vb_indexing¶
a[:,indexes_]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[:,indexes_]
Performance graph
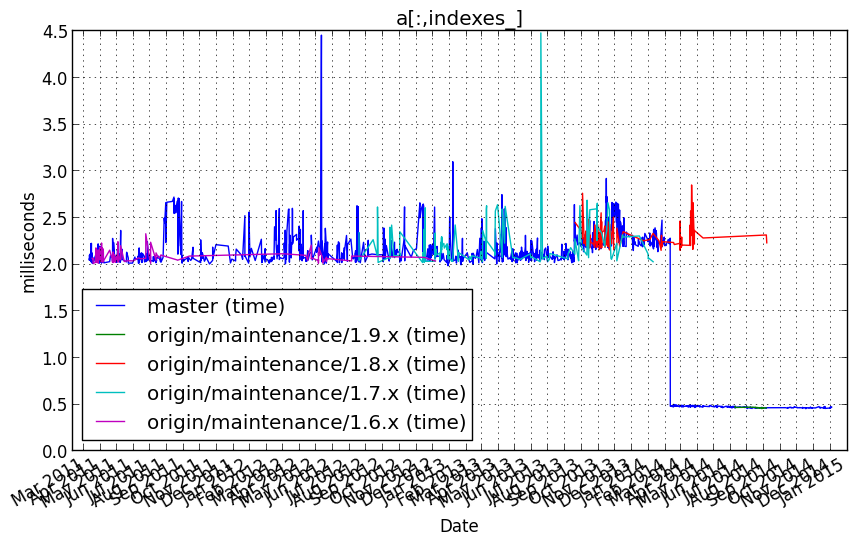
a[:,indexes_]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[:,indexes_]=1
Performance graph
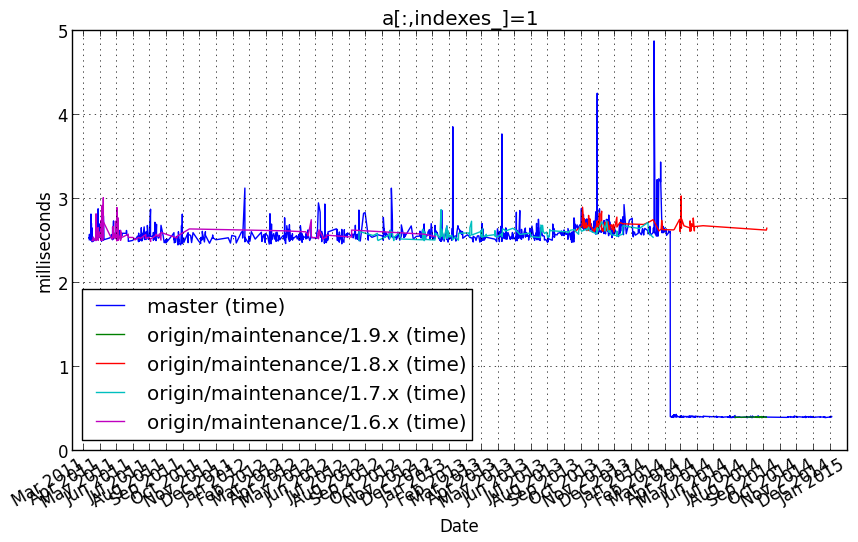
a[:,indexes_rand_]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[:,indexes_rand_]
Performance graph
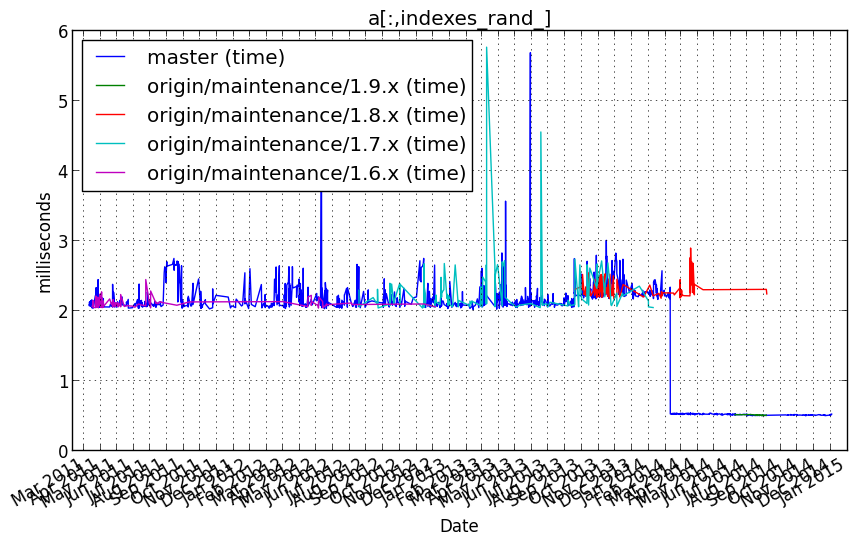
a[:,indexes_rand_]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[:,indexes_rand_]=1
Performance graph
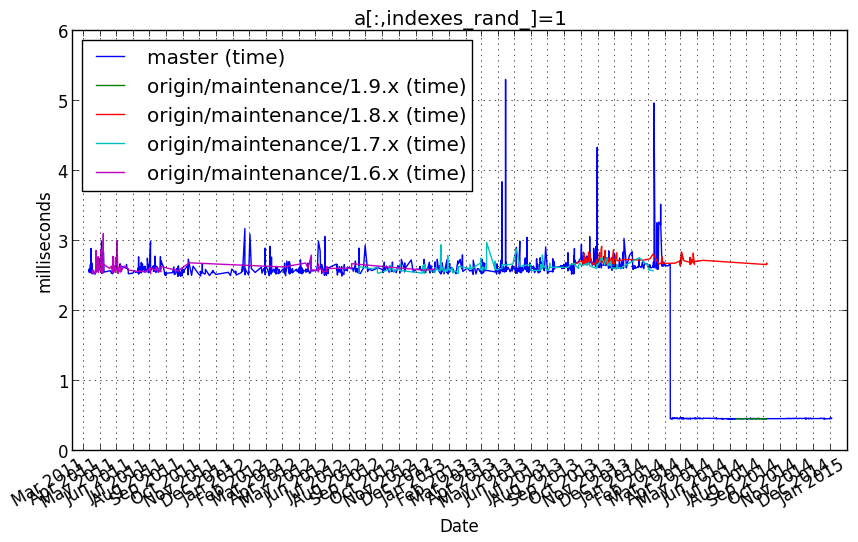
a[indexes_]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[indexes_]
Performance graph
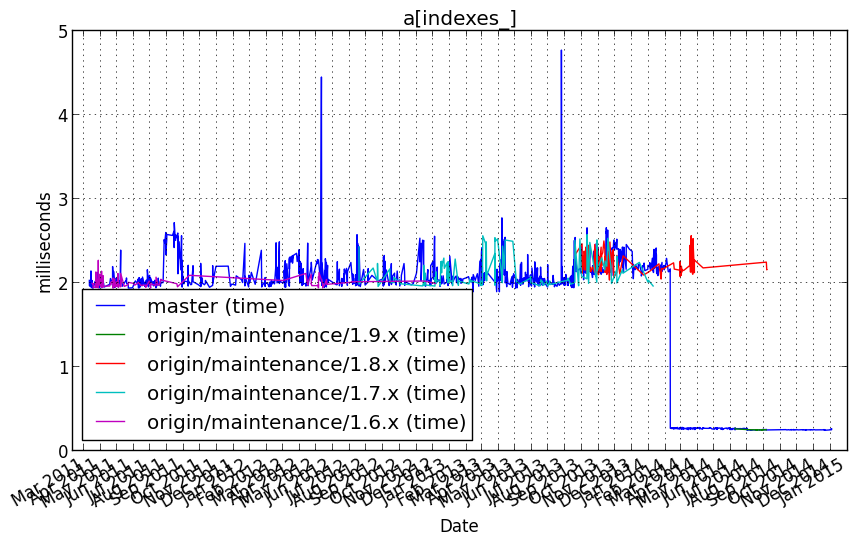
a[indexes_]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[indexes_]=1
Performance graph
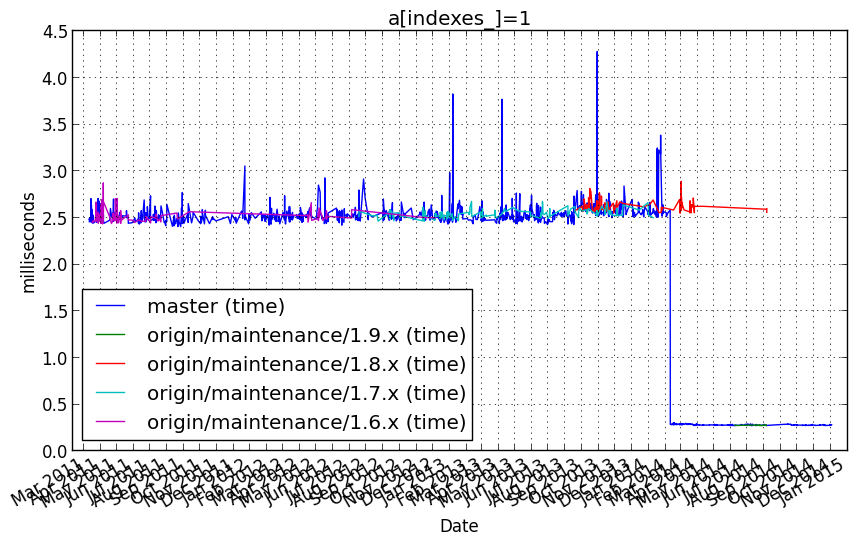
a[indexes_rand_]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[indexes_rand_]
Performance graph
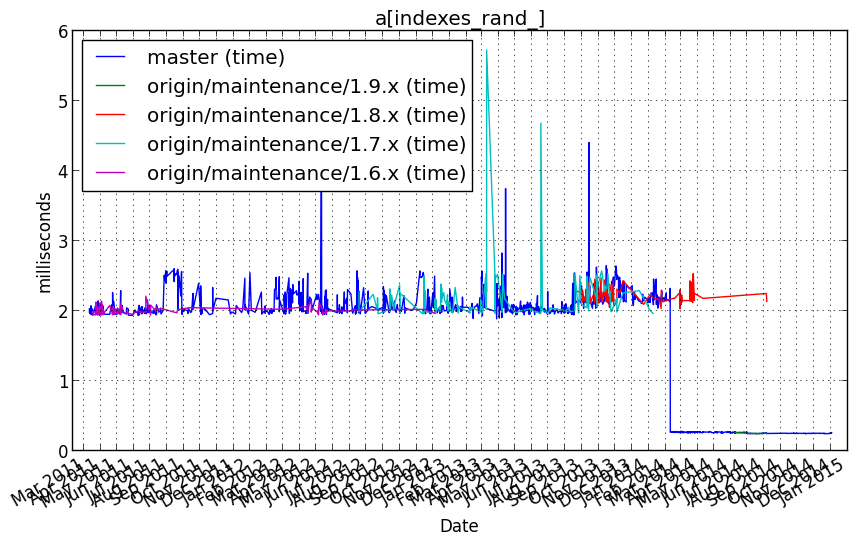
a[indexes_rand_]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[indexes_rand_]=1
Performance graph
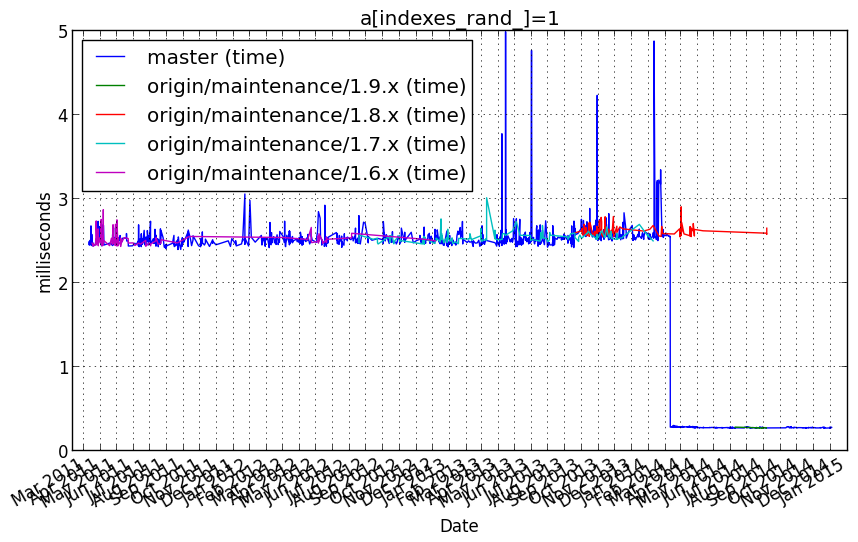
a[np.ix_(indexes_, indexes_)]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[np.ix_(indexes_, indexes_)]
Performance graph
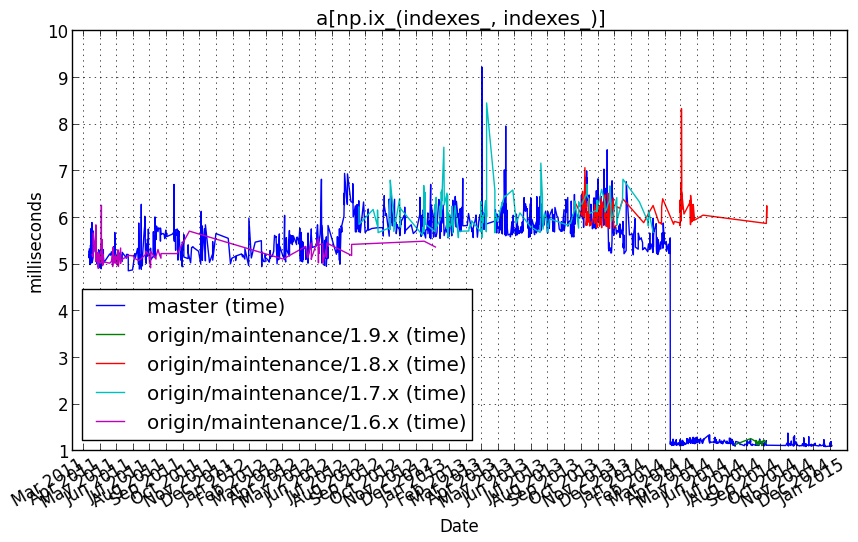
a[np.ix_(indexes_, indexes_)]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[np.ix_(indexes_, indexes_)]=1
Performance graph
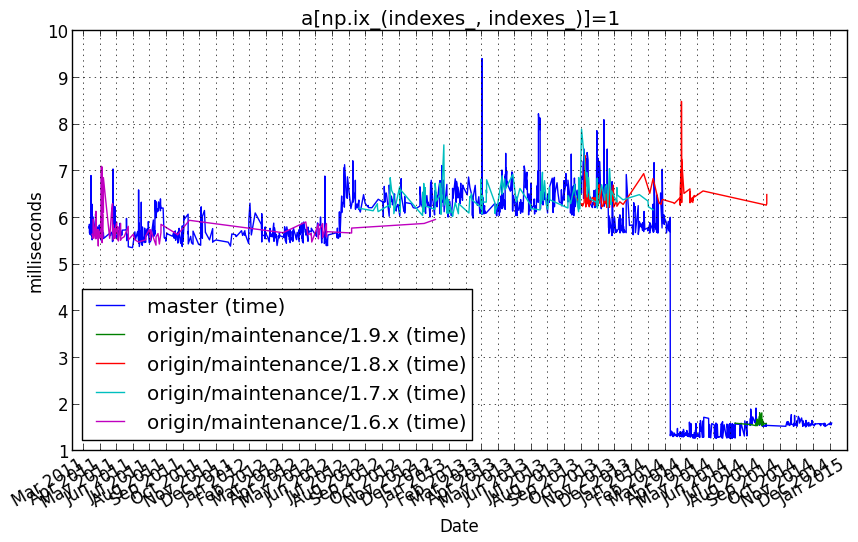
a[np.ix_(indexes_rand_, indexes_rand_)]¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[np.ix_(indexes_rand_, indexes_rand_)]
Performance graph
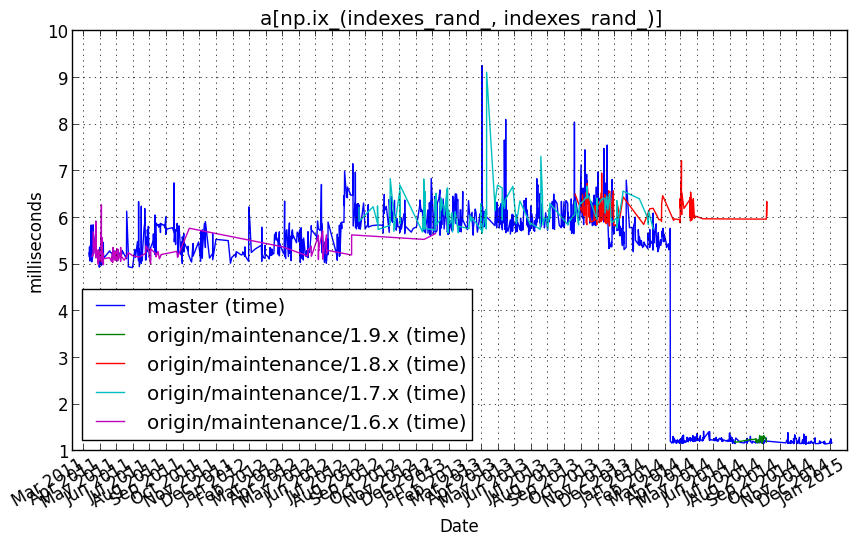
a[np.ix_(indexes_rand_, indexes_rand_)]=1¶
Benchmark setup
from numpy_vb_common import *
Benchmark statement
for a in squares_.itervalues(): a[np.ix_(indexes_rand_, indexes_rand_)]=1
Performance graph
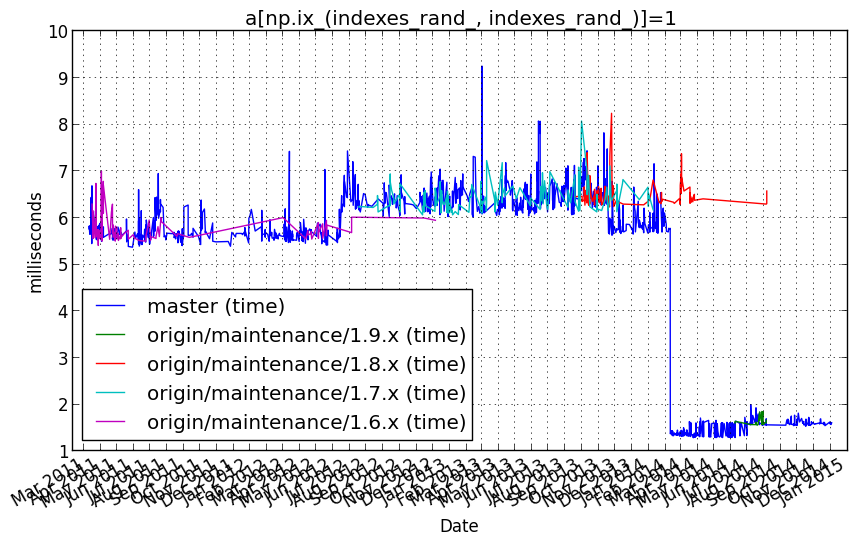
mmap_fancy_indexing¶
Benchmark setup
import tempfile
from numpy import memmap, float32, array
fp = memmap(tempfile.NamedTemporaryFile(), dtype=float32, mode='w+', shape=(50,60))
indexes = array([3,4,6,10,20])
Benchmark statement
for i in range(1000):
fp[indexes]
Performance graph
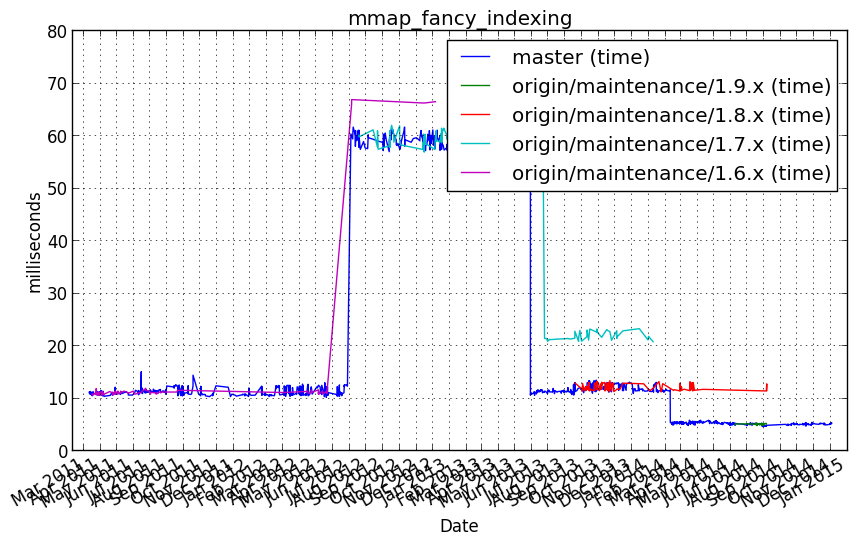
mmap_slicing¶
Benchmark setup
import tempfile
from numpy import memmap, float32, array
fp = memmap(tempfile.NamedTemporaryFile(), dtype=float32, mode='w+', shape=(50,60))
Benchmark statement
for i in range(1000):
fp[5:10]
Performance graph
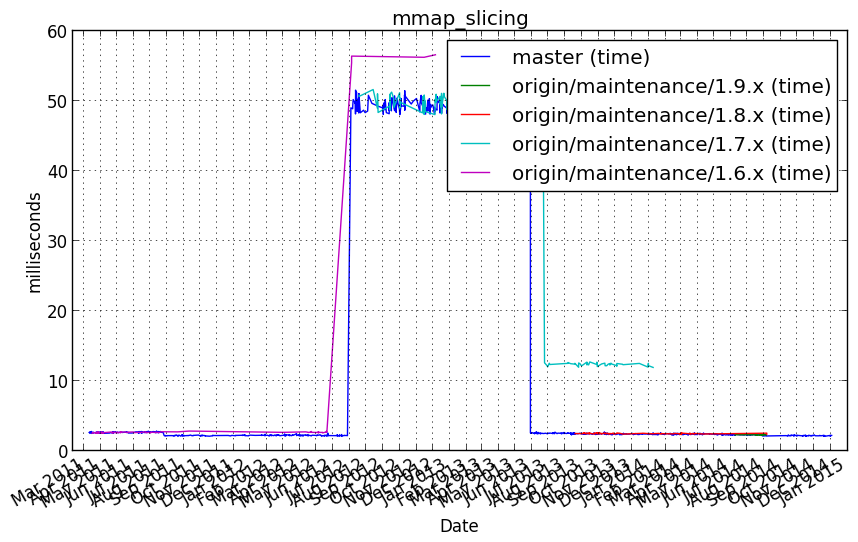