vb_linalg¶
numpy.dot(a, b)¶
Benchmark setup
import numpy
a = numpy.arange(60000.).reshape(150, 400)
b = numpy.arange(240000.).reshape(400, 600)
c = numpy.arange(600)
d = numpy.arange(400)
Benchmark statement
numpy.dot(a, b)
Performance graph
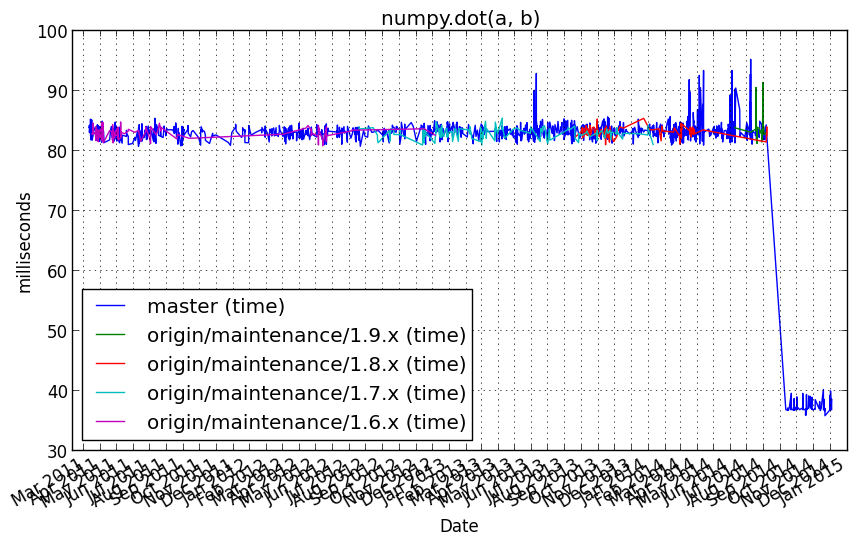
numpy.dot(d, numpy.dot(b, c))¶
Benchmark setup
import numpy
a = numpy.arange(60000.).reshape(150, 400)
b = numpy.arange(240000.).reshape(400, 600)
c = numpy.arange(600)
d = numpy.arange(400)
Benchmark statement
numpy.dot(d, numpy.dot(b, c))
Performance graph
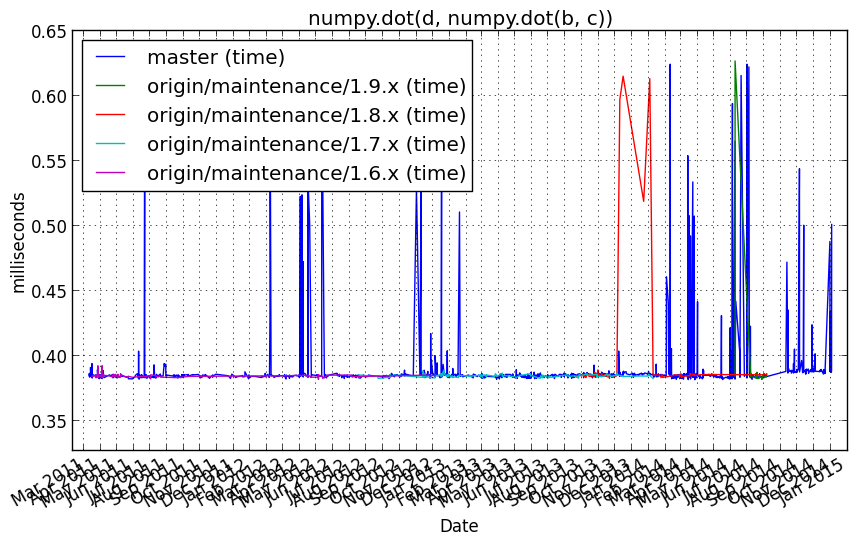
numpy.einsum(‘i,ij,j’, d, b, c)¶
Benchmark setup
import numpy
a = numpy.arange(60000.).reshape(150, 400)
b = numpy.arange(240000.).reshape(400, 600)
c = numpy.arange(600)
d = numpy.arange(400)
Benchmark statement
numpy.einsum('i,ij,j', d, b, c)
Performance graph
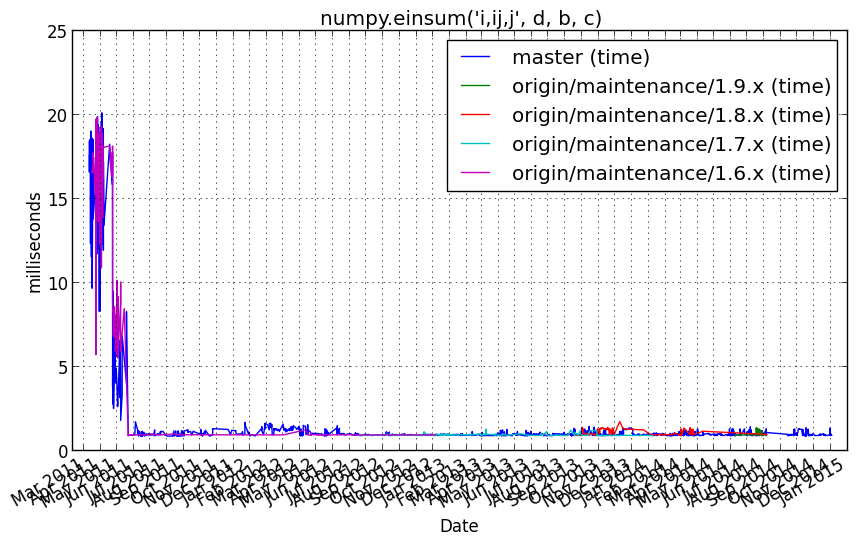
numpy.einsum(‘ij,jk’, a, b)¶
Benchmark setup
import numpy
a = numpy.arange(60000.).reshape(150, 400)
b = numpy.arange(240000.).reshape(400, 600)
c = numpy.arange(600)
d = numpy.arange(400)
Benchmark statement
numpy.einsum('ij,jk', a, b)
Performance graph
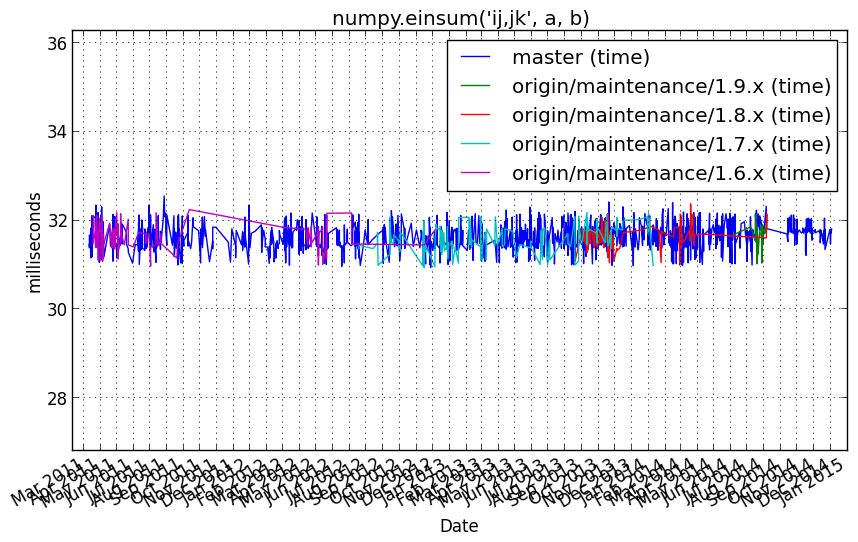
numpy.einsum(‘ijk,jil->kl’, a, b)¶
Benchmark setup
import numpy
a = numpy.arange(480000.).reshape(60, 80, 100)
b = numpy.arange(192000.).reshape(80, 60, 40)
Benchmark statement
numpy.einsum('ijk,jil->kl', a, b)
Performance graph
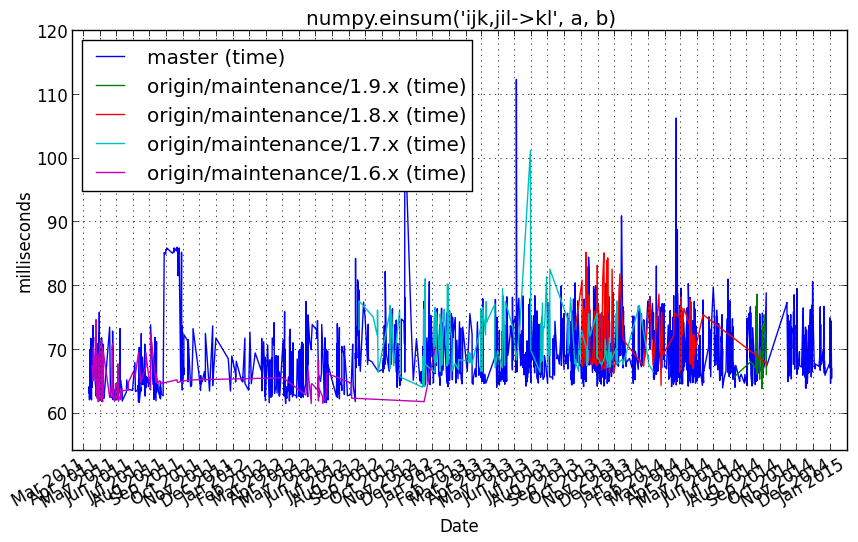
numpy.linalg.det(a)_complex128¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex128']
Benchmark statement
numpy.linalg.det(a)
Performance graph
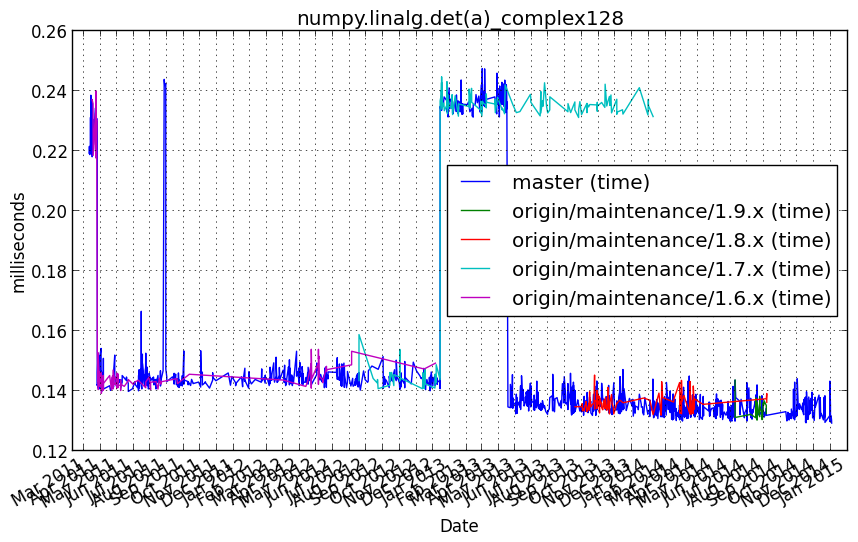
numpy.linalg.det(a)_complex64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex64']
Benchmark statement
numpy.linalg.det(a)
Performance graph
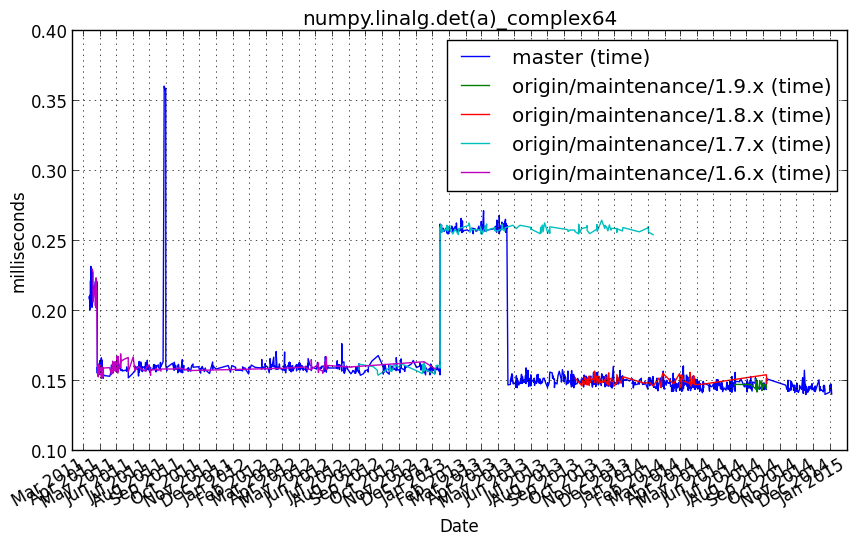
numpy.linalg.det(a)_float32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float32']
Benchmark statement
numpy.linalg.det(a)
Performance graph
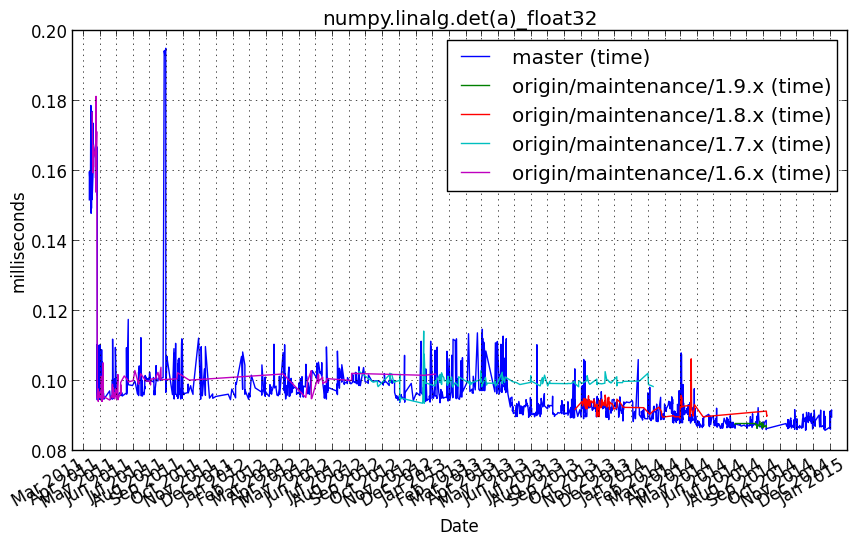
numpy.linalg.det(a)_float64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float64']
Benchmark statement
numpy.linalg.det(a)
Performance graph
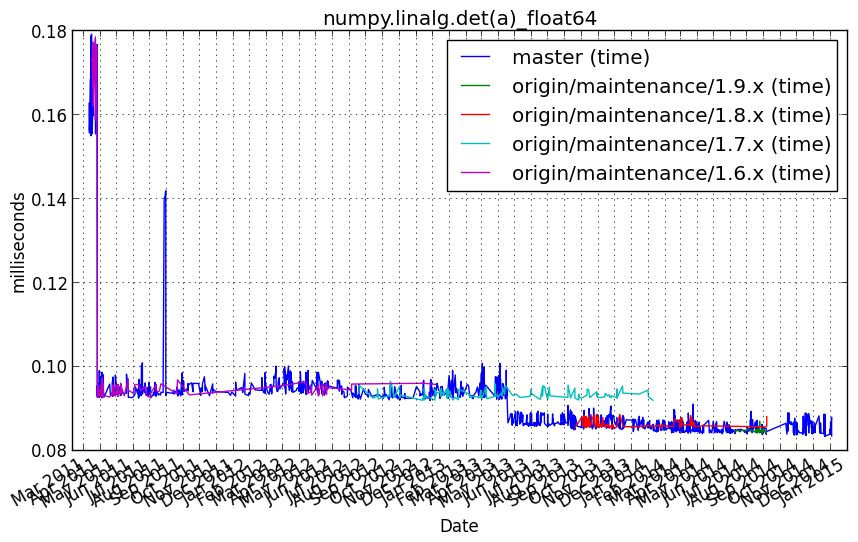
numpy.linalg.det(a)_int16¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int16']
Benchmark statement
numpy.linalg.det(a)
Performance graph
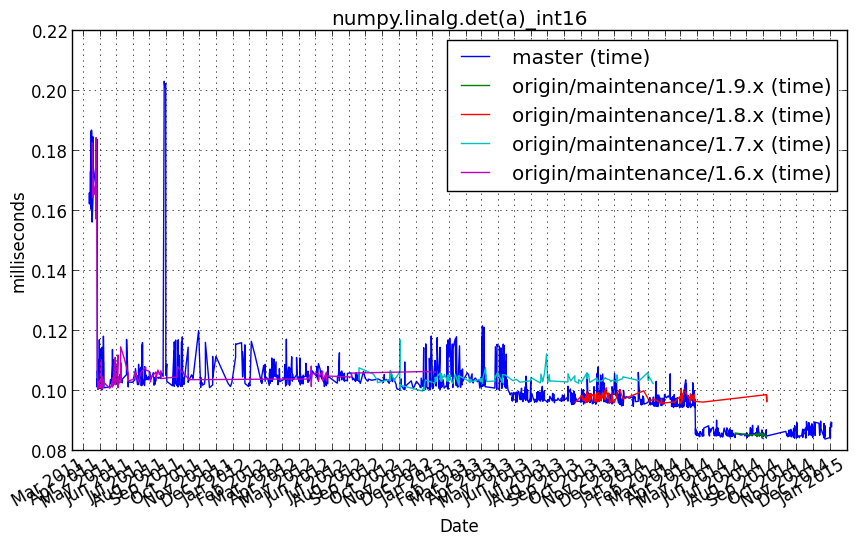
numpy.linalg.det(a)_int32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int32']
Benchmark statement
numpy.linalg.det(a)
Performance graph
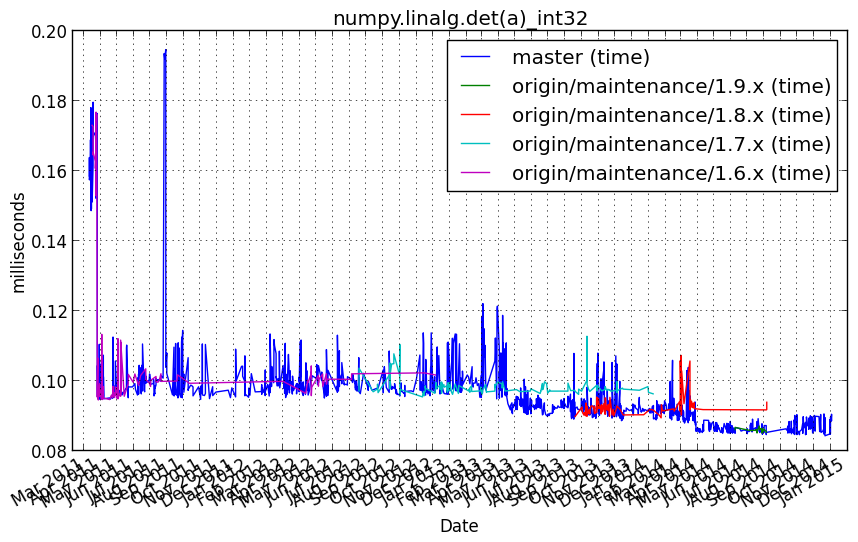
numpy.linalg.det(a)_int64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int64']
Benchmark statement
numpy.linalg.det(a)
Performance graph
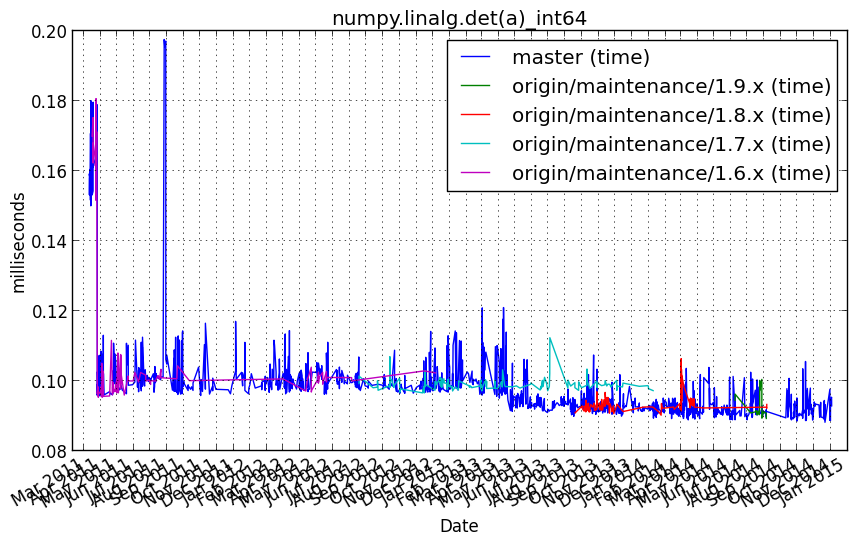
numpy.linalg.lstsq(a, b)_float64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float64']; b=indexes_rand[:100].astype(numpy.float64)
Benchmark statement
numpy.linalg.lstsq(a, b)
Performance graph
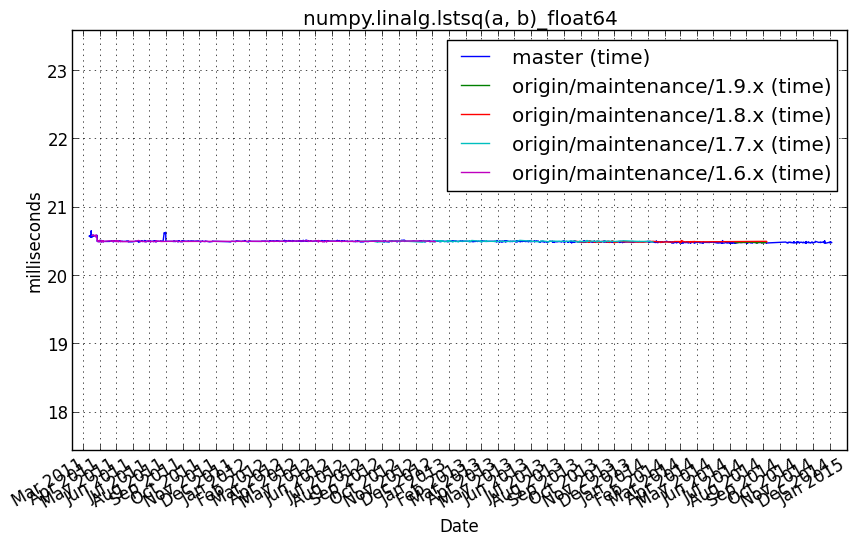
numpy.linalg.norm(a)_complex128¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex128']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
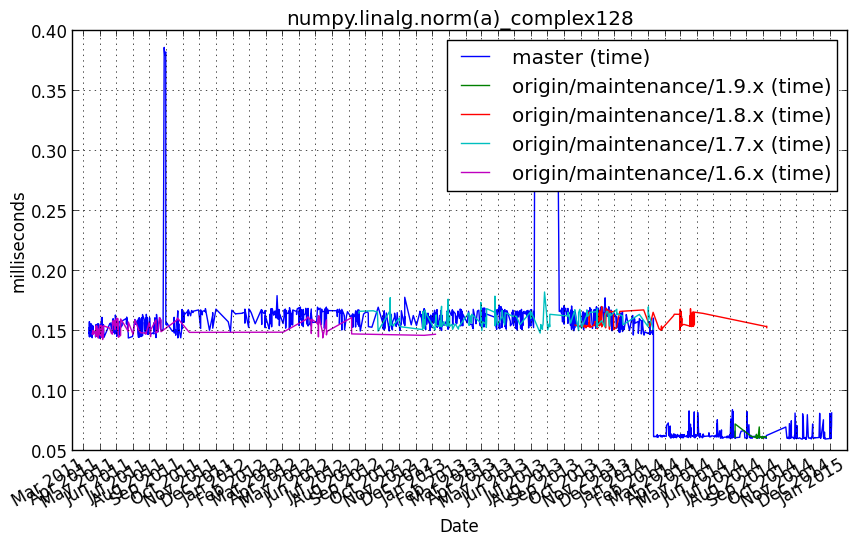
numpy.linalg.norm(a)_complex256¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex256']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
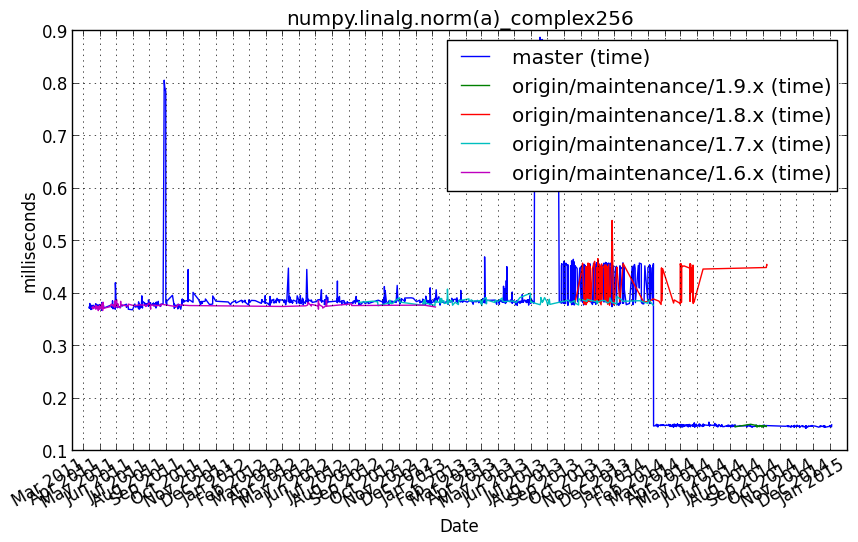
numpy.linalg.norm(a)_complex64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex64']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
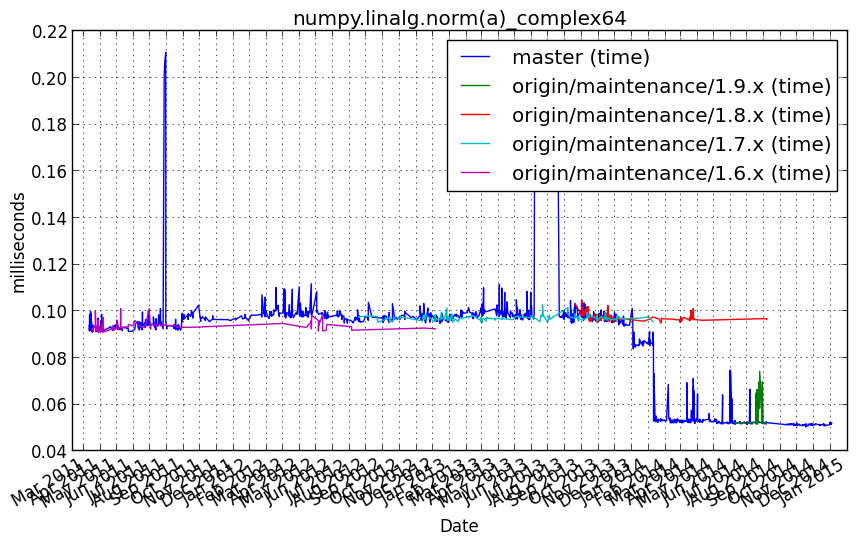
numpy.linalg.norm(a)_float16¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float16']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
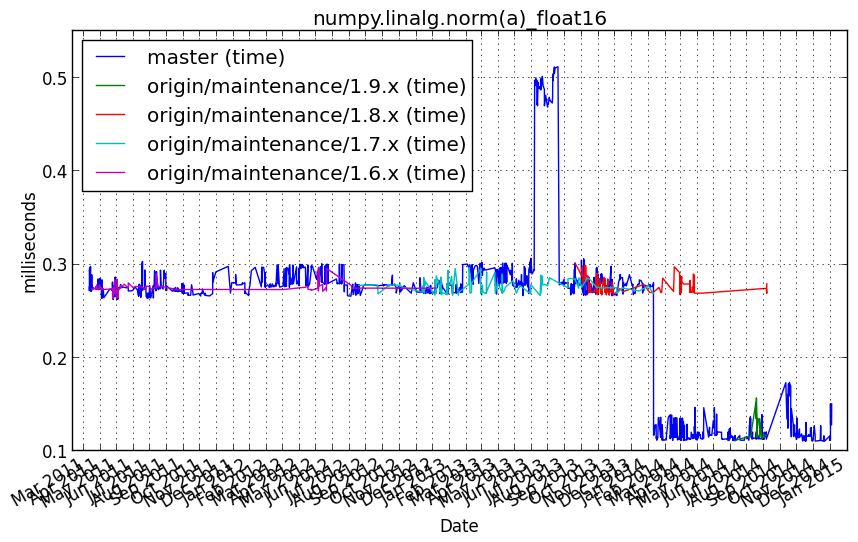
numpy.linalg.norm(a)_float32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float32']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
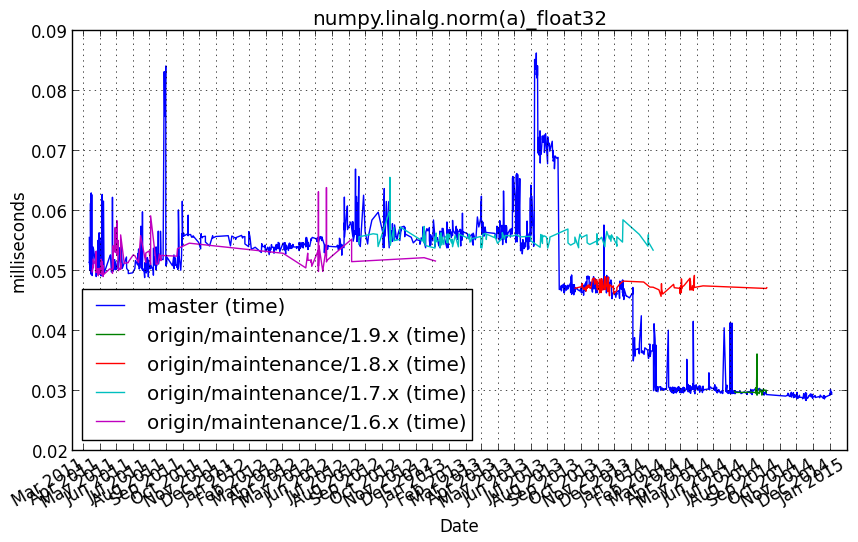
numpy.linalg.norm(a)_float64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float64']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
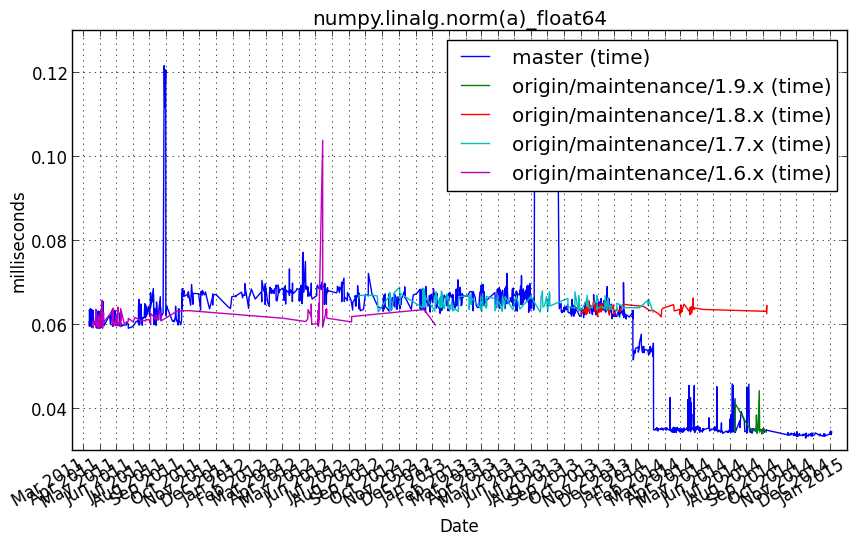
numpy.linalg.norm(a)_int16¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int16']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
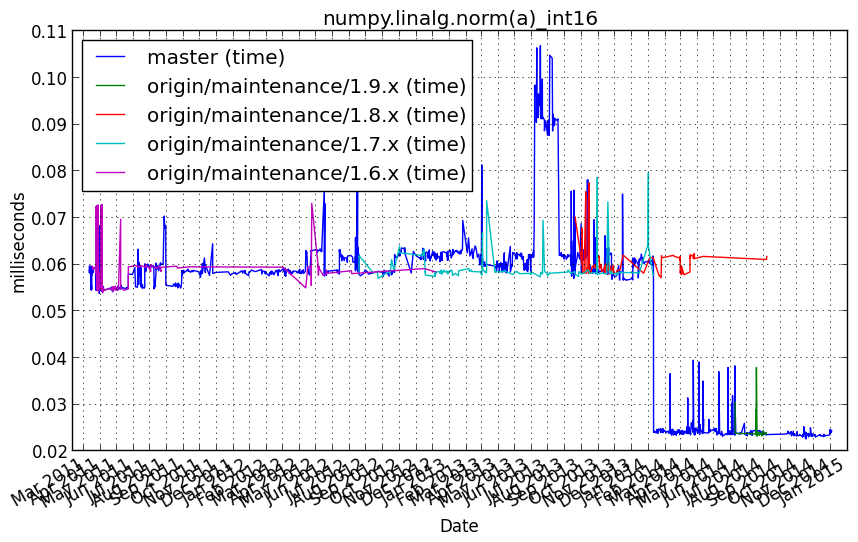
numpy.linalg.norm(a)_int32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int32']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
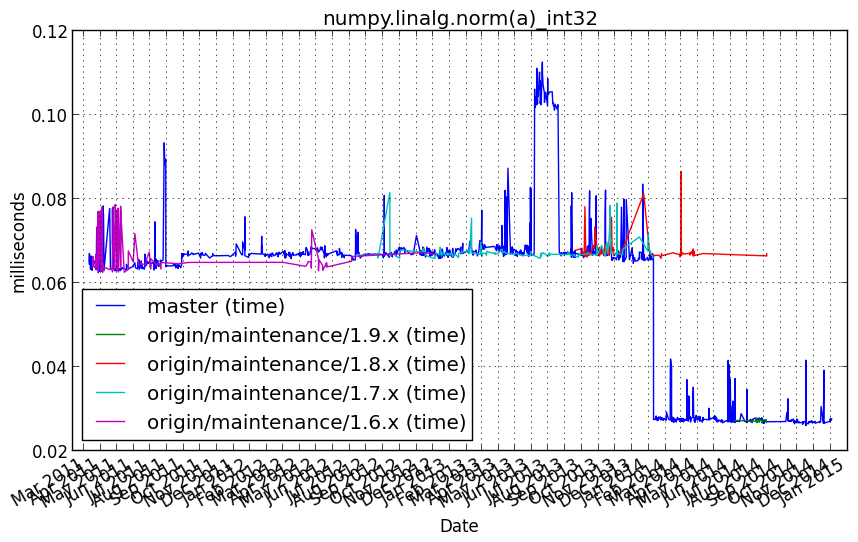
numpy.linalg.norm(a)_int64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int64']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
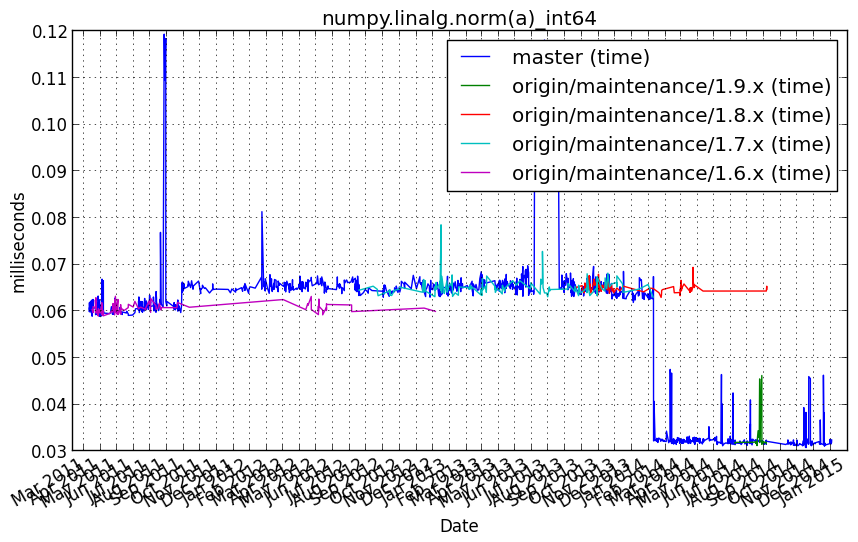
numpy.linalg.norm(a)_longfloat¶
Benchmark setup
from numpy_vb_common import *
a=squares_['longfloat']
Benchmark statement
numpy.linalg.norm(a)
Performance graph
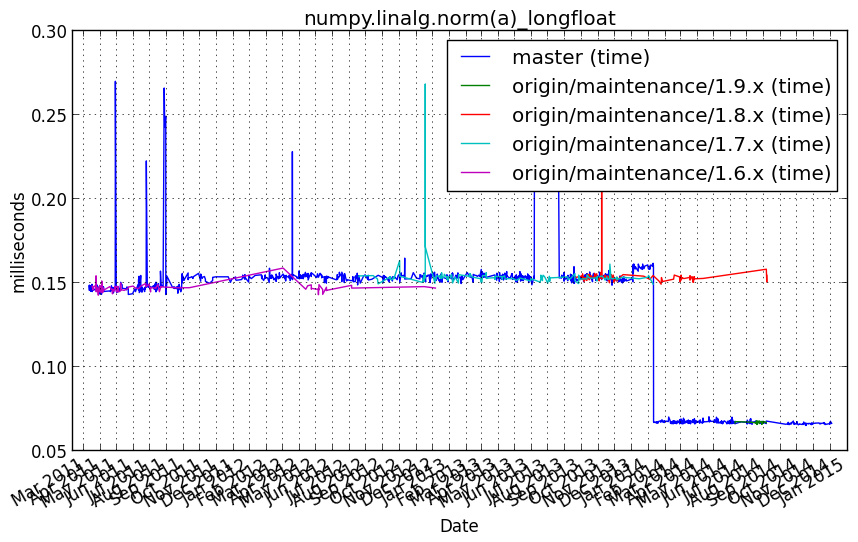
numpy.linalg.pinv(a)_complex128¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex128']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
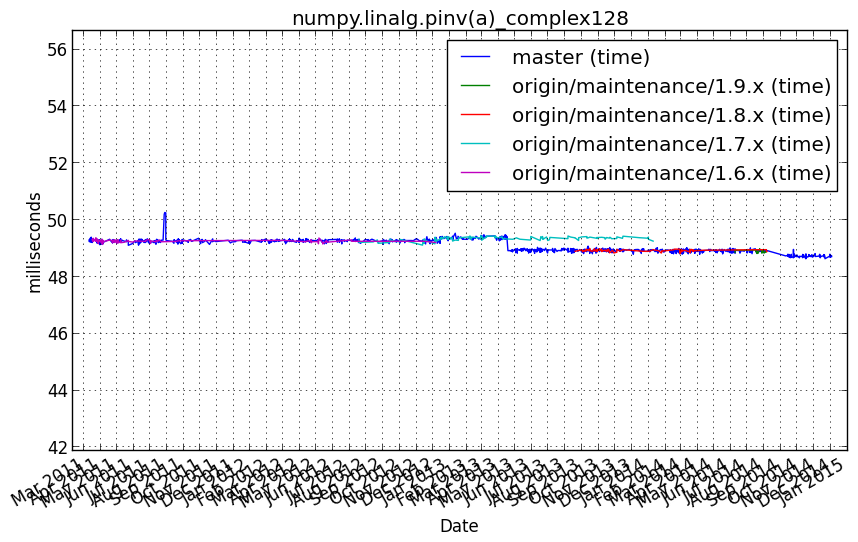
numpy.linalg.pinv(a)_complex64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex64']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
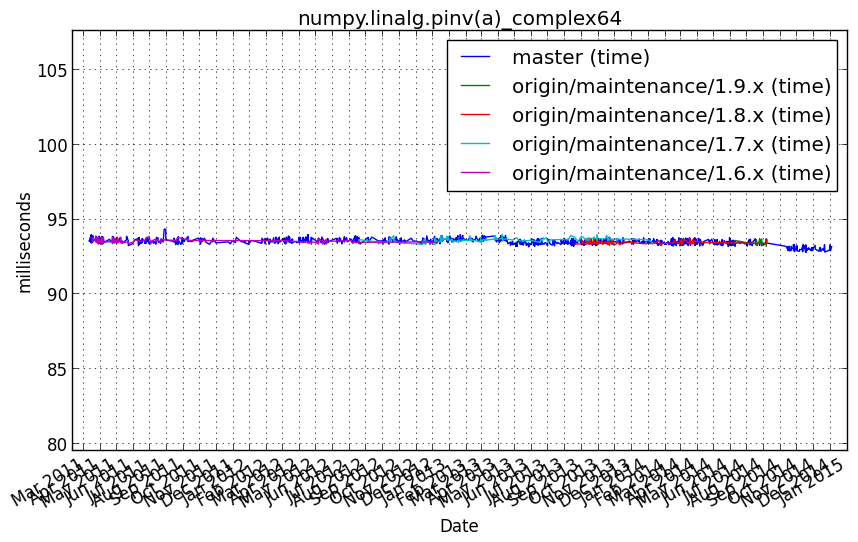
numpy.linalg.pinv(a)_float32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float32']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
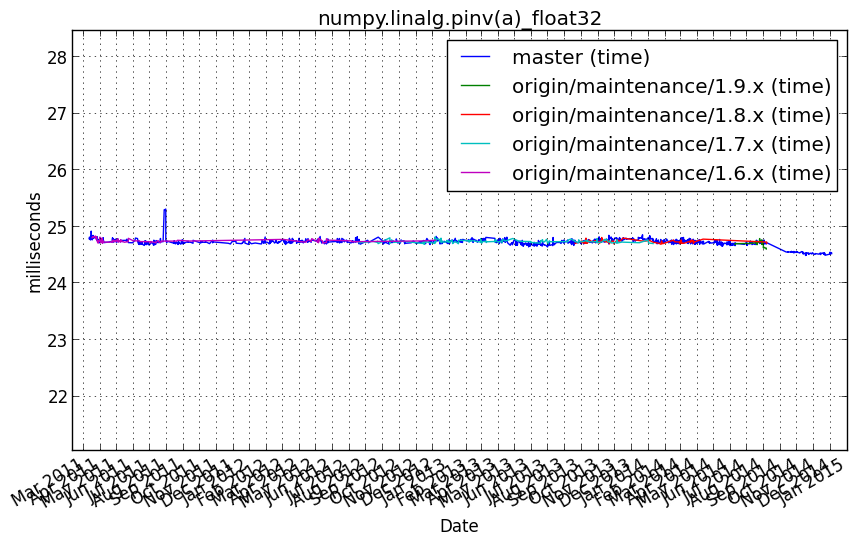
numpy.linalg.pinv(a)_float64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float64']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
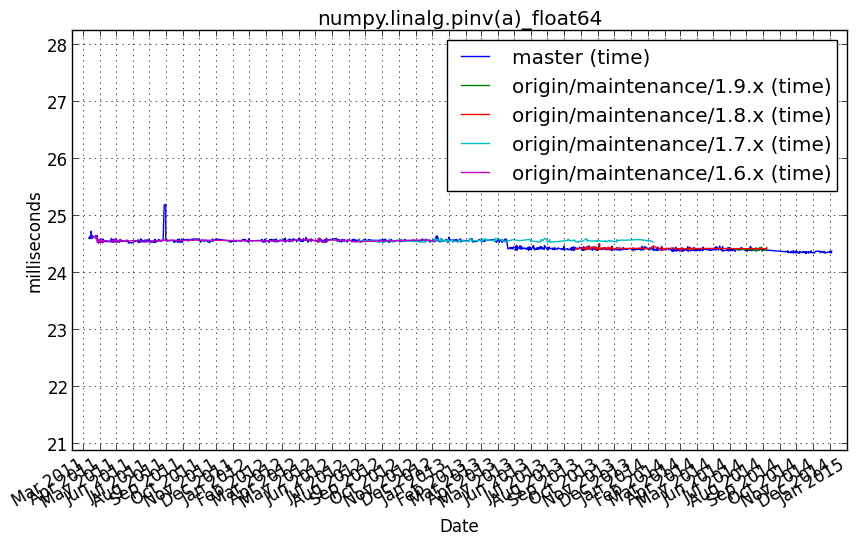
numpy.linalg.pinv(a)_int16¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int16']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
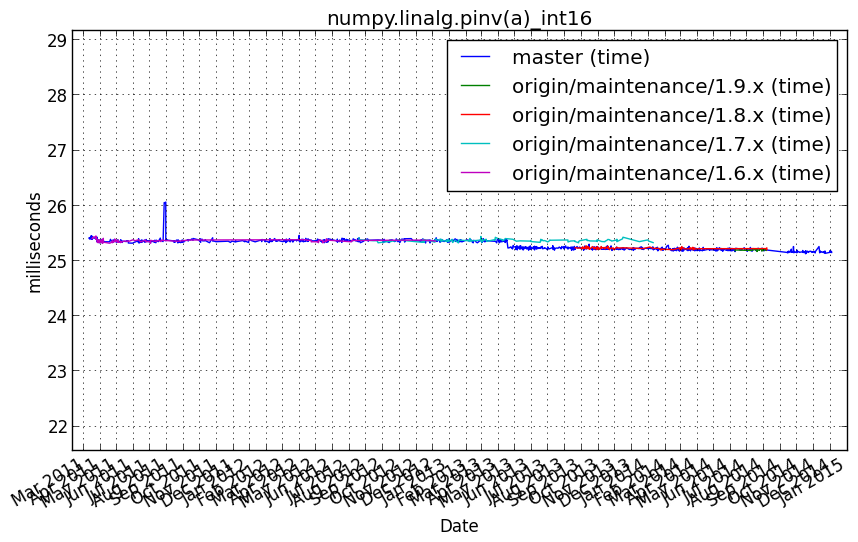
numpy.linalg.pinv(a)_int32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int32']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
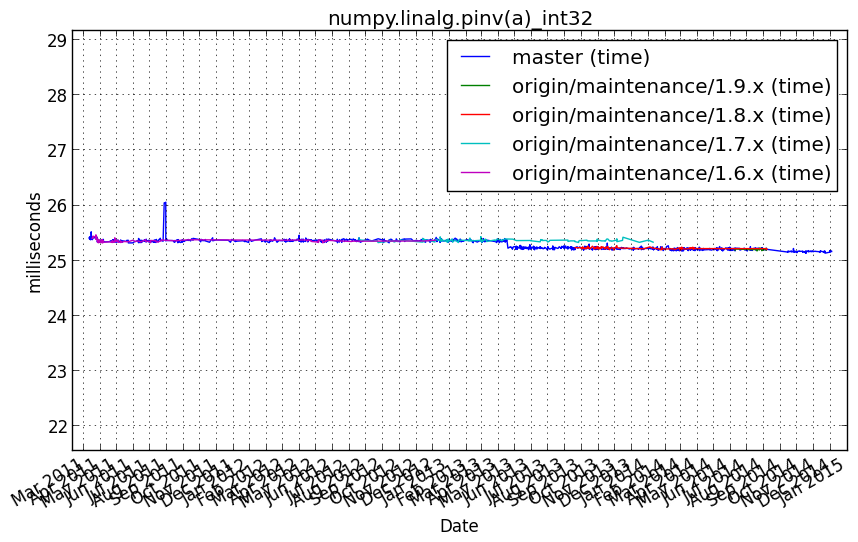
numpy.linalg.pinv(a)_int64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int64']
Benchmark statement
numpy.linalg.pinv(a)
Performance graph
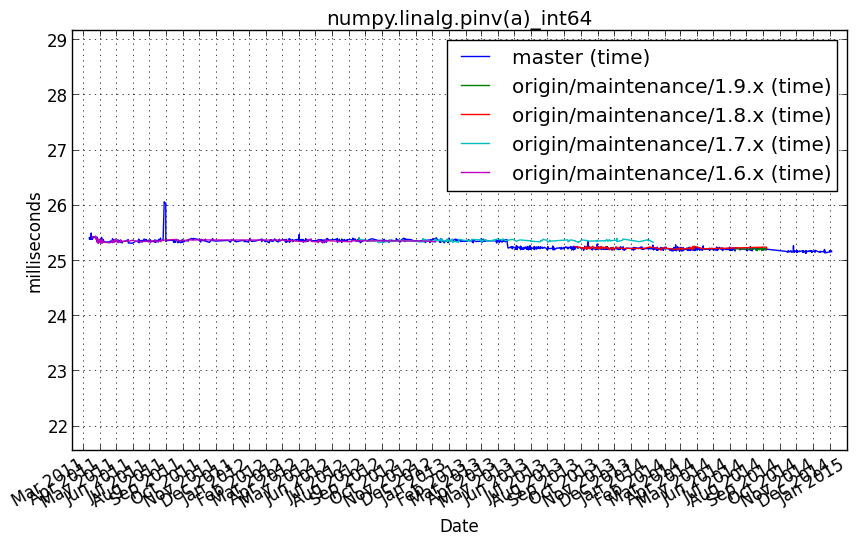
numpy.linalg.svd(a)_complex128¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex128']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
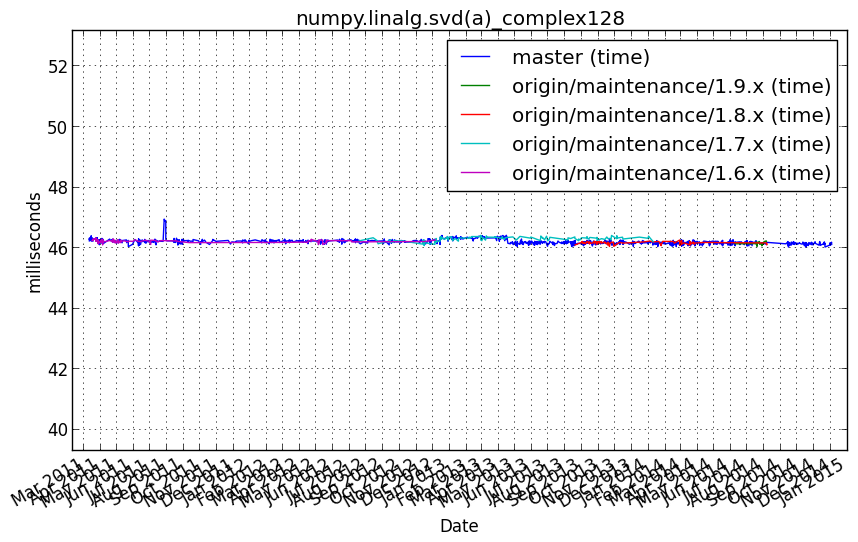
numpy.linalg.svd(a)_complex64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['complex64']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
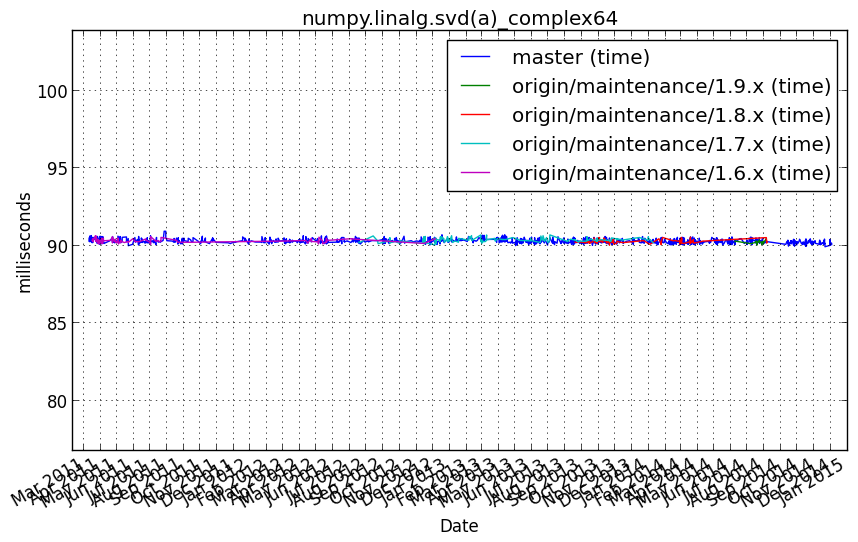
numpy.linalg.svd(a)_float32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float32']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
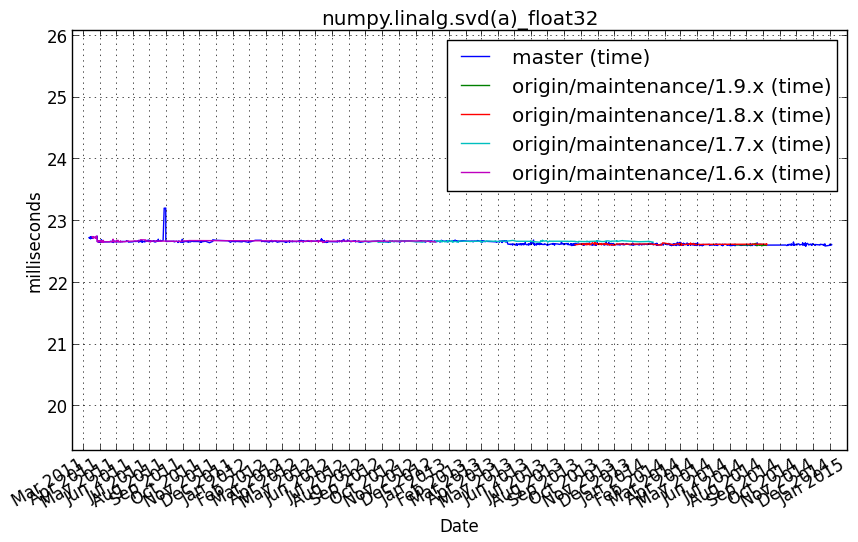
numpy.linalg.svd(a)_float64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['float64']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
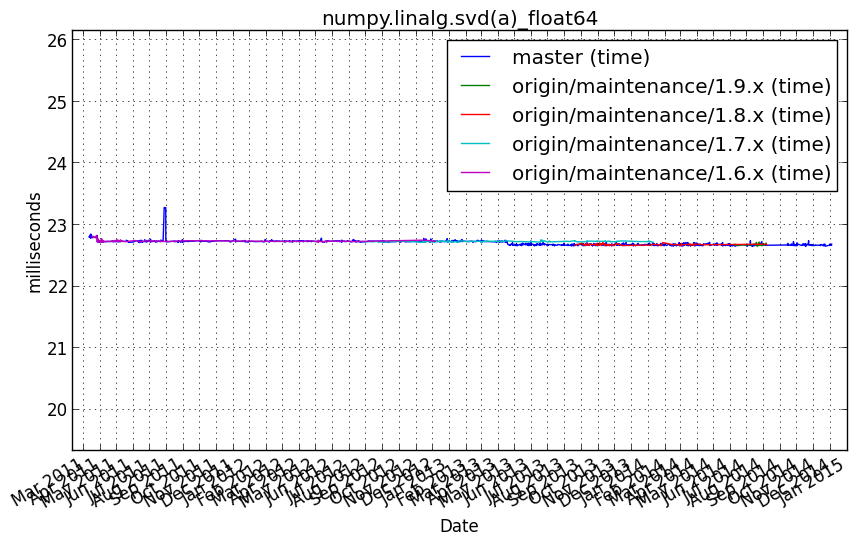
numpy.linalg.svd(a)_int16¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int16']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
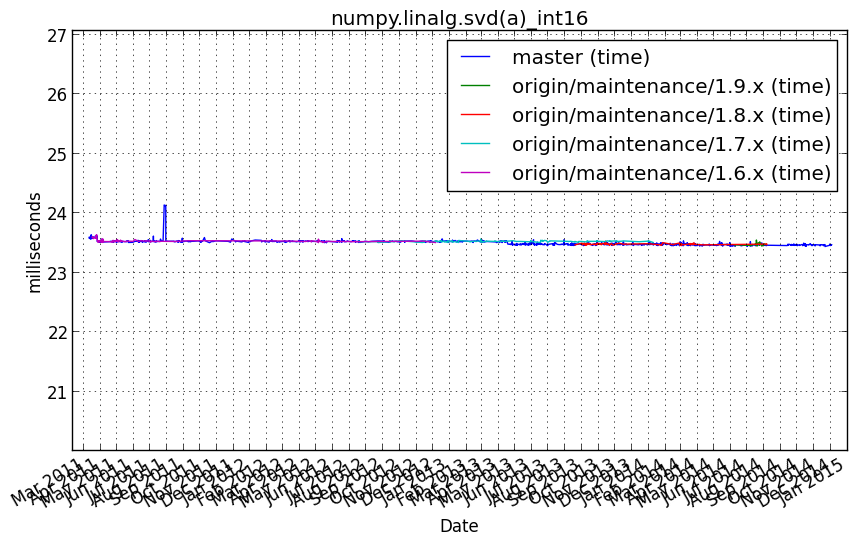
numpy.linalg.svd(a)_int32¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int32']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
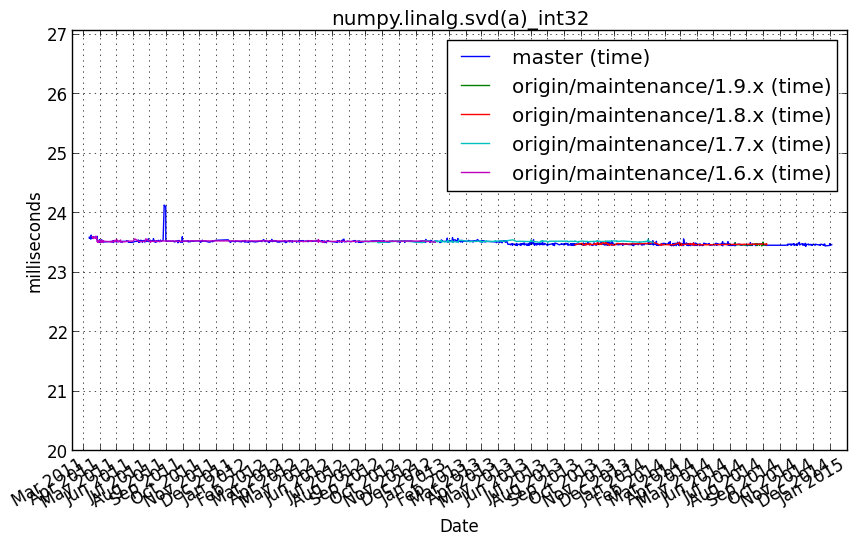
numpy.linalg.svd(a)_int64¶
Benchmark setup
from numpy_vb_common import *
a=squares_['int64']
Benchmark statement
numpy.linalg.svd(a)
Performance graph
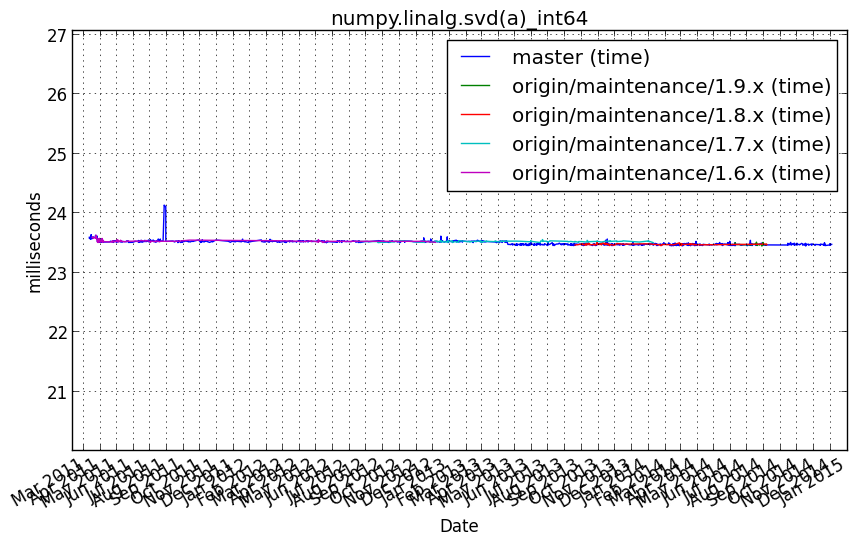
numpy.tensordot(a, b, axes=([1,0], [0,1]))¶
Benchmark setup
import numpy
a = numpy.arange(480000.).reshape(60, 80, 100)
b = numpy.arange(192000.).reshape(80, 60, 40)
Benchmark statement
numpy.tensordot(a, b, axes=([1,0], [0,1]))
Performance graph
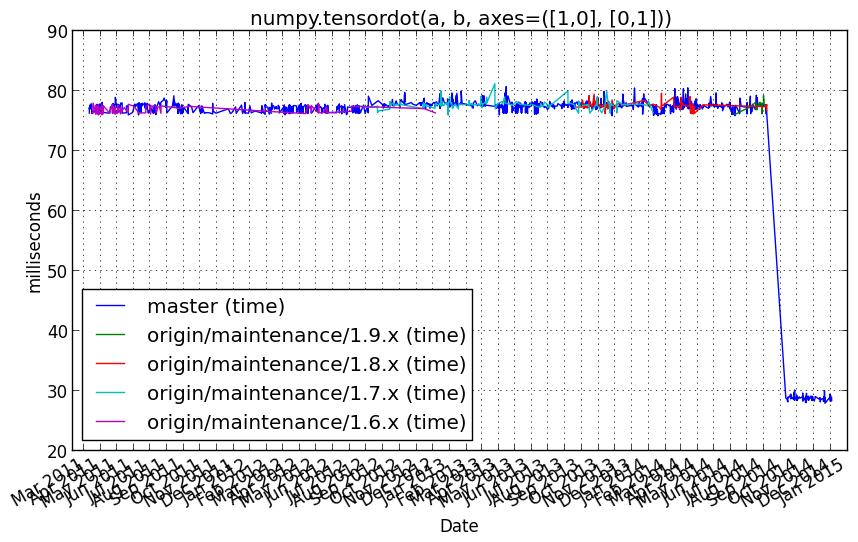