vb_io¶
cont_assign_complex128¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex128).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex128).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex128)
Benchmark statement
d[...] = 1
Performance graph
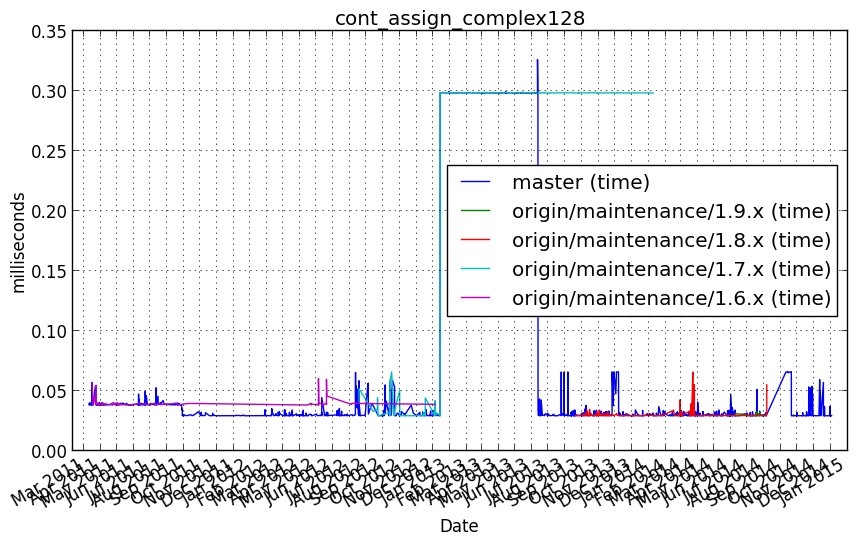
cont_assign_complex64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex64)
Benchmark statement
d[...] = 1
Performance graph
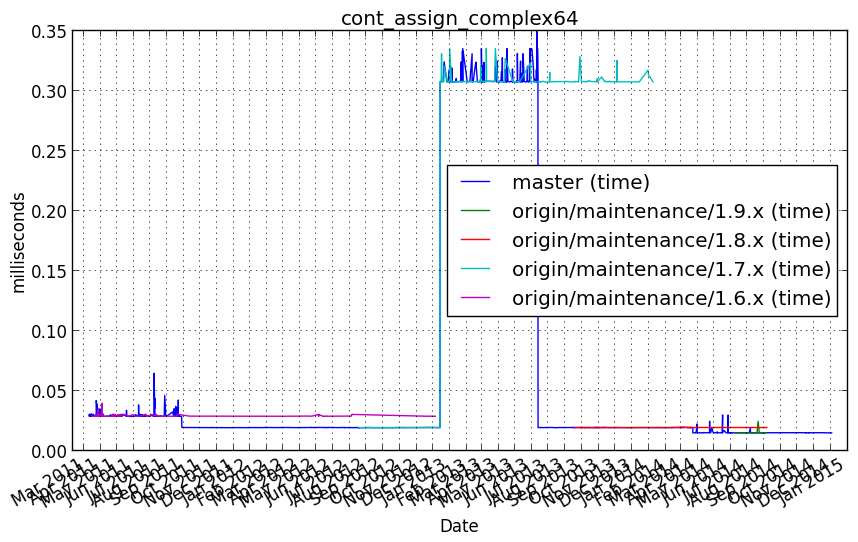
cont_assign_float32¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float32).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float32).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float32)
Benchmark statement
d[...] = 1
Performance graph
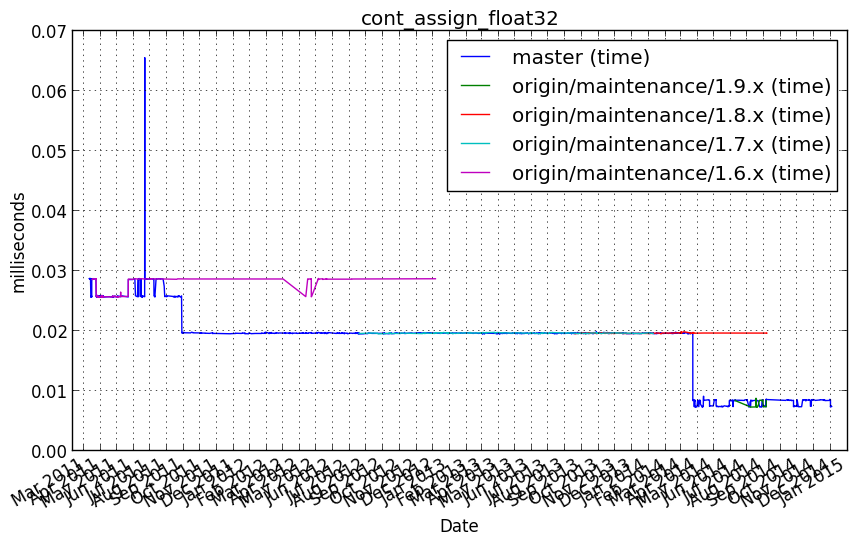
cont_assign_float64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float64)
Benchmark statement
d[...] = 1
Performance graph
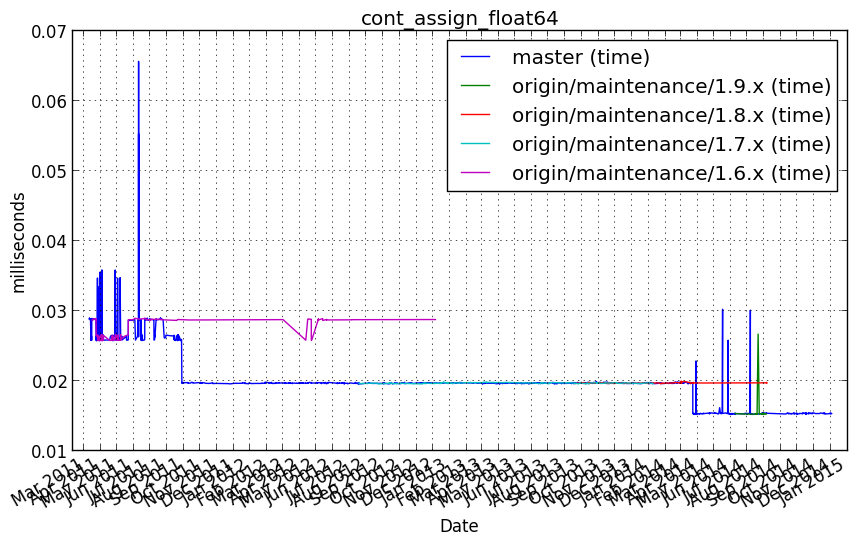
cont_assign_int16¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int16).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int16).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int16)
Benchmark statement
d[...] = 1
Performance graph
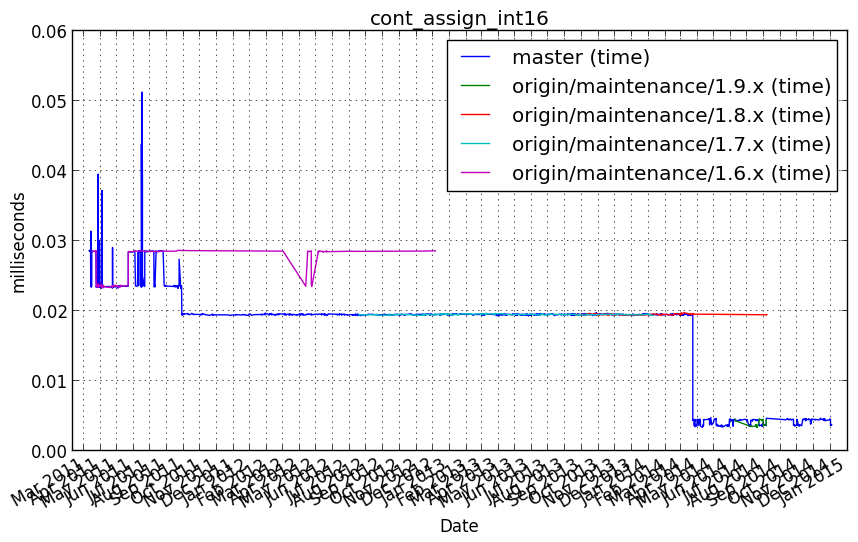
cont_assign_int8¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int8).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int8).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int8)
Benchmark statement
d[...] = 1
Performance graph
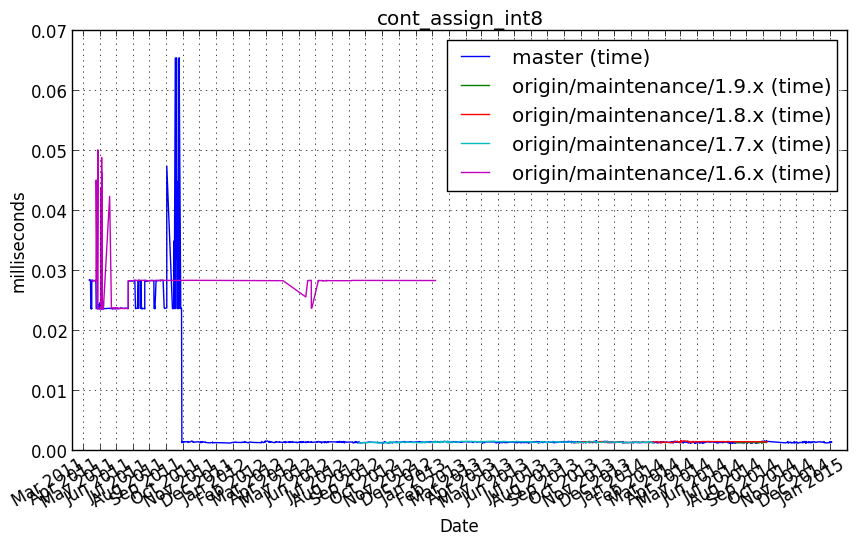
copyto¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.ones(50000)
e = d.copy()
m = d == 1
im = ~m
m8 = m.copy()
m8[::8] = ~(m[::8])
im8 = ~m8
Benchmark statement
np.copyto(d, e)
Performance graph
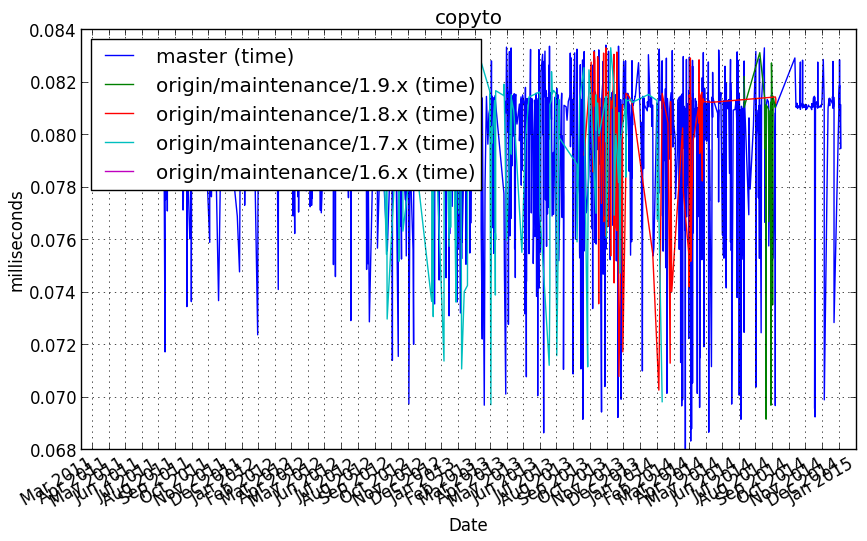
copyto_8_dense¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.ones(50000)
e = d.copy()
m = d == 1
im = ~m
m8 = m.copy()
m8[::8] = ~(m[::8])
im8 = ~m8
Benchmark statement
np.copyto(d, e, where=im8)
Performance graph
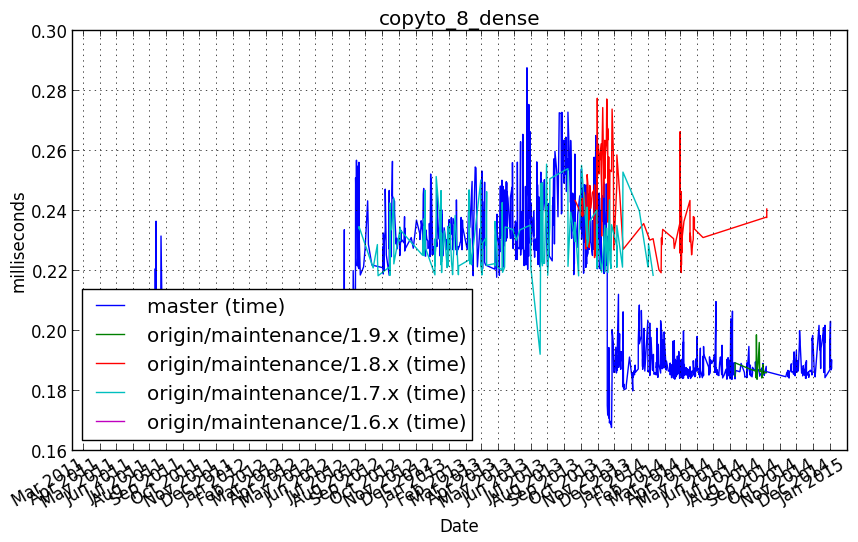
copyto_8_sparse¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.ones(50000)
e = d.copy()
m = d == 1
im = ~m
m8 = m.copy()
m8[::8] = ~(m[::8])
im8 = ~m8
Benchmark statement
np.copyto(d, e, where=m8)
Performance graph
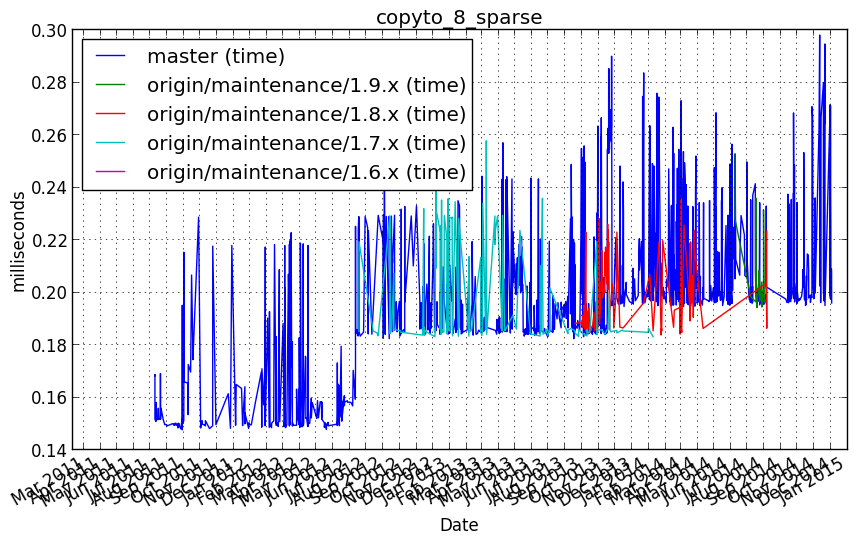
copyto_dense¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.ones(50000)
e = d.copy()
m = d == 1
im = ~m
m8 = m.copy()
m8[::8] = ~(m[::8])
im8 = ~m8
Benchmark statement
np.copyto(d, e, where=im)
Performance graph
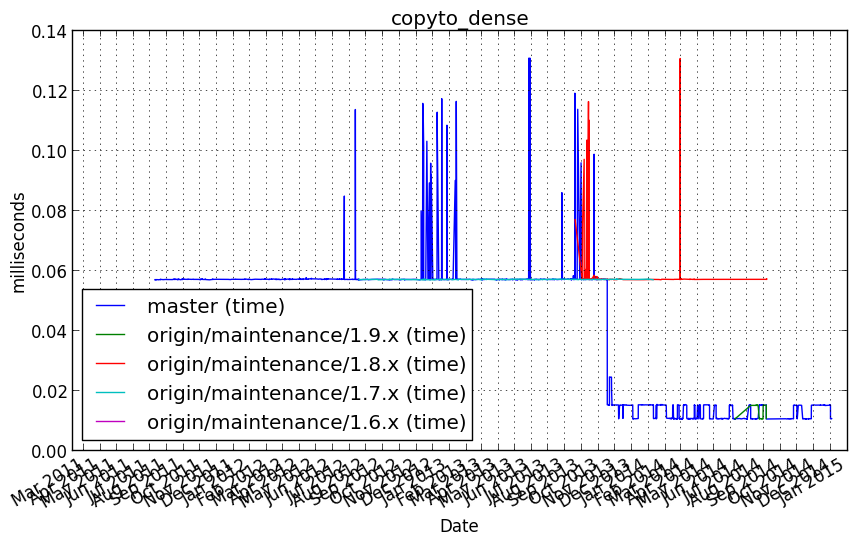
copyto_sparse¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.ones(50000)
e = d.copy()
m = d == 1
im = ~m
m8 = m.copy()
m8[::8] = ~(m[::8])
im8 = ~m8
Benchmark statement
np.copyto(d, e, where=m)
Performance graph
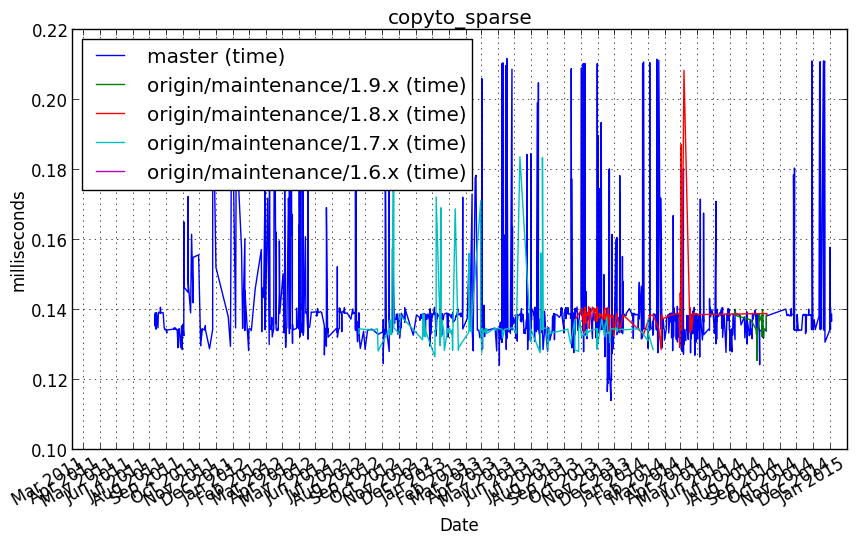
memcpy_complex128¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex128).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex128).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex128)
Benchmark statement
d[...] = e_d
Performance graph
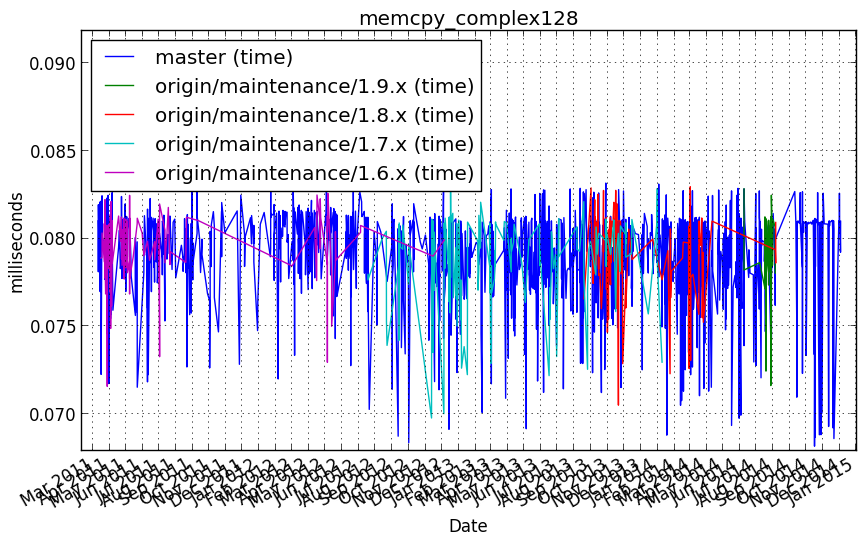
memcpy_complex64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex64)
Benchmark statement
d[...] = e_d
Performance graph
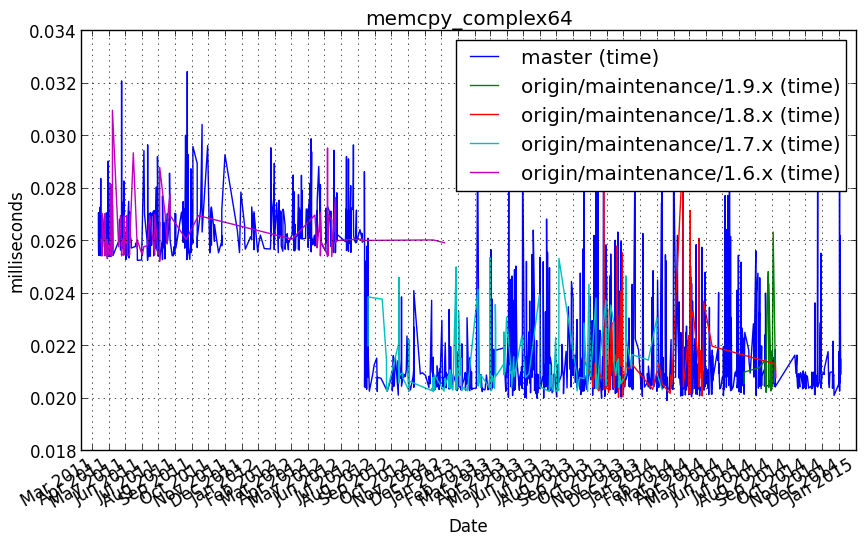
memcpy_float32¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float32).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float32).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float32)
Benchmark statement
d[...] = e_d
Performance graph
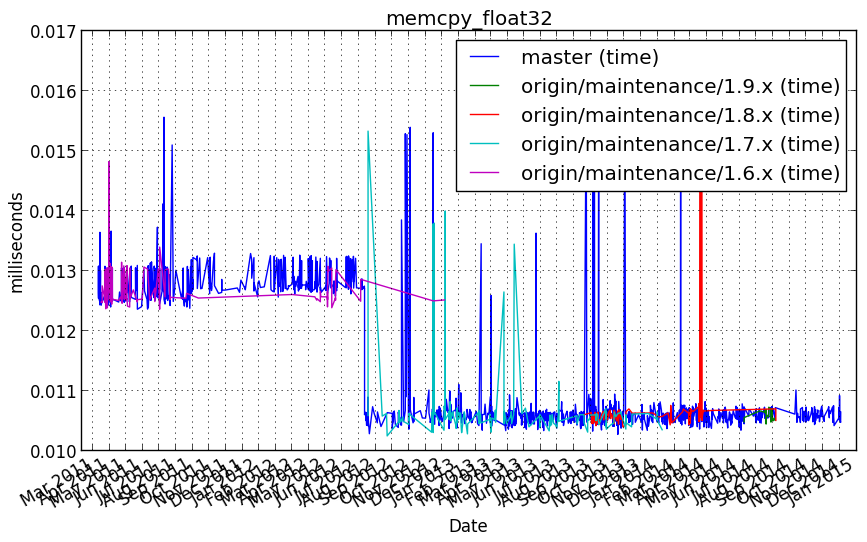
memcpy_float64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float64)
Benchmark statement
d[...] = e_d
Performance graph
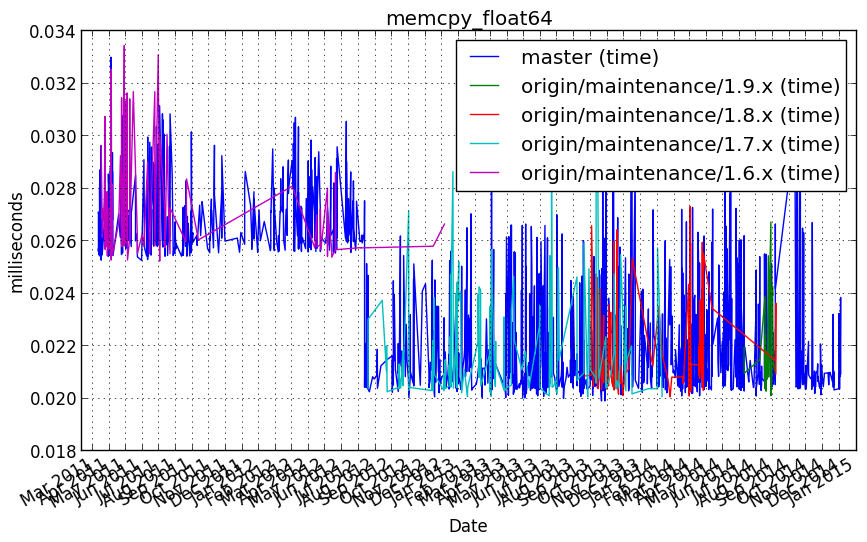
memcpy_int16¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int16).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int16).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int16)
Benchmark statement
d[...] = e_d
Performance graph
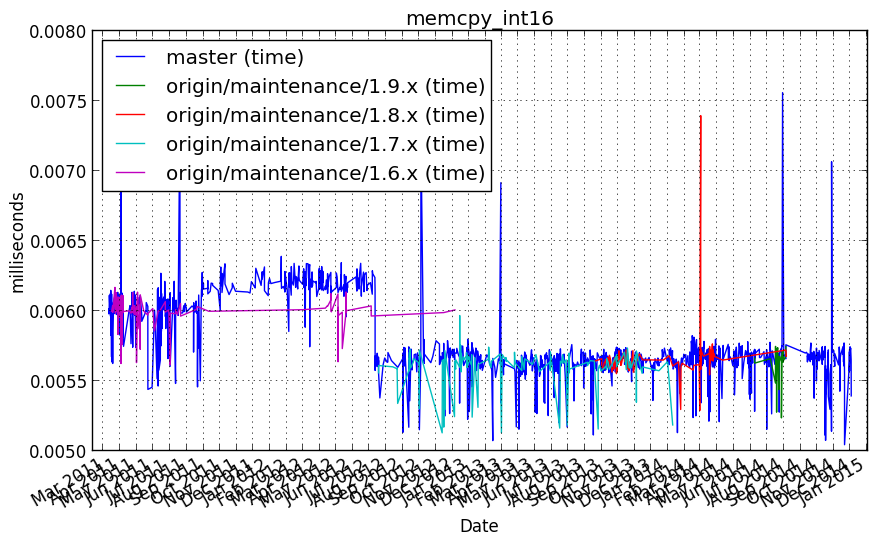
memcpy_int8¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int8).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int8).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int8)
Benchmark statement
d[...] = e_d
Performance graph
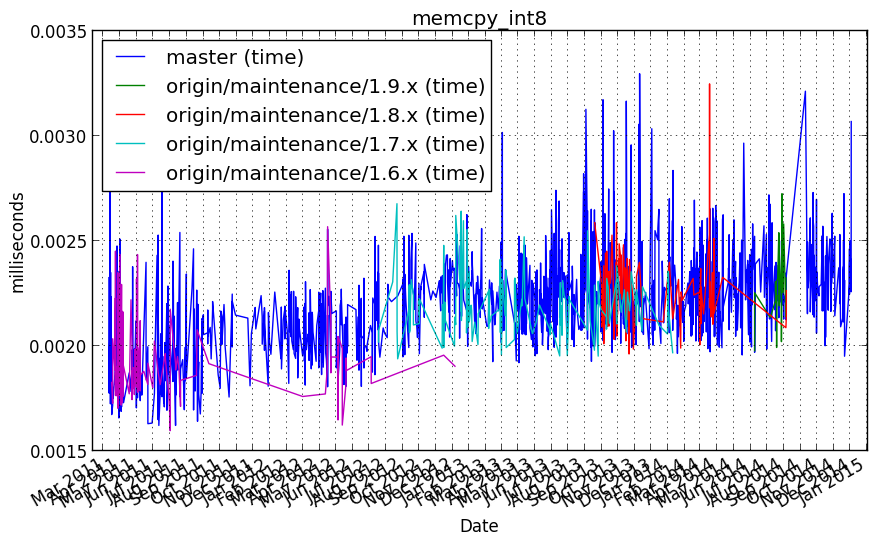
strided_assign_complex128¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex128).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex128).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex128)
Benchmark statement
dflat[::2] = 2
Performance graph
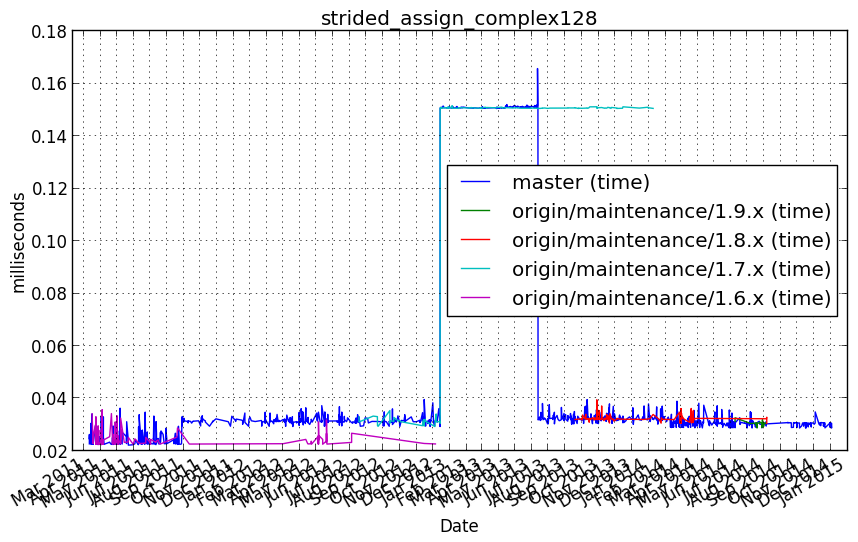
strided_assign_complex64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex64)
Benchmark statement
dflat[::2] = 2
Performance graph
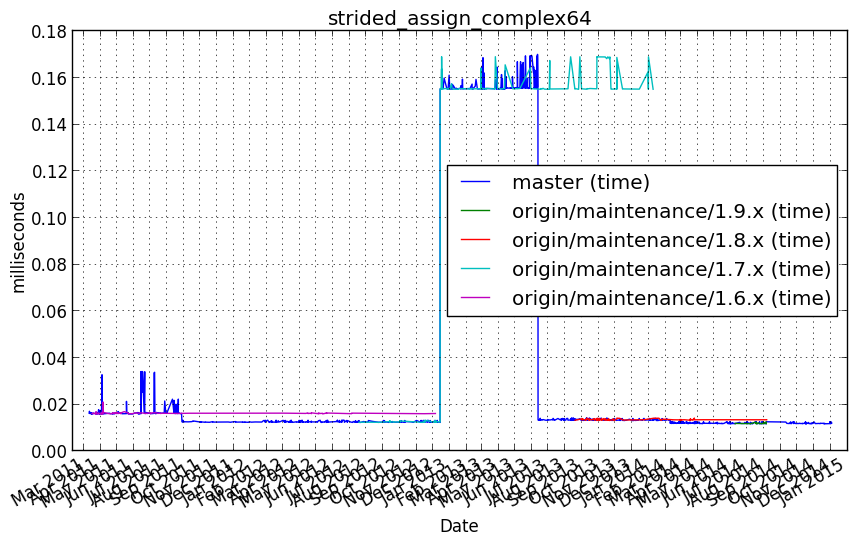
strided_assign_float32¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float32).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float32).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float32)
Benchmark statement
dflat[::2] = 2
Performance graph
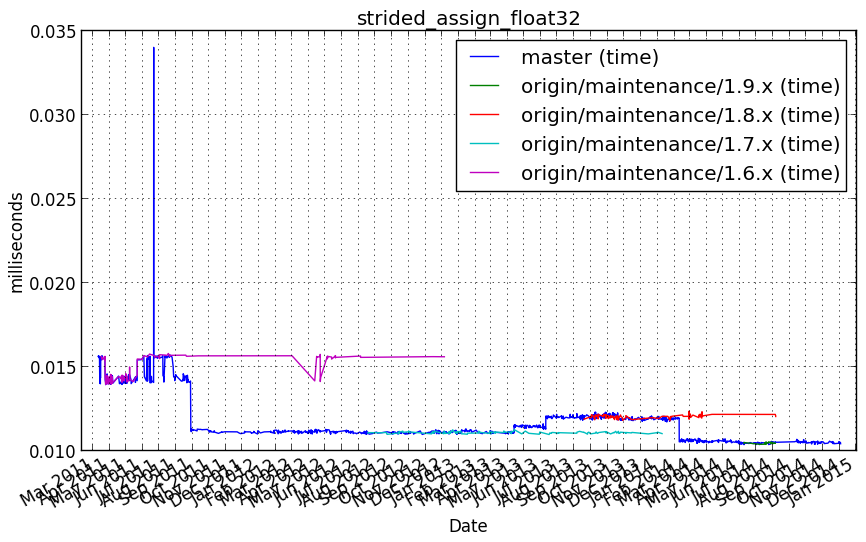
strided_assign_float64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float64)
Benchmark statement
dflat[::2] = 2
Performance graph
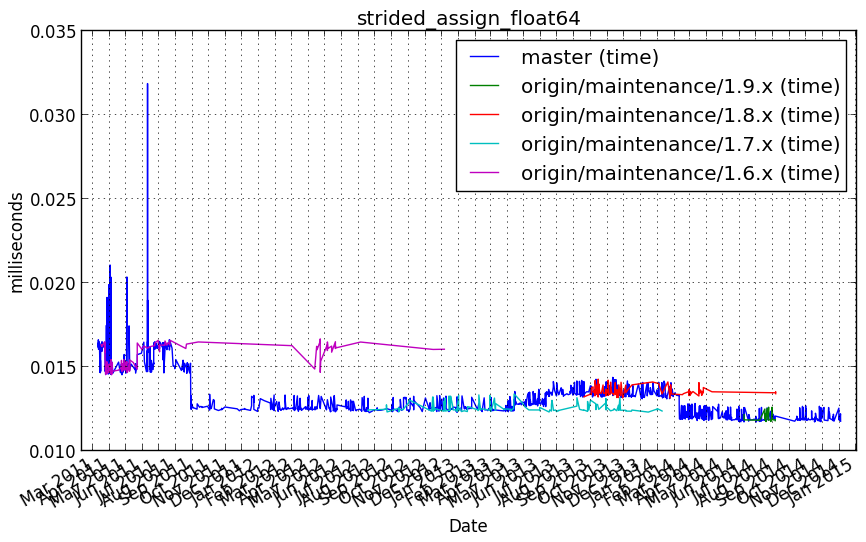
strided_assign_int16¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int16).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int16).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int16)
Benchmark statement
dflat[::2] = 2
Performance graph
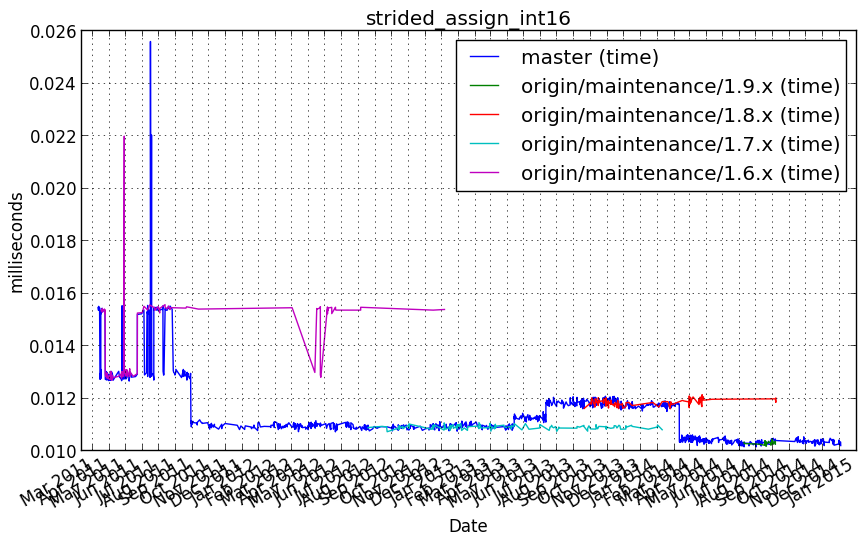
strided_assign_int8¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int8).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int8).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int8)
Benchmark statement
dflat[::2] = 2
Performance graph
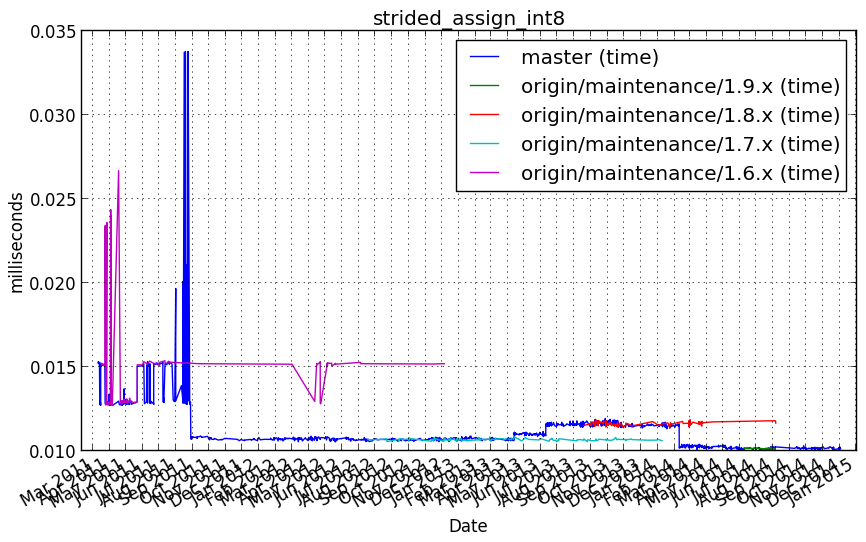
strided_copy_complex128¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex128).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex128).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex128)
Benchmark statement
d[...] = e.T
Performance graph
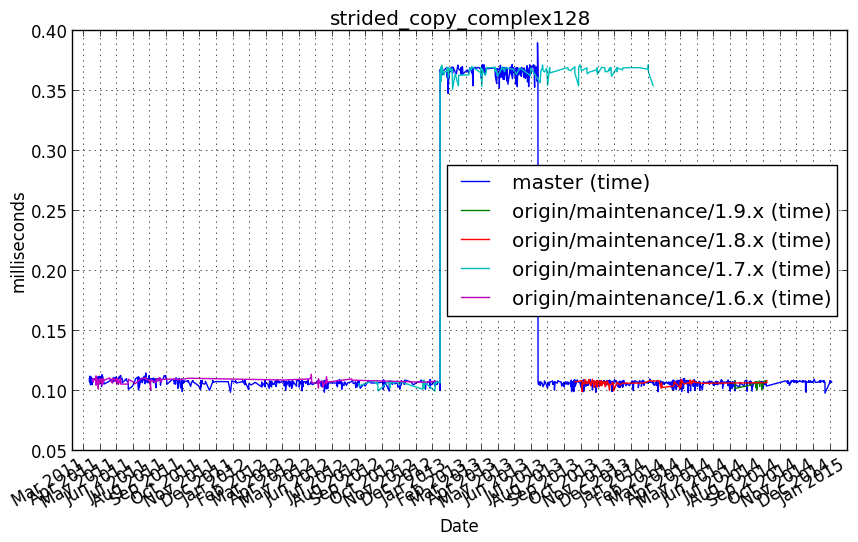
strided_copy_complex64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.complex64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.complex64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.complex64)
Benchmark statement
d[...] = e.T
Performance graph
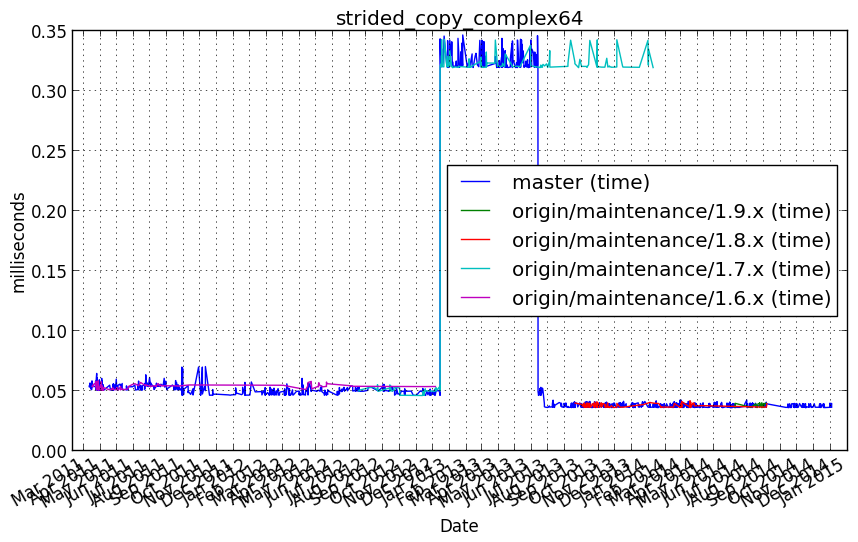
strided_copy_float32¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float32).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float32).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float32)
Benchmark statement
d[...] = e.T
Performance graph
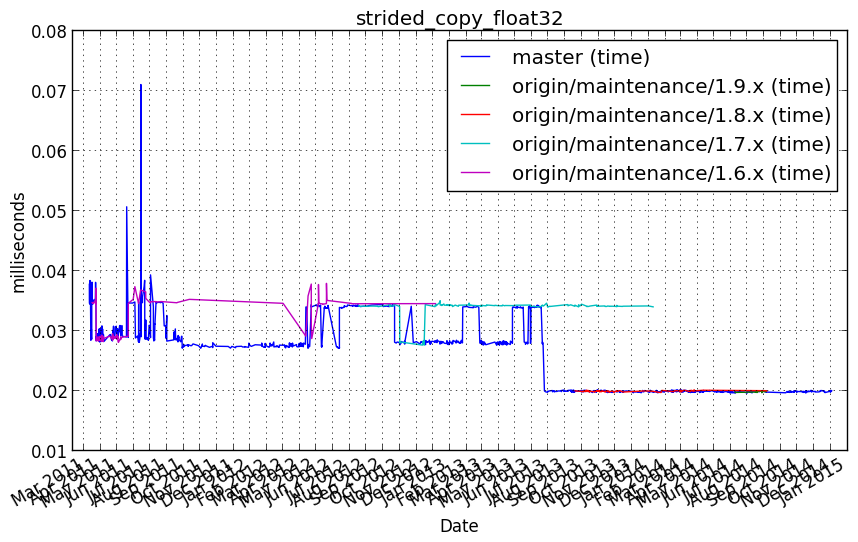
strided_copy_float64¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.float64).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.float64).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.float64)
Benchmark statement
d[...] = e.T
Performance graph
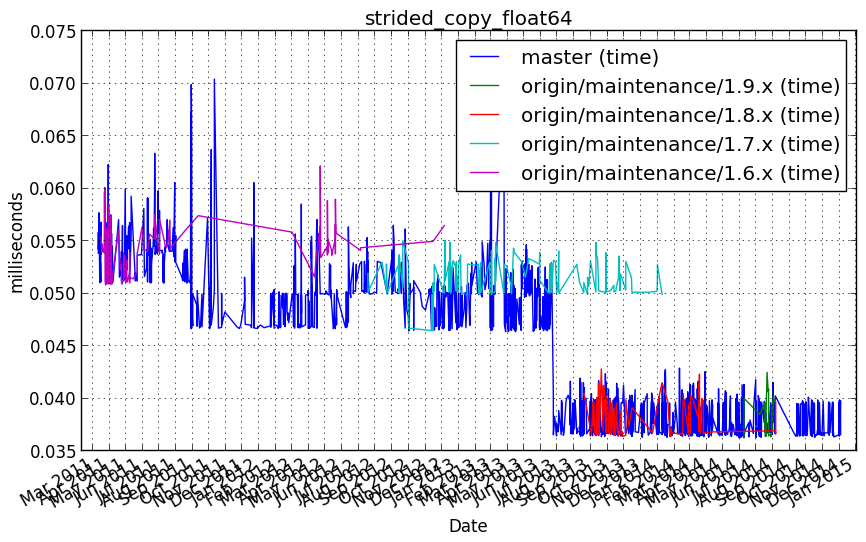
strided_copy_int16¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int16).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int16).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int16)
Benchmark statement
d[...] = e.T
Performance graph
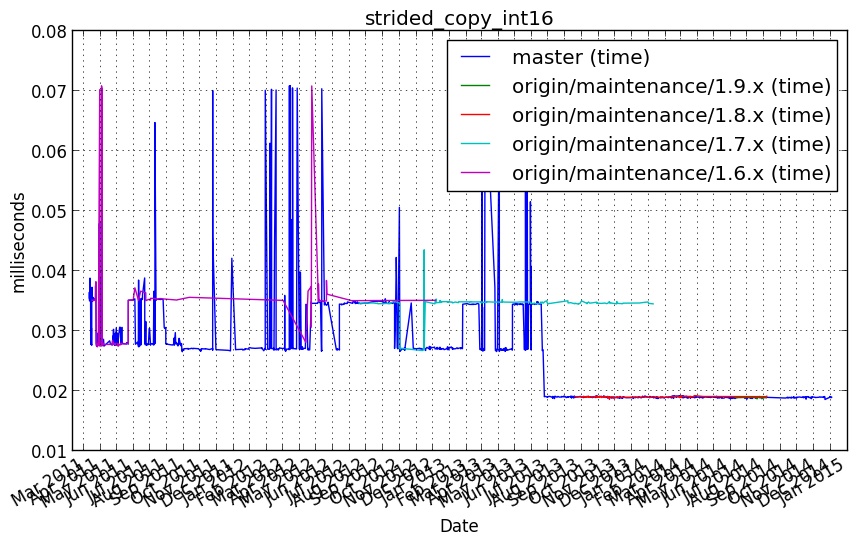
strided_copy_int8¶
Benchmark setup
import numpy
from tempfile import TemporaryFile
d = numpy.arange(50*500, dtype=numpy.int8).reshape((500,50))
e = numpy.arange(50*500, dtype=numpy.int8).reshape((50,500))
e_d = e.reshape(d.shape)
dflat = numpy.arange(50*500, dtype=numpy.int8)
Benchmark statement
d[...] = e.T
Performance graph
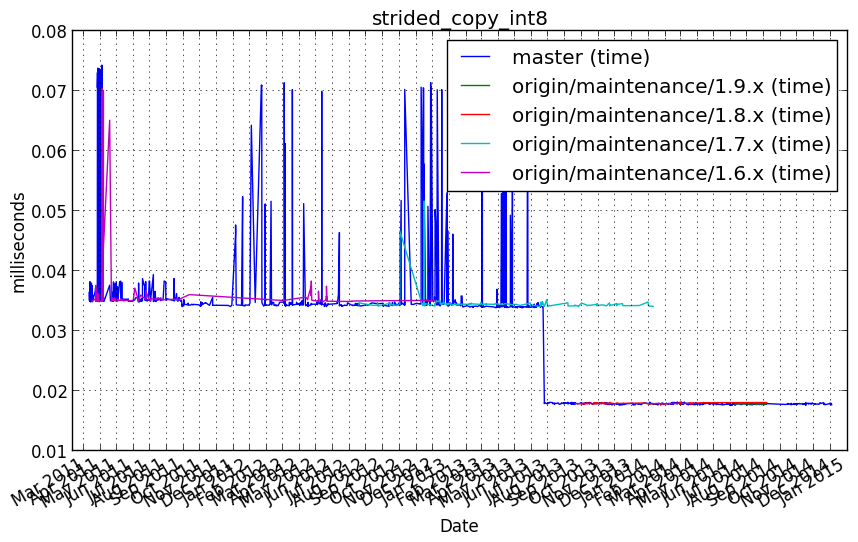
vb_savez_squares¶
Benchmark setup
from numpy_vb_common import squares;
import numpy
from tempfile import TemporaryFile
outfile = TemporaryFile()
Benchmark statement
numpy.savez(outfile, squares)
Performance graph
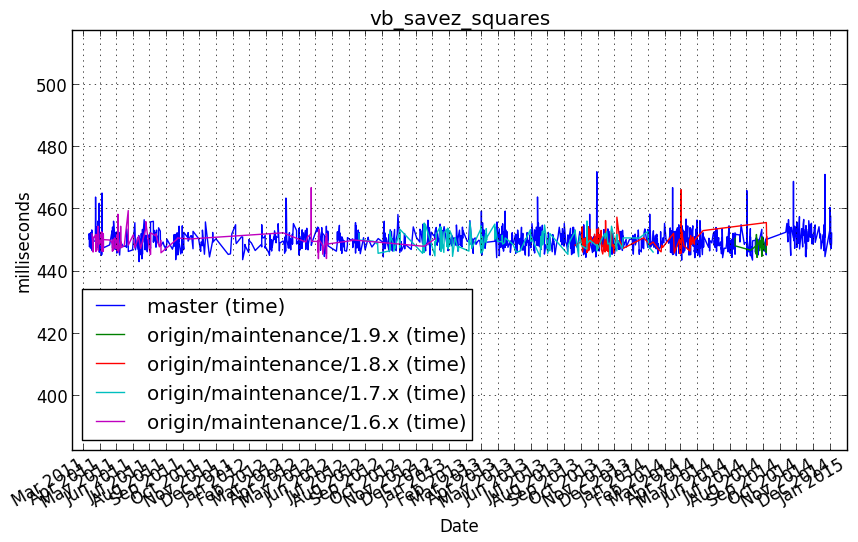