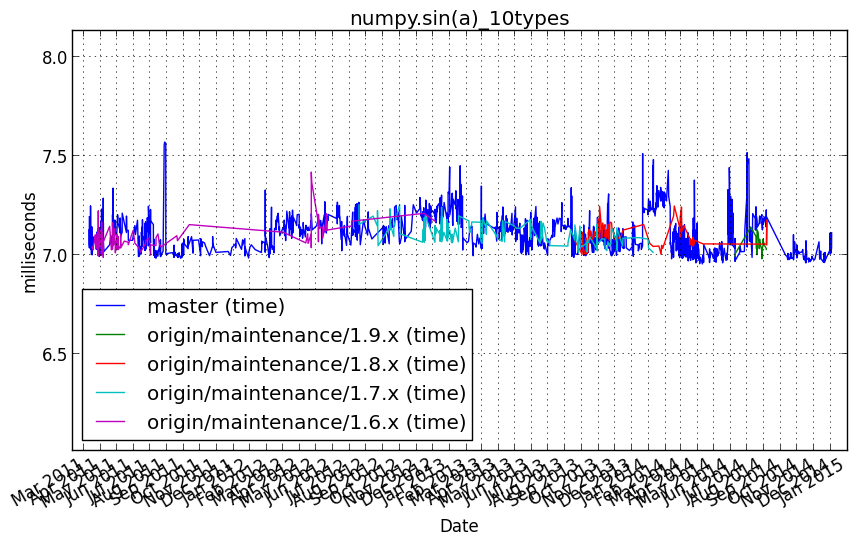
numpy.sinh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.sinh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
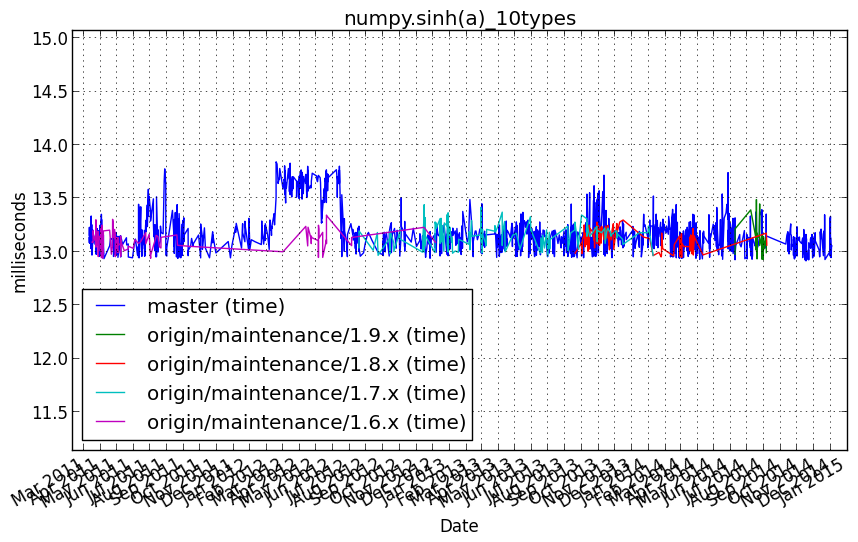
numpy.spacing(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.spacing(a) for t, a in squares_.iteritems() if t in types]
Performance graph
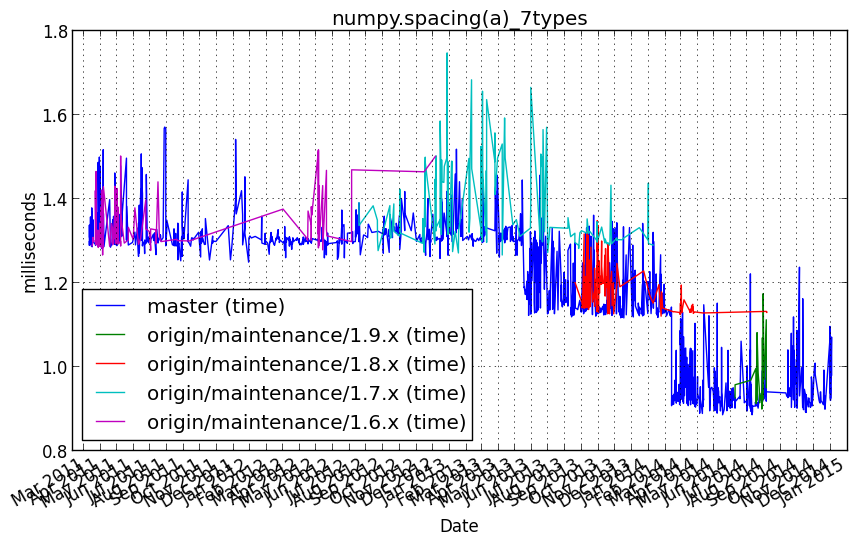
numpy.sqrt(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.sqrt(a) for t, a in squares_.iteritems() if t in types]
Performance graph
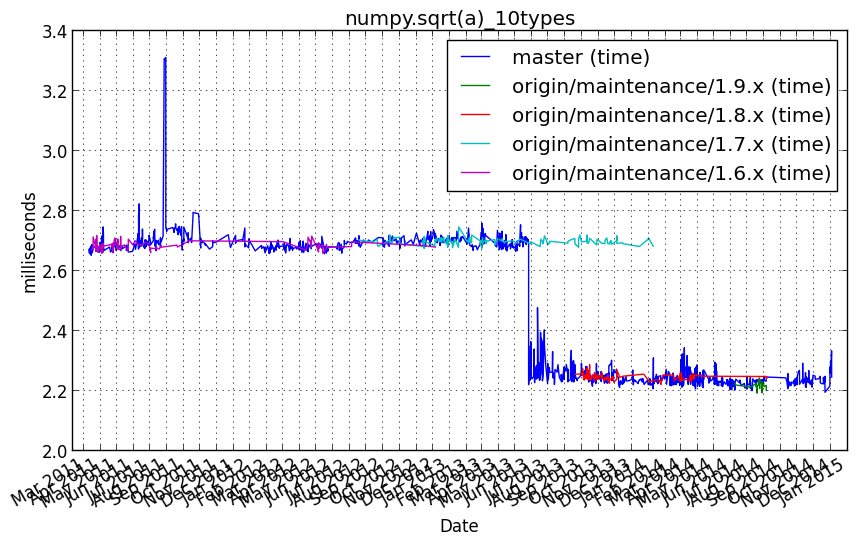
numpy.square(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.square(a) for t, a in squares_.iteritems() if t in types]
Performance graph
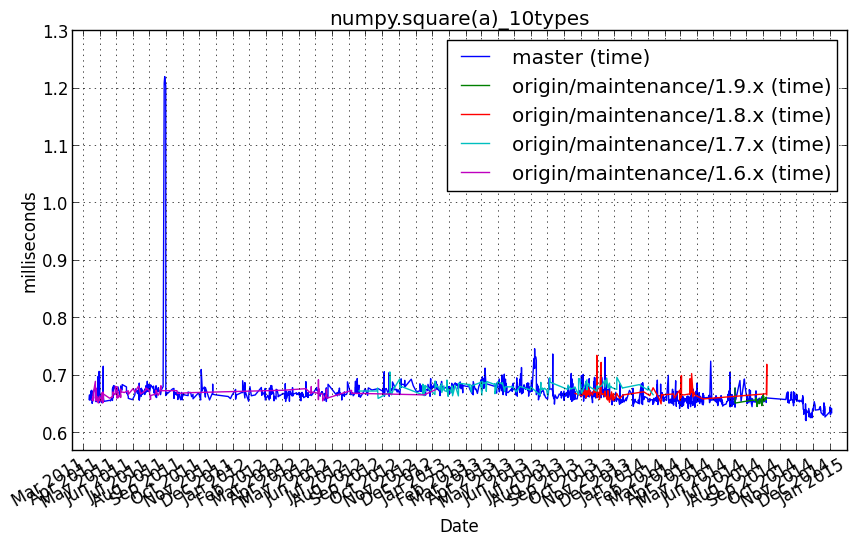
numpy.subtract(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.subtract(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
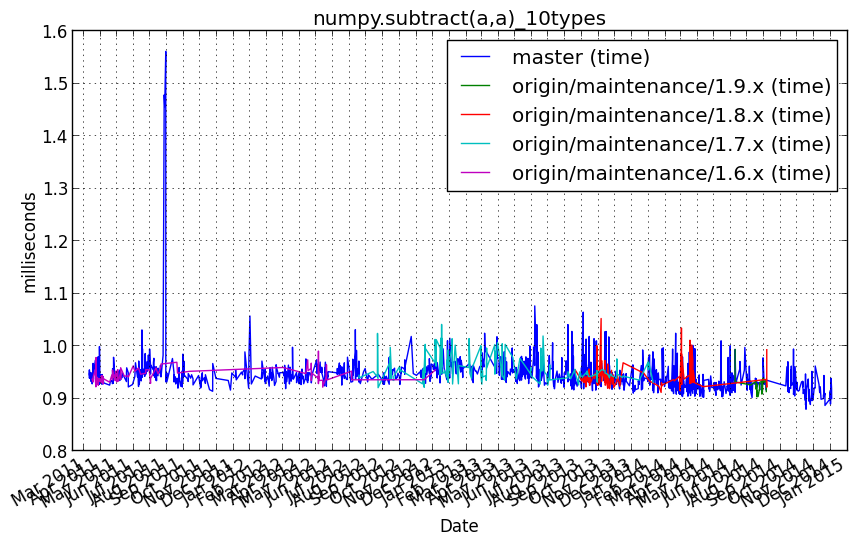
numpy.tan(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.tan(a) for t, a in squares_.iteritems() if t in types]
Performance graph
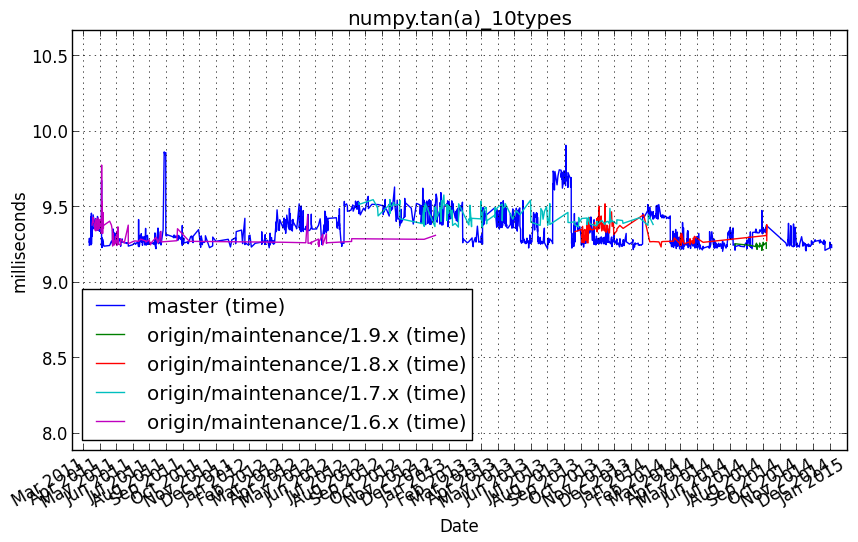
numpy.tanh(a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.tanh(a) for t, a in squares_.iteritems() if t in types]
Performance graph
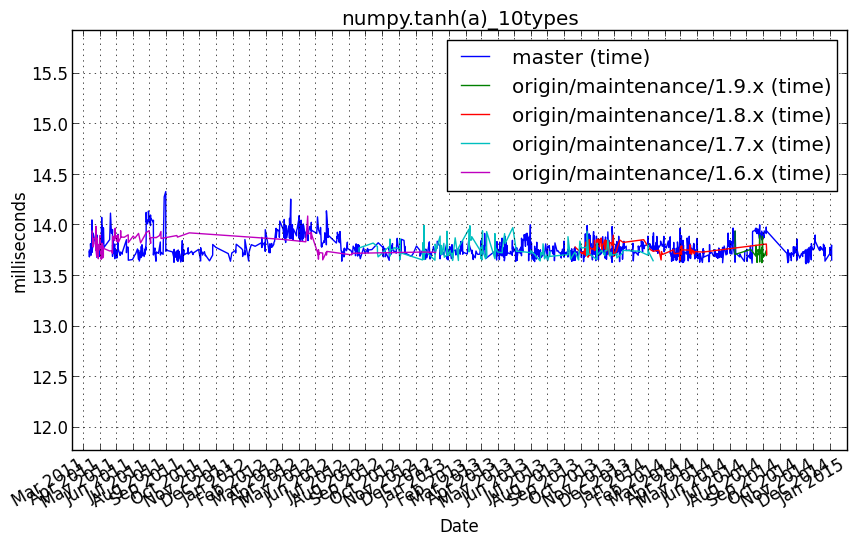
numpy.true_divide(a,a)_10types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'complex256', 'float64', 'complex128', 'complex64', 'int64', 'float32']
Benchmark statement
[numpy.true_divide(a,a) for t, a in squares_.iteritems() if t in types]
Performance graph
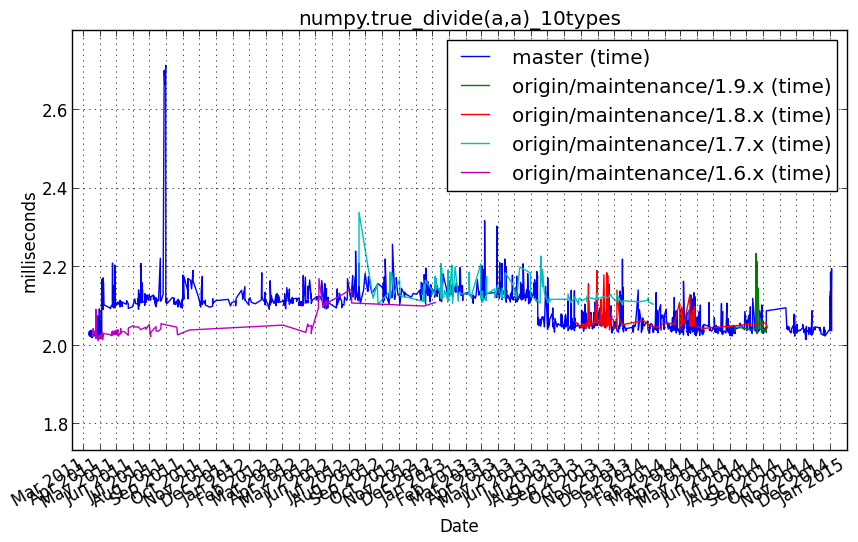
numpy.trunc(a)_7types¶
Benchmark setup
from numpy_vb_common import *
types=['float16', 'int32', 'int16', 'longfloat', 'float64', 'int64', 'float32']
Benchmark statement
[numpy.trunc(a) for t, a in squares_.iteritems() if t in types]
Performance graph
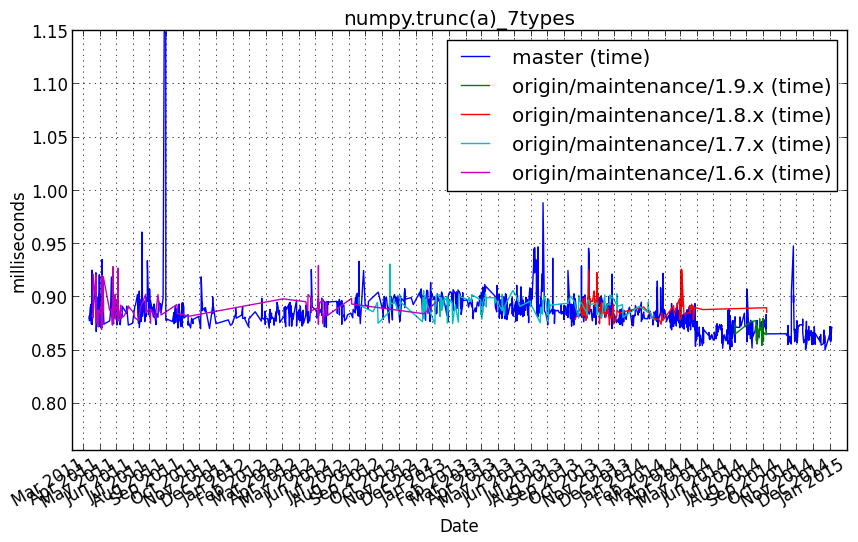